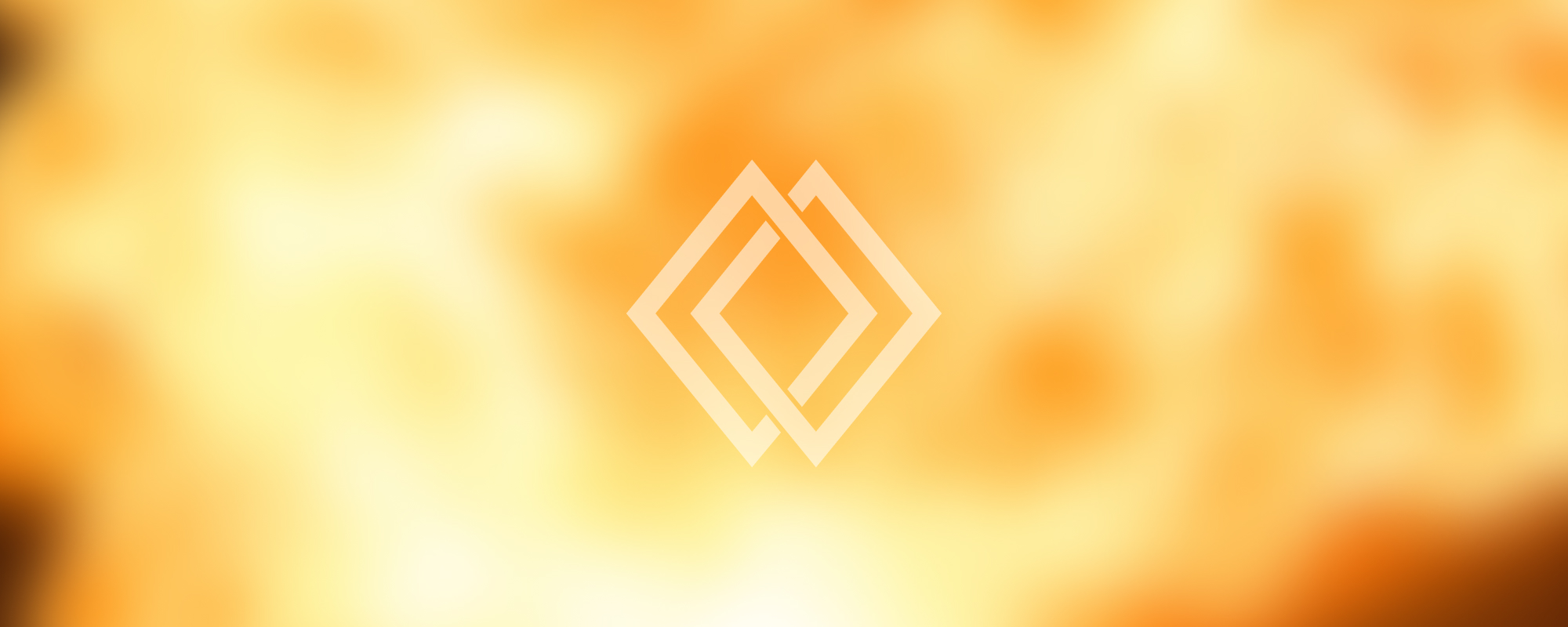
9. JQuery
jQuery in JavaScript
Introduction to jQuery
jQuery is a fast, small, and feature-rich JavaScript library. It makes things like HTML document traversal and manipulation, event handling, and animation much simpler with an easy-to-use API that works across a multitude of browsers. With a combination of versatility and extensibility, jQuery has changed the way that millions of people write JavaScript.
Key Points:
- jQuery is a powerful tool for manipulating HTML documents.
- It handles events, creates animations, and assists with AJAX interactions in a browser.
- jQuery code is succinct and easier to write than raw JavaScript.
AJAX in jQuery
AJAX (Asynchronous JavaScript and XML) is a technique for creating fast and dynamic web pages. AJAX allows web pages to be updated asynchronously by exchanging small amounts of data with the server behind the scenes. This means that it is possible to update parts of a web page, without reloading the whole page.
AJAX in jQuery is a set of methods that allow you to perform AJAX requests with less code compared to raw JS AJAX calls.
Key Points:
- AJAX allows asynchronous communication with the server.
- It makes it possible to update parts of a web page without reloading the entire page.
- jQuery provides a simpler interface for making AJAX calls.
Accessing HTML Elements with jQuery
In jQuery, you can select HTML elements much like you would in CSS. The $
function is used to select elements. For example, $(".pages")
selects all elements with the class pages
.
Example:
// Select all elements with class 'pages'
var pages = $(".pages");
// Change the text color of these elements to red
pages.css("color", "red");
This code selects all elements with the class pages
and changes their text color to red. This is much simpler and more intuitive than using raw JavaScript.
Relationship Between jQuery and JavaScript
jQuery is a library built on top of JavaScript to provide a simpler and more intuitive interface for common JavaScript tasks. It is not a separate language, but a tool that makes writing JavaScript easier and more efficient.
Remember:
- jQuery simplifies tasks like HTML manipulation, event handling, and AJAX.
- jQuery is a library of JavaScript, not a separate language.
- jQuery code is usually shorter and easier to understand than raw JavaScript code.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version