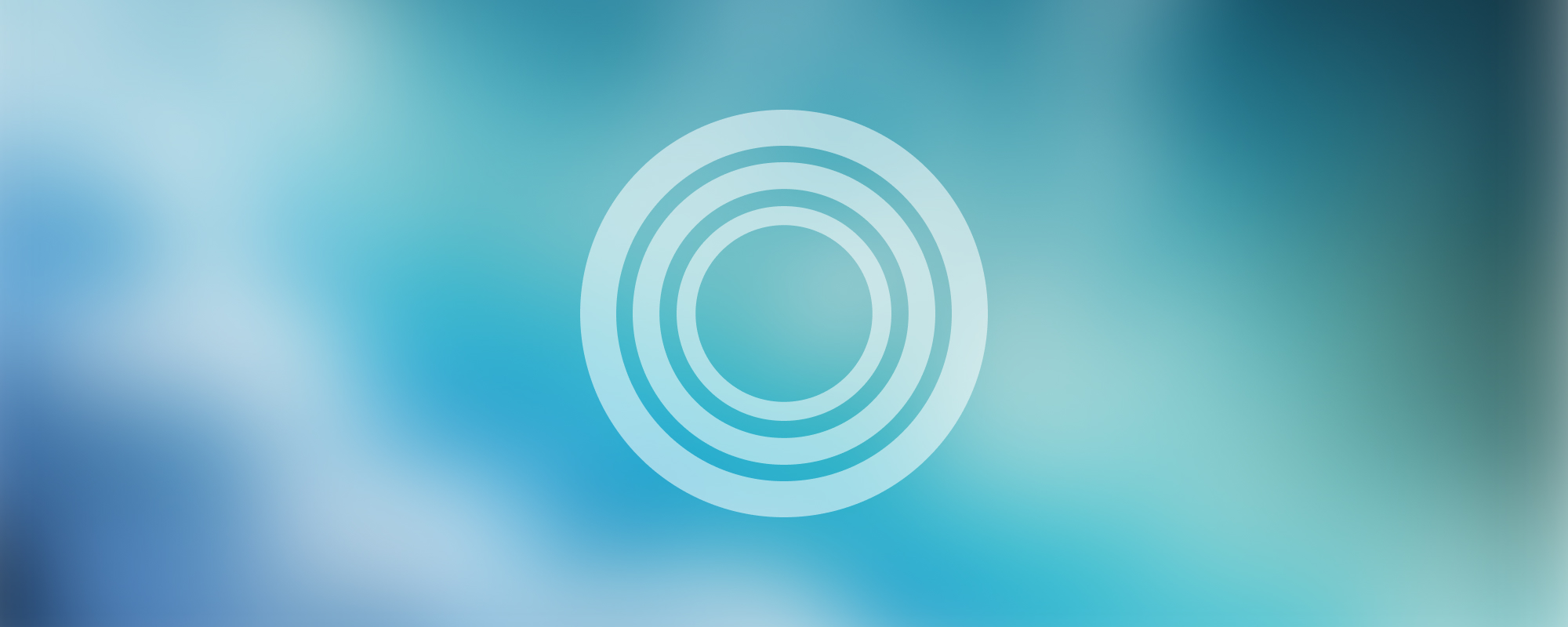
9. Visitor Pattern, SOLID Principles
Visitor Pattern and SOLID Principles in Object-Oriented Programming
Visitor Pattern
Introduction to Visitor Pattern
The Visitor Pattern is a behavioral design pattern that allows adding new behaviors to existing class hierarchy without altering any existing code. It is a way of separating an algorithm from an object structure on which it operates.
Benefits of Visitor Pattern
- It allows you to add or change operations on a class hierarchy without changing the classes themselves.
- You can execute an operation on a set of objects with different classes.
- Open/Closed Principle. You can introduce new behavior without changing existing code.
Example of Visitor Pattern
Here is a basic example in C#:
public interface IVisitor
{
void Visit(Element element);
}
public class ConcreteVisitor : IVisitor
{
public void Visit(Element element)
{
element.Operation();
}
}
public abstract class Element
{
public abstract void Accept(IVisitor visitor);
public abstract void Operation();
}
public class ConcreteElement : Element
{
public override void Accept(IVisitor visitor)
{
visitor.Visit(this);
}
public override void Operation()
{
// implementation
}
}
SOLID Principles
Introduction to SOLID Principles
SOLID is an acronym for a set of design principles that are widely used in Object-Oriented Programming (OOP) to make software design more understandable, flexible, and maintainable.
Single Responsibility Principle
The Single Responsibility Principle (SRP) states that a class should have only one reason to change. It means that a class should only have one job or responsibility.
Open/Closed Principle
The Open/Closed Principle (OCP) states that classes should be open for extension but closed for modification. It means that we should strive to write code that doesn't have to be changed every time the requirements change.
Liskov Substitution Principle
The Liskov Substitution Principle (LSP) states that subclasses must be substitutable for their base classes. In other words, a class can be replaced by any of its subclasses without affecting the correctness of the program.
Interface Segregation Principle
The Interface Segregation Principle (ISP) states that clients should not be forced to depend upon interfaces that they do not use. It means that a class should not be forced to implement interfaces it doesn't use.
Dependency Inversion Principle
The Dependency Inversion Principle (DIP) states that high-level modules should not depend on low-level modules. Both should depend on abstractions. In other words, dependencies on modules and classes should be based on abstractions not on concretions.
Clean Code Examples
Introduction to Clean Code
Clean code refers to writing code that is easily understandable, easy to maintain, and robust. The aim is to make the code more readable and understandable for any developer who works on it.
Example of Clean Code
- A method should not contain more than 100 lines of code. If it does, it should be split into smaller methods.
// Bad
public void DoSomethingComplex()
{
// 100+ lines of code
}
// Good
public void DoSomethingComplex()
{
DoFirstThing();
DoSecondThing();
DoThirdThing();
}
private void DoFirstThing() { /* ... */ }
private void DoSecondThing() { /* ... */ }
private void DoThirdThing() { /* ... */ }
- A class should not contain more than 1000 lines of code. If it does, it should be split into smaller classes.
// Bad
public class SuperComplexClass
{
// 1000+ lines of code
}
// Good
public class FirstSimpleClass { /* ... */ }
public class SecondSimpleClass { /* ... */ }
public class ThirdSimpleClass { /* ... */ }
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version