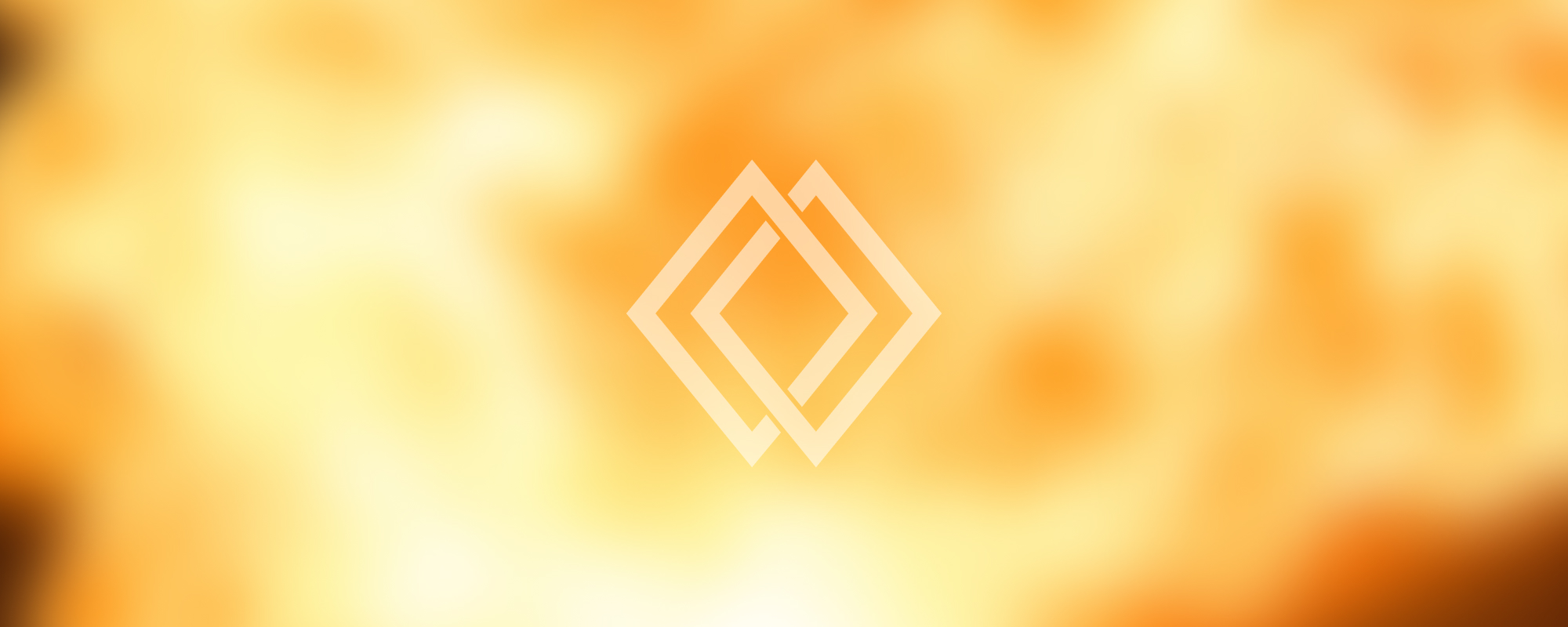
6. Work with Forms, Canvas
Working with Forms and Canvas in JavaScript
Introduction
This guide will demonstrate how to work with HTML forms and the HTML <canvas>
element using JavaScript.
HTML Forms
HTML forms are a critical component of interactive websites, allowing users to input data that can be sent to a server for processing.
Key Points:
- HTML forms are defined using the
<form>
tag
- Input types include: text, numbers, passwords, checkboxes, radio buttons, submit buttons, etc.
- Form data can be sent using GET or POST methods
Form Example:
Here's an example of a simple form with a text input and a submit button.
<form id="myForm">
<label for="fname">First name:</label><br>
<input type="text" id="fname" name="fname"><br>
<input type="submit" value="Submit">
</form>
In JavaScript, you can access the form and its data using the document.forms
property. Here's an example:
document.forms['myForm'].addEventListener('submit', function(event) {
event.preventDefault(); // prevent the form from being submitted
var name = document.forms['myForm']['fname'].value;
console.log('Form submitted! Name: ' + name);
});
HTML Canvas
The HTML <canvas>
element is used to draw graphics, on the fly, via JavaScript.
Key Points:
- The
<canvas>
tag creates a fixed-size drawing surface that exposes one or more rendering contexts.
- You can draw shapes, text, images, and other objects on the canvas.
- The canvas is initially blank and requires JavaScript to make it show anything.
Canvas Example:
Here's an example of a simple canvas with a rectangle drawn on it.
<canvas id="myCanvas" width="500" height="500" style="border:1px solid #d3d3d3;">
Your browser does not support the HTML5 canvas tag.
</canvas>
In JavaScript, you can access the canvas and its context to draw shapes or images. Here's an example:
var canvas = document.getElementById('myCanvas');
var ctx = canvas.getContext('2d');
ctx.fillStyle = '#FF0000';
ctx.fillRect(0, 0, 150, 75);
This JavaScript code gets a reference to the <canvas>
element, gets its context, sets the fill color to red, and then draws a rectangle at the top-left corner of the canvas.
In conclusion, forms and canvas are powerful tools in HTML and JavaScript that allow for user interaction and dynamic content. They are fundamental to creating interactive and visually engaging web applications.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version