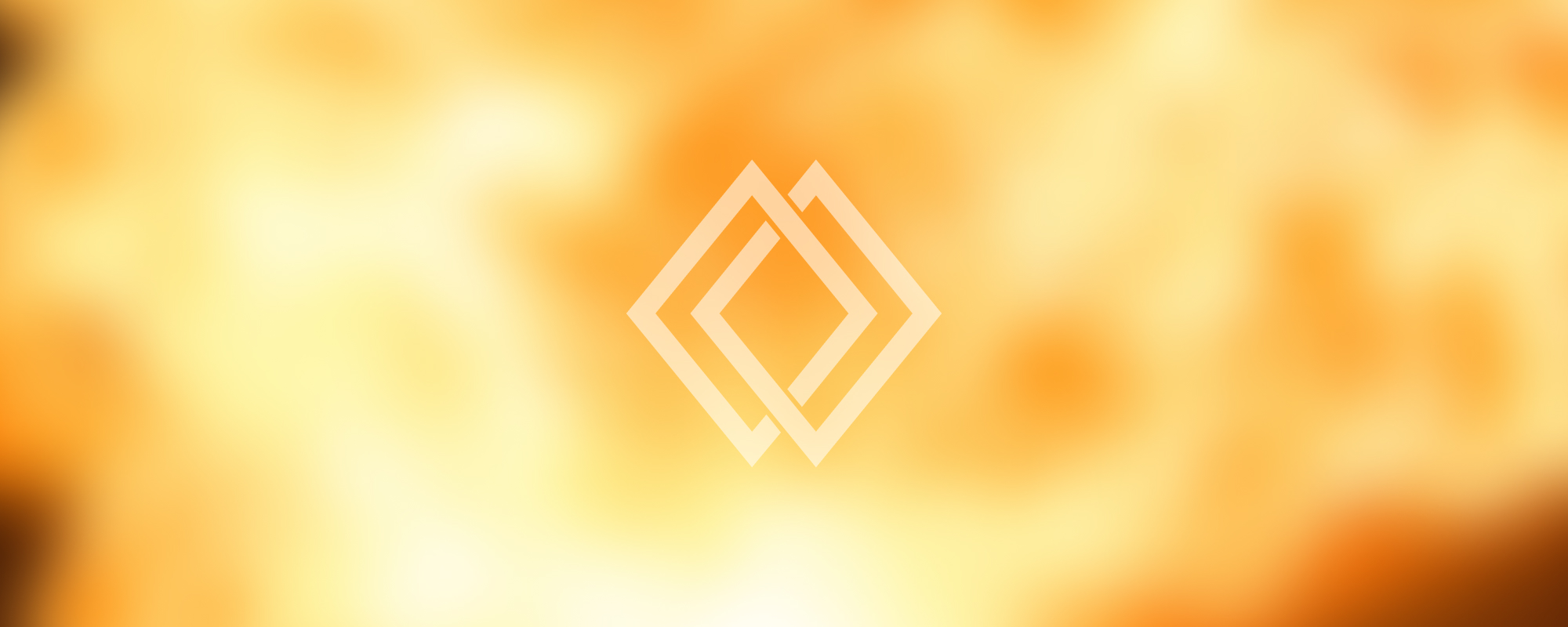
7. Async, Await, XHR
Async, Await, and XHR in JavaScript
Overview
This note covers important concepts in JavaScript related to asynchronous programming, such as XHR
(XMLHttpRequest), async/await
, Promises, and JSON (JavaScript Object Notation).
JSON (JavaScript Object Notation)
JSON is a syntax for storing and exchanging data in a lightweight data-interchange format. It is easy for humans to read and write, and easy for machines to parse and generate.
Key methods:
JSON.parse()
- Converts a JSON string into a JavaScript object.
JSON.stringify()
- Converts a JavaScript object or value to a JSON string.
Promises
A Promise in JavaScript is an object representing the eventual completion or failure of an asynchronous operation. Essentially, a promise is a returned object to which you attach callbacks, instead of passing callbacks into a function.
Key methods:
.then()
- Attaches callbacks for the resolution and/or rejection of the promise.
.catch()
- Attaches a callback for only the rejection of the promise.
.finally()
- Attaches a callback that will be invoked when the promise is settled, regardless of its outcome.
Example:
ForCallAsync().then(() => {
setTimeout(() => {
console.log("Completed callback");
}, 2000);
}).catch((e) => {
console.log(`Error : ${e}`);
}).finally(() => {
console.log("End");
});
Async/Await
async
and await
are additions to JavaScript that simplify working with Promises. An async
function is a function declared with the async
keyword, and the await
keyword can only be used inside an async
function. async
functions are instances of the AsyncFunction constructor, and the await
keyword is permitted within them.
XMLHttpRequest (XHR)
XMLHttpRequest (XHR) is an API available to web browser scripting languages such as JavaScript. It is used to send HTTP or HTTPS requests to a web server and load the server response data back into the script.
Code example:
// Querying a server
const url = "<http://127.0.0.1:5500//car.txt>";
let xhttp = new XMLHttpRequest();
xhttp.open("GET", url, true);
xhttp.onload = () => {
// for txt files
let data = xhttp.response;
// for json files
const obj = JSON.parse(xhttp.responseText);
};
xhttp.send();
Note: If you are unable to use arrow functions (=>
), you can replace them with the function()
keyword.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version