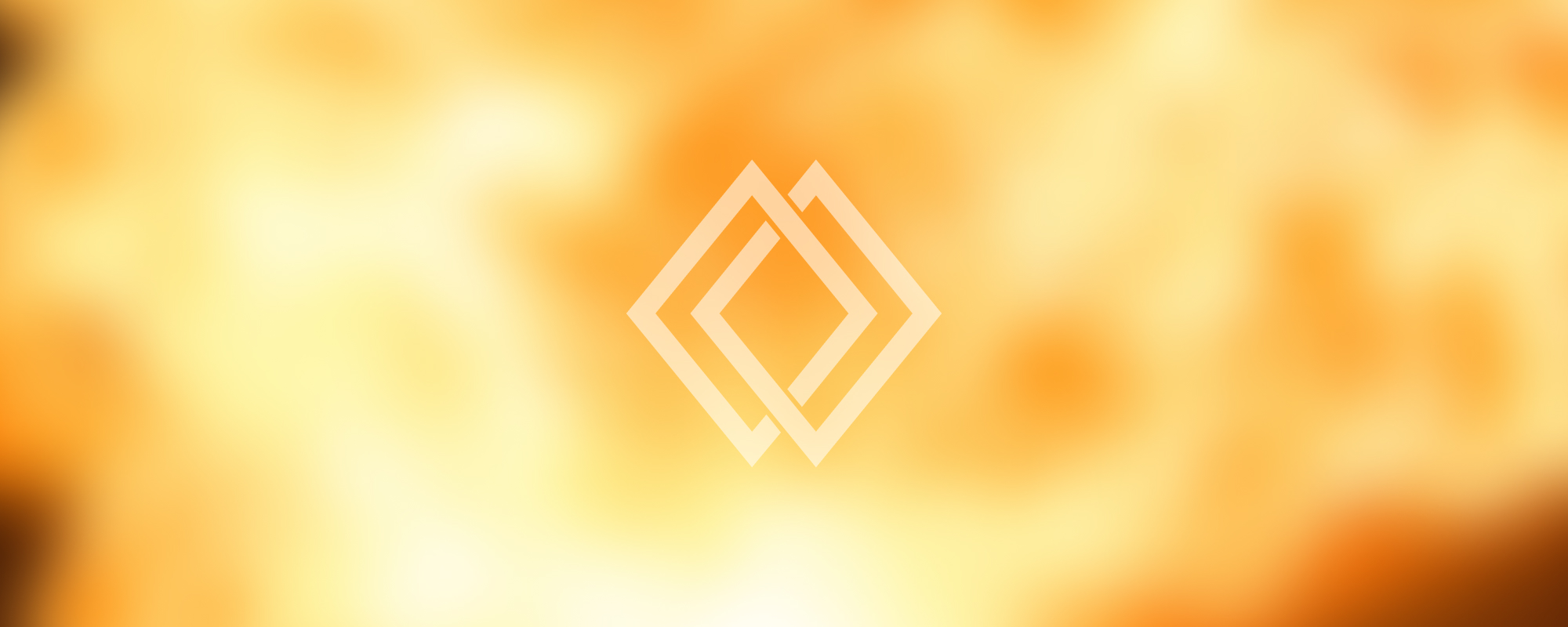
12. AJAX
AJAX in Web Development
Introduction to AJAX
AJAX stands for "Asynchronous JavaScript and XML". It is a powerful set of web development techniques employed to create fast, dynamic, and interactive web pages. A significant advantage of AJAX is that it allows updating parts of a web page without reloading the entire page. This enhances the website's performance and leads to a smoother and more responsive user experience.
Key Features of AJAX:
- AJAX allows for asynchronous requests, meaning the user can continue to use the website while the server processes the request.
- Supports multiple data formats such as XML, JSON, or HTML.
- It is supported by all major web browsers and is an essential part of modern web development.
How AJAX Works
The fundamental concept behind AJAX involves using JavaScript to make asynchronous requests to the server. The server responds with data in various formats (XML, JSON, or HTML), which can then be processed and displayed on the web page without requiring a full page reload.
Common Uses of AJAX
AJAX is commonly used in web applications to provide features such as:
- Auto-suggest search boxes: As the user types, suggestions appear based on their input.
- Live chat: Allows real-time communication between users or between a user and an AI.
- Dynamic forms: Forms that change based on user input or other actions.
AJAX with jQuery
jQuery simplifies the process of working with AJAX by providing easy-to-use methods. Some common jQuery functions that interact with AJAX are:
jQuery Methods
find()
This method is used to find an element inside another element.
$("div").find("p"); // Finds all <p> elements inside <div> elements
children()
This method is used to find an element in element’s children elements.
$("div").children(".intro");//Finds all children with class="intro" of all <div> elements
siblings()
This method is used to get all sibling elements of the selected element.
$("h2").siblings(); // Gets all sibling elements of <h2>
first()
This method is used to get the first element of the selected elements.
$("div").first(); // Selects the first <div> element
hide()
This method is used to hide the selected elements.
$("p").hide(); // Hides all <p> elements
eq()
This method is used to select an element with a specific index number.
$("p").eq(2); // Selects the third <p> element (index starts at 0)
filter()
This method is used to filter out elements that don't match certain criteria.
$("p").filter(".intro"); // Selects all <p> elements with class="intro"
not()
This method is used to select elements that do not match certain criteria.
$("p").not(".intro"); // Selects all <p> elements that do not have class="intro"
prevUntil()
This method returns all preceding siblings of an element up to but not including the element matched by the parameter.
$("h2").prevUntil("h6");// Selects all sibling elements between a <h2> and a <h6> element
nextAll()
This method returns all following siblings of an element.
$("h2").nextAll(); // Selects all sibling elements that follow a <h2>
load()
This method loads data from a server and puts the returned data into the selected element.
$("#div1").load("demo_test.txt"); // Loads the content of "demo_test.txt" into a specific <div>
$.get()
This method requests data from the server with a HTTP GET request.
$.get("demo_test.asp", function(data, status){
alert("Data: " + data + "\\nStatus: " + status);
});
$.post()
This method sends data to the server with a HTTP POST request.
$.post("demo_test_post.asp",
{
name: "Donald Duck",
city: "Duckburg"
},
function(data, status){
alert("Data: " + data + "\\nStatus: " + status);
});
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version