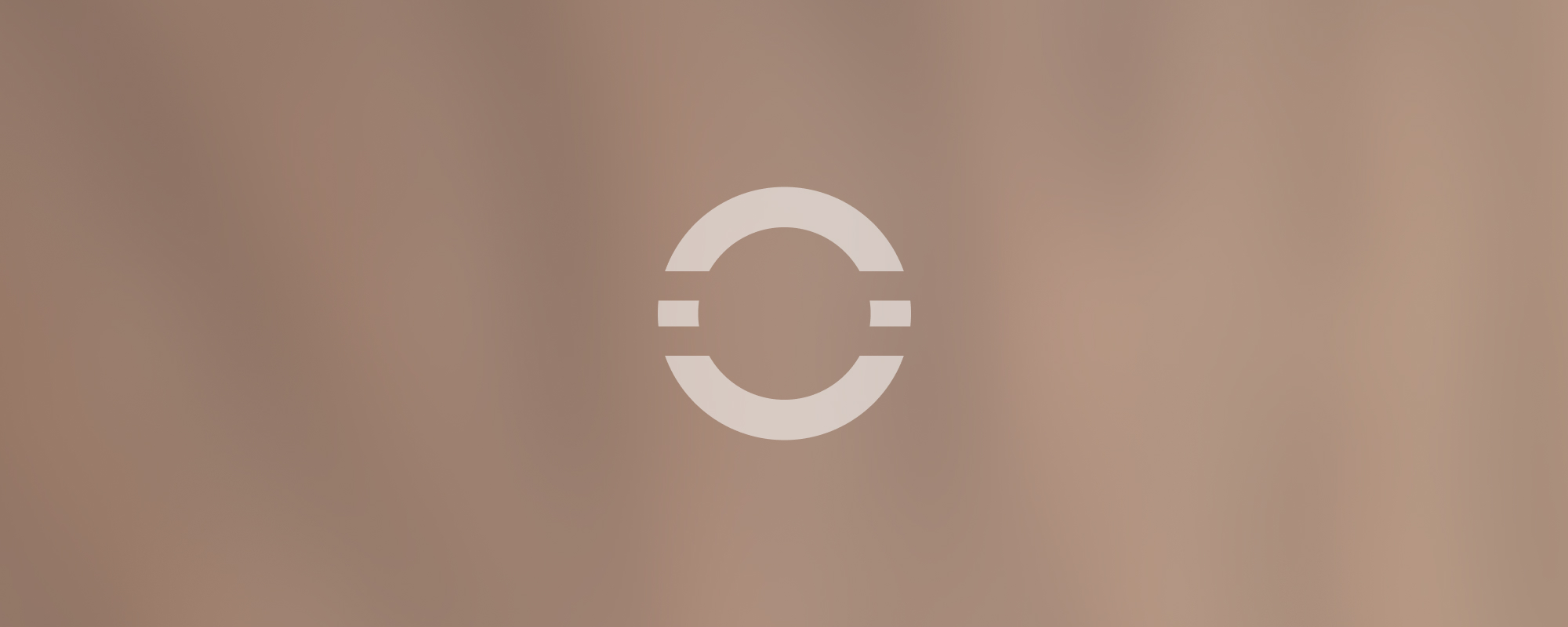
1. Introduction to Network Programming
Introduction to Network Programming
Overview
Network Programming is the process of writing software that allows multiple computers to communicate and exchange data. It involves the use of protocols and technologies to achieve reliable communication between different points on a network. This makes it possible to share resources and information across different systems.
Defining Network Programming
Network programming is the practice of designing and implementing software applications that communicate over a network. It involves creating and managing connections between local and remote endpoints.
Key Concepts in Network Programming
EndPoints
An EndPoint in network programming refers to an entity that participates in network communication. It is composed of an IP (Internet Protocol) address and a port number. An endpoint can be categorized into:
- Local EndPoint: The endpoint where the communication originates.
- Remote EndPoint: The endpoint to which data is being sent.
Port
In the context of network programming, a port is a communication endpoint that can send or receive data. It can be likened to a door that allows data to enter or exit.
IP (Internet Protocol)
An IP is a unique address that identifies a device on the internet or a local network. It serves a similar function to the address of a house, with the ports serving as doors and windows.
Server
A server is a computer or system that provides resources, data, services, or programs to other computers, known as clients, over a network. In terms of network programming, a server is a program or device that accepts and responds to requests made over a network.
Network Models
(Network Models content to be expanded...)
Socket
A Socket is a class in C# (.Net's network class) used for network communication.
Network Programming in C#
The .NET Framework provides a comprehensive set of classes for network programming. The Socket
class is one of the fundamental classes for networking in C#. It provides methods for sending and receiving data over a network.
Example:
Socket s = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
s.Connect(new IPEndPoint(IPAddress.Parse("127.0.0.1"), 80));
Types of Network Communications
Unicast
Unicast communication is a one-to-one connection between the client and the server.
Multicast
Multicast communication is a form of communication where a single sender communicates with multiple receivers at the same time.
Broadcast
Broadcast communication is a type of communication where a single packet of data is sent from one point to all other points. In this case, there is one sender, and the information is sent to all connected receivers.
Network Protocols
TCP (Transmission Control Protocol)
TCP is a connection-oriented protocol that guarantees the delivery of packets in the order they were sent.
UDP (User Datagram Protocol)
UDP is a connectionless protocol. Unlike TCP, it doesn't guarantee the delivery or the order of packets.
RDP (Reliable Datagram Protocol)
RDP is a protocol that solves the problems of UDP by adding a sequence number to each packet. This allows the receiver to reorder the packets correctly.
FTP (File Transfer Protocol)
FTP is a standard network protocol used to transfer computer files between a client and server on a computer network.
HTTP (Hypertext Transfer Protocol)
HTTP is the foundation of any data exchange on the Web, and it is a protocol used for transmitting hypertext over the internet.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version