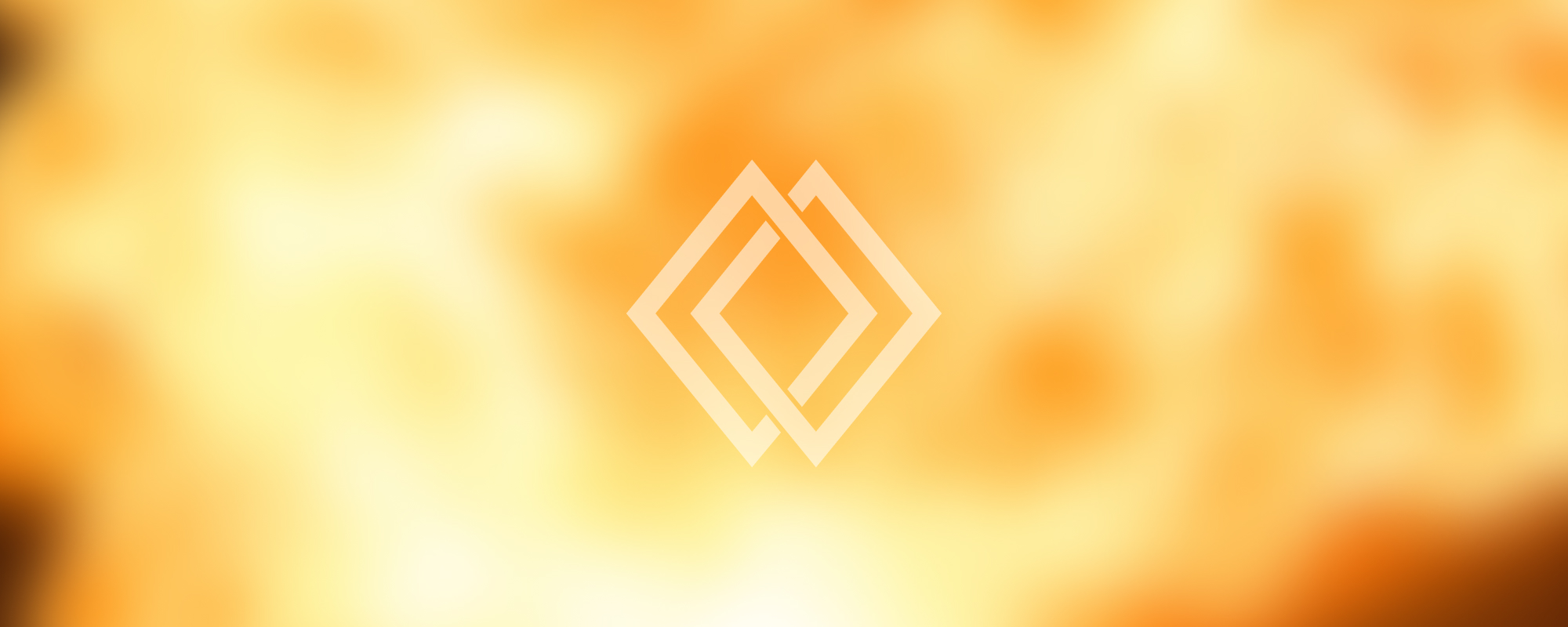
1. Introduction to Java Script
Introduction to JavaScript
Overview of JavaScript
JavaScript is a high-level, dynamic, interpreted programming language that is mainly used to create interactive web pages and web applications. It was initially created in 1995 by Brendan Eich while he was working at Netscape Communications Corporation with an aim to add dynamic and interactive elements to HTML pages, thus enabling developers to design more interactive and responsive websites.
JavaScript is one of the most popular programming languages globally and is supported by all major web browsers. It is a versatile and powerful tool for web development and server-side programming, thanks to the popular Node.js runtime environment.
How to Include JavaScript Code
JavaScript code can be embedded directly into HTML pages using the <script>
tag. It can also be included in separate .js files and then referenced by the HTML page.
<script src="index.js"></script>
Note: It is common practice to place the script tag at the end of the HTML body to prevent blocking the rendering of the webpage if a JavaScript error occurs.
Variables in JavaScript
In JavaScript, we can define variables using var
, let
, and const
.
var
: This keyword is used to declare a variable globally or locally to an entire function regardless of block scope.
let
: It allows you to declare variables that are limited to the scope of a block statement.
const
: It allows you to declare variables whose value are read-only after assignment.
var name = "John";
let age = 20;
const pi = 3.14;
Displaying Output in JavaScript
There are several ways to display output in JavaScript:
alert()
: Displays an alert box with a specified message.
console.log()
: Outputs a message to the web console.
document.write()
: Writes HTML expressions or JavaScript code to a document.
innerHTML
: Used to get or replace the content of HTML elements.
alert("Hello, World!");
console.log("Hello, World!");
document.write("Hello, World!");
document.getElementById("myTitle").innerHTML = "Hello, World!";
JavaScript Operators
===
: This is a strict equality operator. It returns true if the operands are equal and of the same type.
==
: This is a loose equality operator. It returns true if the operands are equal.
console.log(1 === 1); // Outputs: true
console.log('1' == 1); // Outputs: true
JavaScript Input Methods
prompt()
: Displays a dialog box that prompts the user for input.
confirm()
: Displays a dialog box with a specified message, along with an OK and a Cancel button.
let age = prompt("Please enter your age", "0");
let isConfirmed = confirm("Are you sure?");
JavaScript Eval Function
The eval()
function evaluates or executes an argument if it's a JavaScript expression.
console.log(eval('10 + 20')); // Outputs: 30
JavaScript is a functional language and also supports OOP (Object Oriented Programming). It is primarily used to make the browser interactive, manipulate HTML elements, handle user interactions, make HTTP requests to web servers, and much more. It is an essential tool for modern web development.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version