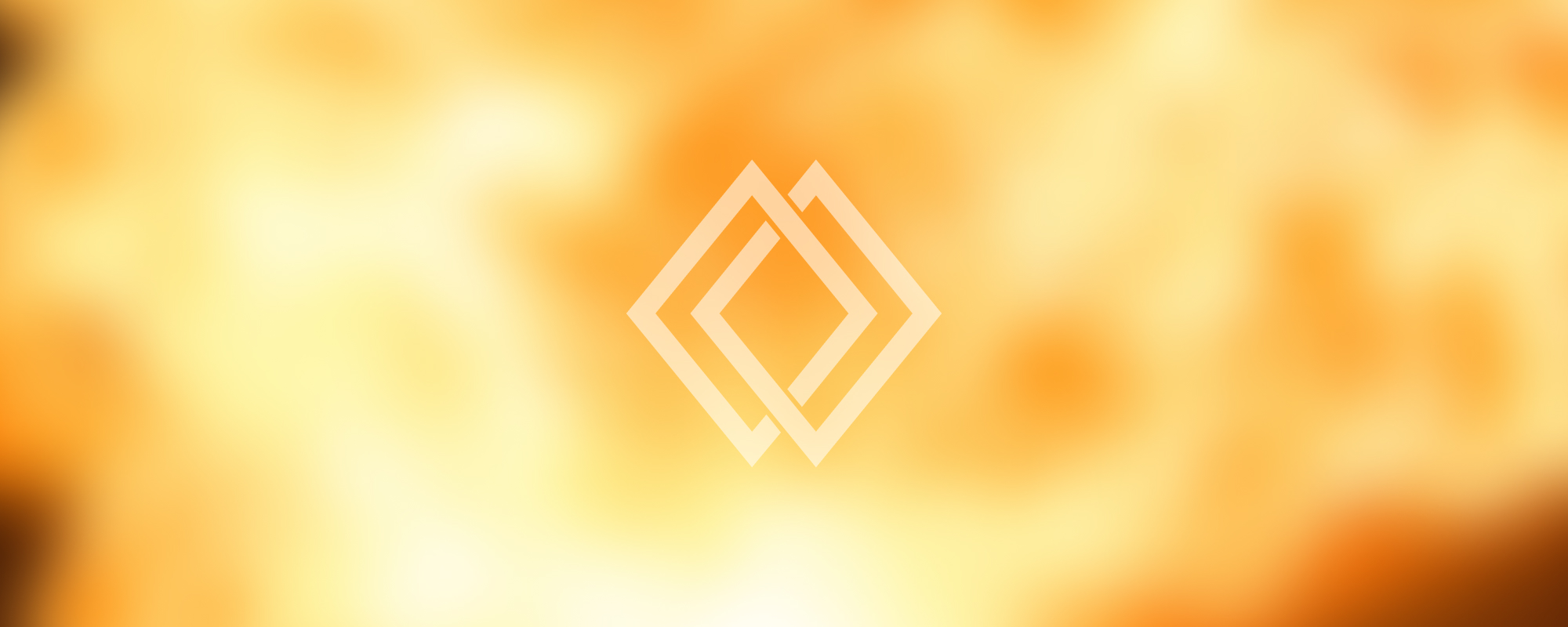
2. JavaScript Arrays, String
JavaScript: Understanding Arrays, Strings, setTimeout and setInterval
Introduction to JavaScript Arrays
An array is a special type of object in JavaScript that is used to store multiple values in a single variable. An array can hold many values under a single name and can be accessed via a reference to the array name along with the index of the specific value.
Key Points:
- Arrays are ordered, meaning that the items in an array have a specific order.
- Arrays can store values of different types (e.g., string, number, object, another array, function).
- JavaScript arrays are zero-indexed: the first element of an array is at index 0, and the last element is at the array's length minus 1.
Example:
let fruits = ['Apple', 'Banana', 'Mango'];
console.log(fruits[0]); // Outputs: Apple
Understanding JavaScript Strings
A string in JavaScript is a sequence of characters. It is used to represent text. Strings can be created as primitive values from string literals or as string objects using the String constructor.
Key Points:
- Strings are immutable, which means that the characters within them cannot be changed.
- The length of a string can be found by accessing the
.length
property.
Example:
let greeting = 'Hello, World!';
console.log(greeting.length); // Outputs: 13
Understanding setTimeout
The setTimeout
function in JavaScript is used to schedule code execution after a designated amount of milliseconds. This function is only executed once.
Key Points:
- The first argument is the function to be executed.
- The second argument is the delay in milliseconds (1000 ms = 1 second).
Example:
setTimeout(function() {
console.log('This message will appear after 4 seconds');
}, 4000);
Understanding setInterval
The setInterval
function in JavaScript is used to repeatedly execute a function, with a fixed time delay between each call. Unlike setTimeout
, setInterval
continues to call the function until clearInterval
is called or the window is closed.
Key Points:
- Like
setTimeout
, the first argument is the function to be executed, and the second is the delay.
- The function keeps executing at regular intervals until it is stopped.
Example:
let count = 0;
let intervalId = setInterval(function() {
count++;
console.log('This function has been called ' + count + ' times');
if (count == 5) {
clearInterval(intervalId);
}
}, 1000);
In this code, the function logs a message to the console every second (1000ms). After it has been called 5 times, the clearInterval
function is used to stop further calls.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version