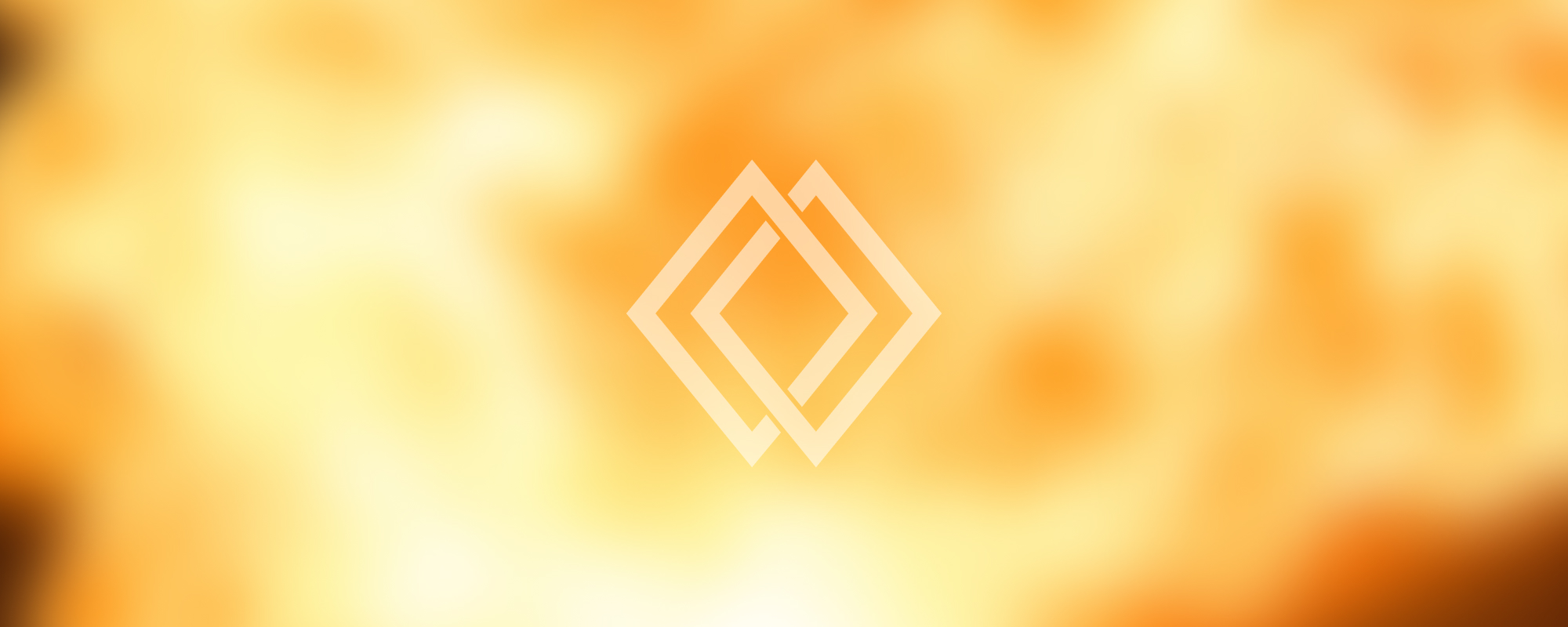
10. JQuery Project Description
JQuery Project Description
Introduction to JQuery
JQuery is a fast, small, and feature-rich JavaScript library. It makes things like HTML document traversal and manipulation, event handling, and animation much simpler with an easy-to-use API that works across a multitude of browsers.
Key Points:
- JQuery is a lightweight JavaScript library.
- It simplifies HTML document traversing, event handling, AJAX interactions and animation for rapid web development.
- It provides a simple way to pick up DOM elements.
Basic Syntax of jQuery
The basic syntax of jQuery is $(selector).action()
$
sign to define/access jQuery
(selector)
to query HTML elements
action()
to be performed on the element(s)
Example:
$(document).ready(function(){
// jQuery methods go here...
});
The above code means to execute the jQuery function when the document is ready to be manipulated.
Creating a JQuery Project
Creating a jQuery project involves various steps which include:
1. Setting up the environment
This step involves linking the jQuery library to your HTML document. You can either download the jQuery library from jQuery.com or include it from a Content Delivery Network (CDN).
Example:
<head>
<script src="<https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js>"></script>
</head>
2. Understanding jQuery Selectors
jQuery selectors allow you to select and manipulate HTML element(s). They are used to select HTML elements based on their id, classes, types, attributes, values of attributes, etc.
Example:
$('p').click(function(){
// action to be performed
});
The above code selects all paragraph elements and adds a click event to them.
3. Manipulating DOM Elements
JQuery provides a powerful set of tools for manipulating the Document Object Model (DOM).
Example:
$("button").click(function(){
$("p").hide();
});
The above example hides all paragraphs when the button is clicked.
4. Handling Events
JQuery also provides a robust and efficient way of handling user-triggered events such as clicking, hovering, keyboard input, etc.
Example:
$("p").dblclick(function(){
$(this).hide();
});
The above example hides the paragraph on double click.
5. Using AJAX for asynchronous web requests
With jQuery, AJAX, (Asynchronous JavaScript and XML), can be used to perform a variety of tasks such as updating a web page without reloading the page, request data from a server after the page has loaded, send data to a server in the background etc.
Example:
$("button").click(function(){
$.ajax({url: "demo_test.txt", success: function(result){
$("#div1").html(result);
}});
});
The above example fetches data from a file named "demo_test.txt" on the server and places the returned data into the element with id="div1".
Conclusion
Building a project with jQuery involves several other advanced topics and methods. However, the above-given steps and examples provide a basic understanding of how to get started with jQuery.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version