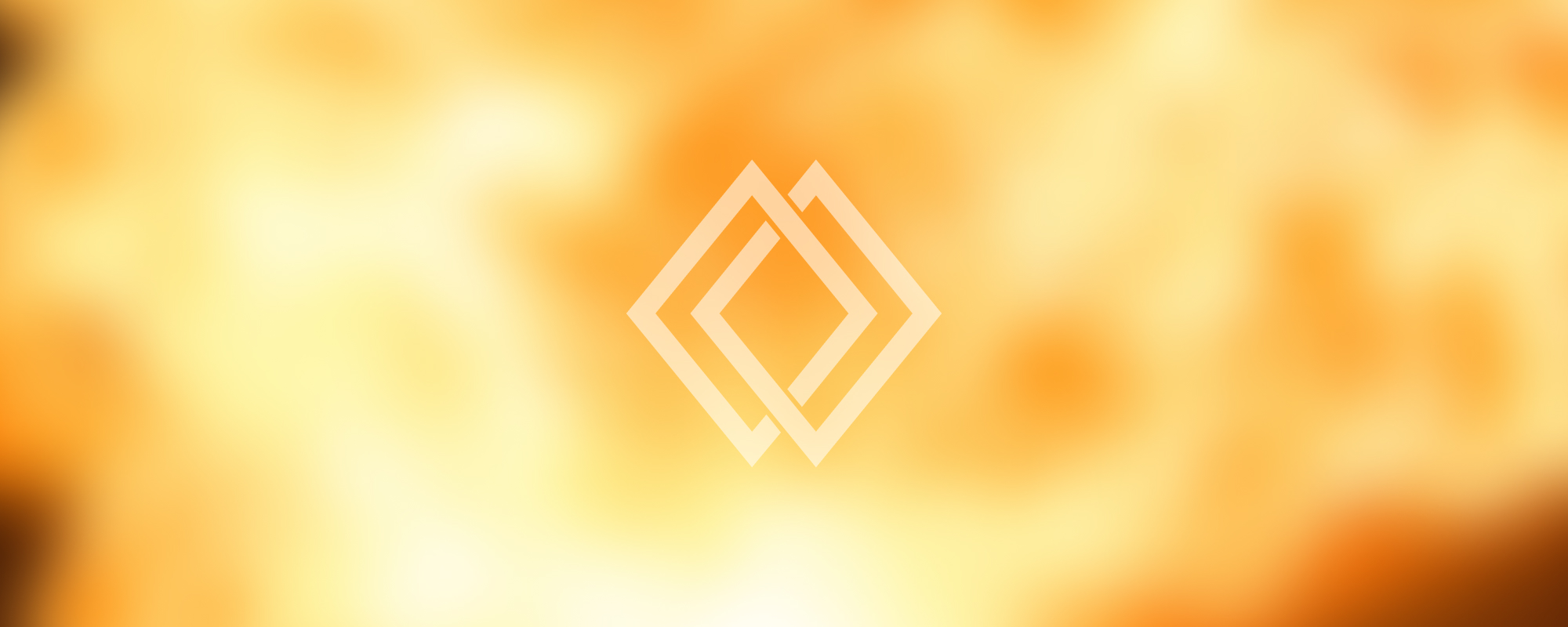
3. DOM Elements
JavaScript: Interacting with DOM Elements
Introduction to DOM Elements
In JavaScript, DOM (Document Object Model) elements represent the various components of an HTML or XML document. The DOM is essentially a tree-like structure that is built by the browser, which JavaScript can interact with to dynamically change the content, structure, and styles of a webpage.
Accessing DOM Elements
There are several methods available in JavaScript to access DOM elements, including:
getElementById()
: Selects an element based on its ID.
getElementsByClassName()
: Selects elements based on their class name.
getElementsByTagName()
: Selects elements based on their tag name.
querySelector()
: Selects the first element that matches a specified CSS selector.
querySelectorAll()
: Selects all elements that match a specified CSS selector.
These methods are used to select specific elements from the DOM, which can then be manipulated as needed.
Manipulating DOM Elements
Once a DOM element has been selected, JavaScript provides various properties and methods that can be used to manipulate it. Some examples include:
innerHTML
: Changes the content of an element.
style
: Changes the style of an element.
classList
: Allows you to add or remove classes from an element.
addEventListener()
: Adds an event listener to an element.
Here's an example of accessing a DOM element using the getElementById()
method and changing its content:
<!DOCTYPE html>
<html>
<head>
<title>DOM Elements Example</title>
</head>
<body>
<div id="myDiv">This is a div element.</div>
<script>
// Access the element with the ID "myDiv"
var myElement = document.getElementById("myDiv");
// Change the content of the element
myElement.innerHTML = "This content has been changed!";
</script>
</body>
</html>
In this example, we first select the element with the ID "myDiv" using getElementById()
. We then change the content of the element using the innerHTML
property.
Power of JavaScript with DOM
With the ability to interact with the DOM, JavaScript can:
- Change all HTML elements, attributes, and CSS styles on the page.
- Remove or add new HTML elements and attributes.
- Respond to all existing HTML events on the page.
- Create new HTML events on the page.
The Browser Object Model (BOM)
The Browser Object Model (BOM) is a larger representation of everything provided by the browser, which includes the window
object, the document
object (the DOM), and other objects that represent the browser's current state or provide access to the browser's functionality.
There are no official standards for the BOM, but modern browsers have implemented almost the same methods and properties for JavaScript interactivity, which are often referred to as methods and properties of the BOM.
The Window Object
The window
object is a global object that represents the browser's window. All global JavaScript objects, functions, and variables automatically become members of the window object. Even the document
object (of the HTML DOM) is a property of the window
object. For example, window.document.getElementById("myDiv")
is the same as document.getElementById("myDiv")
.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version