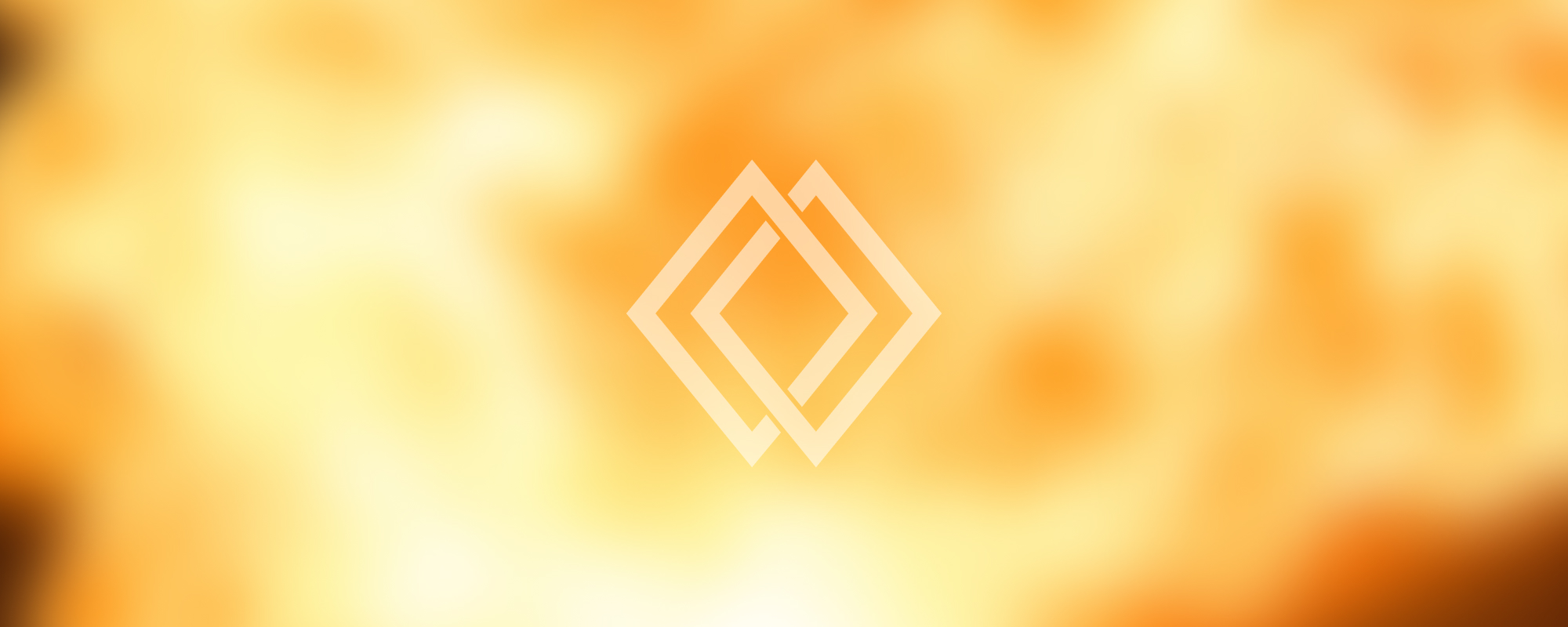
4. Slider Practise
Slider Practice (Carousels)
Introduction to Sliders (Carousels)
A Slider or Carousel is a popular UI component typically used to display a sequence of images or cards that can be browsed through by the user. This can be used either for a meaningful visual presentation or for engaging the user interactively on a website or an application.
Key Points:
- Sliders are often used to display multiple items in a limited amount of space.
- They can be automated or controlled manually by the user using navigation arrows or pagination bullets.
Implementing a Basic Slider
You can create a simple slider using HTML, CSS, and JavaScript. Here is a very basic example:
<!DOCTYPE html>
<html>
<head>
<style>
.slider {
display: flex;
overflow: auto;
scroll-snap-type: x mandatory;
scroll-padding: 50%;
scroll-behavior: smooth;
}
.slide {
flex: none;
border: 1px solid black;
scroll-snap-align: center;
width: 100%;
height: 100vh;
line-height: 100vh;
text-align: center;
font-size: 50px;
}
</style>
</head>
<body>
<div class="slider">
<div class="slide">1</div>
<div class="slide">2</div>
<div class="slide">3</div>
</div>
</body>
</html>
Slider Libraries
There are many JavaScript libraries available that can be used to create sliders. Some popular ones include:
Using these libraries, you can create more advanced sliders with a variety of configurations and features, such as autoplay, looping, and responsive breakpoints.
For example, here is how you would set up a basic slider using Swiper:
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="<https://unpkg.com/swiper/swiper-bundle.min.css>">
</head>
<body>
<div class="swiper-container">
<div class="swiper-wrapper">
<div class="swiper-slide">Slide 1</div>
<div class="swiper-slide">Slide 2</div>
<div class="swiper-slide">Slide 3</div>
</div>
<div class="swiper-pagination"></div>
<div class="swiper-button-next"></div>
<div class="swiper-button-prev"></div>
</div>
<script src="<https://unpkg.com/swiper/swiper-bundle.min.js>"></script>
<script>
var swiper = new Swiper('.swiper-container', {
pagination: { el: '.swiper-pagination', clickable: true, },
navigation: { nextEl: '.swiper-button-next', prevEl: '.swiper-button-prev', },
});
</script>
</body>
</html>
This code creates a slider with three slides, navigation arrows, and clickable pagination bullets. You can adjust the configuration options to customize the behavior of the slider as per your needs.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version