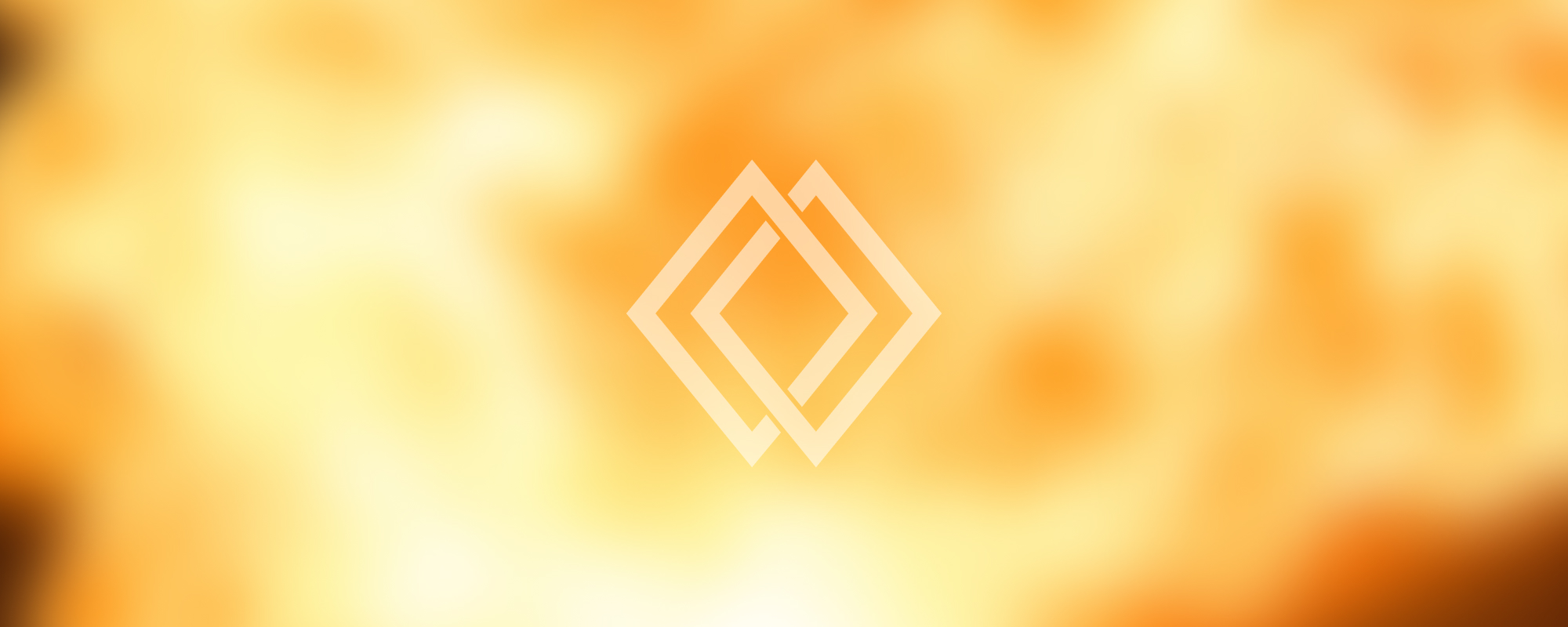
8. Java Script Fetch
JavaScript Fetch API
Introduction
The Fetch API is a modern interface in JavaScript that provides a powerful and flexible feature to fetch resources across the network. It returns promises and uses the same promise chaining concept you find in JavaScript Promises. It is easier to use and more powerful than the traditional XMLHttpRequest
.
GET Request
The GET method is used to retrieve information from the given server using a given URI. Fetch with a get request can be made as follows:
const requestUrl = "<https://jsonplaceholder.typicode.com/users>";
function sendRequest(method, url, body = null) {
return fetch(url).then((response) => {
return response.json();
});
}
sendRequest('GET', requestUrl)
.then((data) => {console.log(data)})
.catch((error) => console.log(error));
In this example, we define a function sendRequest
that accepts the HTTP method, URL, and body as parameters. We call the fetch
function with the URL. The fetch
function returns a promise that resolves to the Response object representing the response to the request. This response is parsed as JSON.
POST Request
The POST request method is used to send data to the server. An HTTP request consists of two parts, the header and the body. The header contains the metadata and the body contains the actual data (also known as the payload).
const requestUrl = "<https://jsonplaceholder.typicode.com/users>";
function sendRequest(method, url, body = null) {
const headers = {
'Content-type': 'application/json'
};
return fetch(url,
{
method: method,
body: JSON.stringify(body),
headers: headers
})
.then(response => {
return response.json();
});
}
const body = {
name: 'Ayxan',
age: '17'
};
sendRequest('POST', requestUrl, body)
.then((data) => {console.log(data)})
.catch((error) => console.log(error));
In this example, we define a function sendRequest
that accepts the HTTP method, URL, and body as parameters. We define headers with the 'Content-type' as 'application/json'. We then call the fetch
function with the URL and an options object. This options object includes the method, body, and headers. The body is stringified using JSON.stringify()
before sending. The fetch
function returns a promise that resolves to the Response object representing the response to the request. This response is parsed as JSON.
Summary
Fetch makes it easier to make web requests and handle responses than with the older XMLHttpRequest
, which often requires additional logic for different data formats. It is versatile and modular, bringing a range of new possibilities for fetching resources.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version