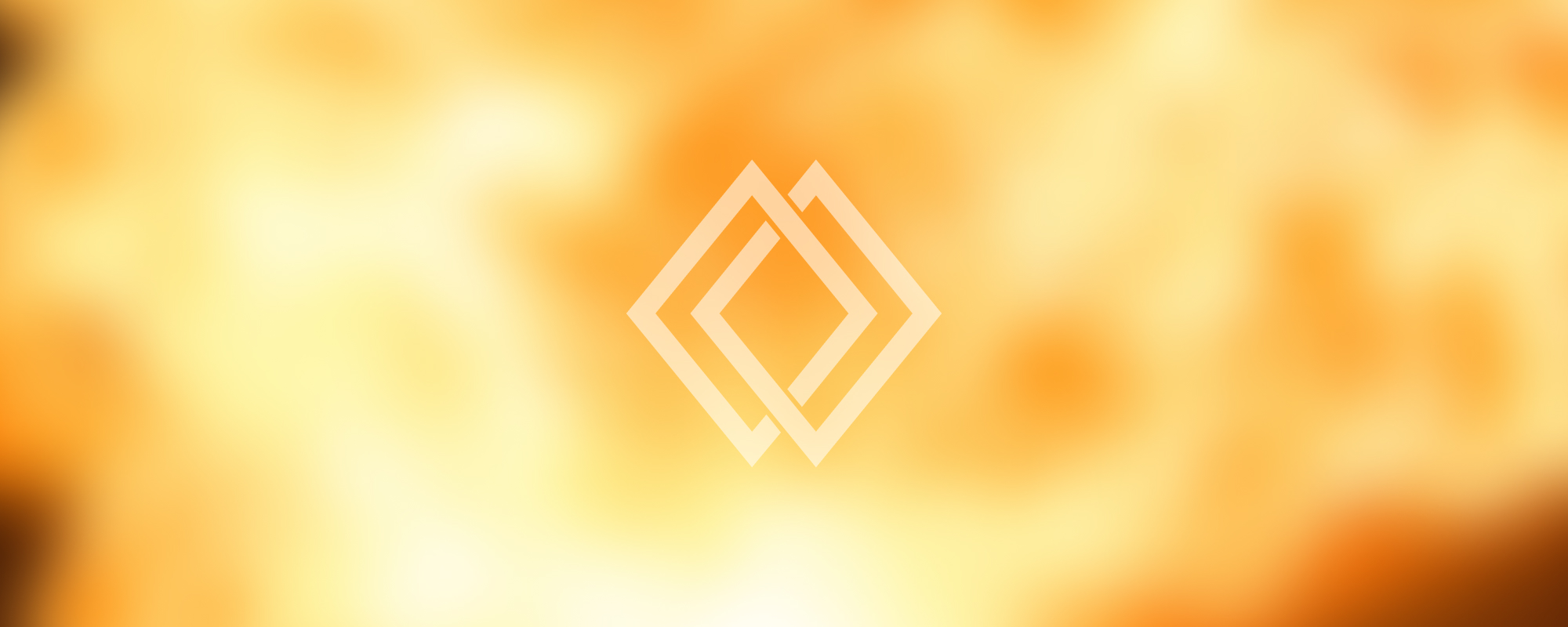
11. JQuery Practise
JQuery Practise
Introduction to JQuery
JQuery is a fast, small, and feature-rich JavaScript library that simplifies HTML document traversal and manipulation, event handling, animation, and AJAX. It is designed to change the way you write JavaScript.
Key Points:
- JQuery is a library that makes it easier to use JavaScript on your website.
- It takes a lot of common tasks that require many lines of JavaScript code to accomplish and wraps them into methods that you can call with a single line of code.
- JQuery also simplifies complicated things from JavaScript, like AJAX calls and DOM manipulation.
Basic Syntax
The basic syntax of JQuery is $(selector).action()
- A dollar sign to define/access jQuery
- A (selector) to "query (or find)" HTML elements
- A jQuery action() to be performed on the element(s)
Examples:
$(document).ready(function(){
// jQuery methods go here...
});
In the example above, $(document).ready()
is a function to prevent any jQuery code from running before the document is finished loading.
Selectors
JQuery selectors allow you to select and manipulate HTML element(s). They are used to "find" (or select) HTML elements based on their name, id, classes, types, attributes, values of attributes, etc.
Example:
$("p").click(function(){
// action goes here...
});
In the example above, $("p")
is a selector that selects all <p>
elements.
Events
Events are the visitors' actions that a web page can respond to, such as moving a mouse over an element, selecting a radio button, clicking on an element, etc. JQuery provides simple methods for handling events.
Example:
$("p").click(function(){
// action goes here...
});
In the example above, the click
method defines what will happen when a click event fires.
Effects and Animations
JQuery comes with a bunch of built-in animation effects which you can use in your websites. Some basic effects are: hide()
, show()
, toggle()
, fadeOut()
, fadeIn()
, slideUp()
, slideDown()
, animate()
, etc.
Example:
$("button").click(function(){
$("p").hide();
});
In the example above, hide
method is used to hide all <p>
elements when a button is clicked.
AJAX
AJAX is the art of exchanging data with a server, and updating parts of a web page without reloading the whole page. The load()
, $.get()
, $.post()
, $.ajax()
methods are some of the AJAX methods provided by JQuery.
Example:
$("button").click(function(){
$.get("demo_test.asp", function(data, status){
alert("Data: " + data + "\\nStatus: " + status);
});
});
In the example above, $.get()
method is used to request data from a server.
By practising JQuery, you can create dynamic, feature-rich, and responsive websites with less code and effort.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version