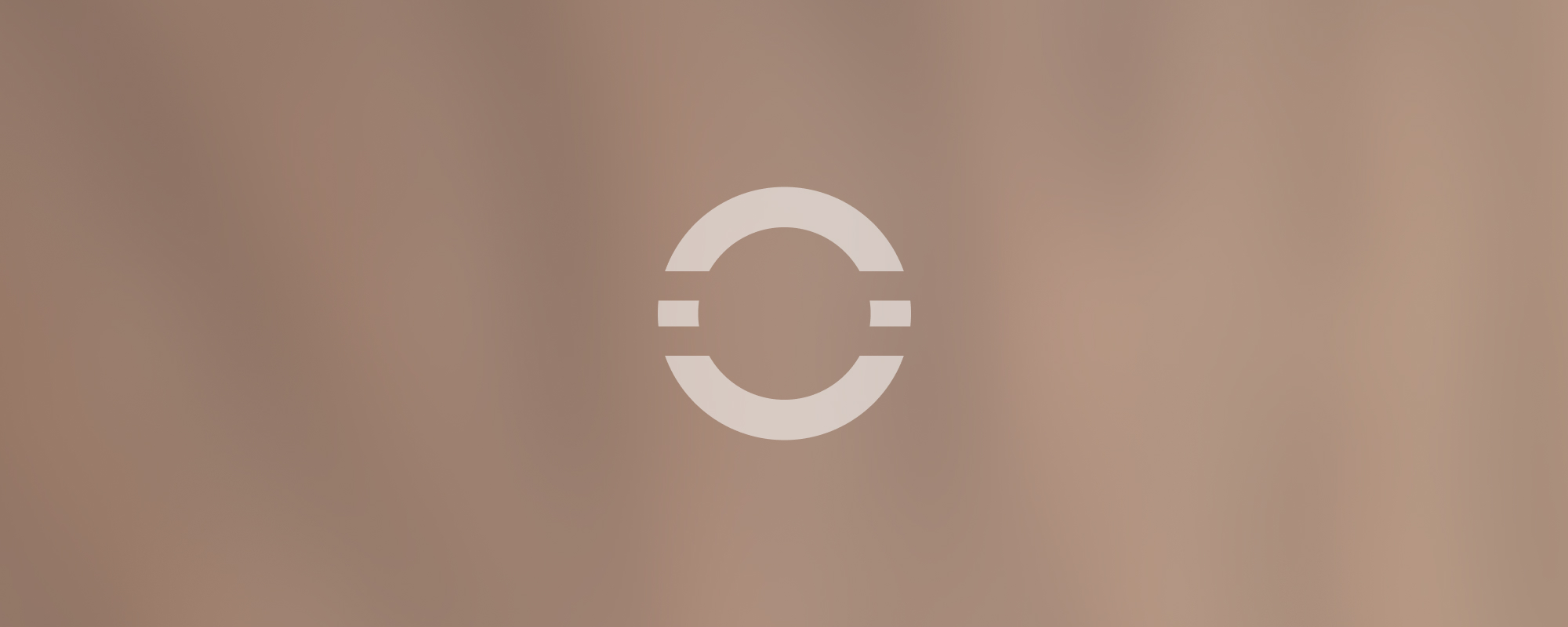
3. UDP
Understanding User Datagram Protocol (UDP)
Introduction to UDP
The User Datagram Protocol (UDP) is a core member of the Internet protocol suite. It is a transport protocol that sends data without checking if the data is received at the destination. This makes it faster than other protocols, such as TCP, since it doesn't spend time checking if data packets have reached their intended destination.
Key Features of UDP
Speed
As mentioned, the UDP protocol is faster than its counterparts because it doesn't check whether data has been successfully received. It simply sends the data and moves on, hence saving time.
No Error-checking or Recovery
UDP does not perform error-checking or recovery of packets. If a packet is lost or corrupted during transmission, UDP does not provide any mechanism to detect or recover it.
Unreliable
Due to the lack of error-checking and recovery mechanisms, UDP is often referred to as an "unreliable" protocol. However, this does not necessarily mean it's inferior - it simply means that it's better suited for applications where speed is more important than reliability.
How UDP Works
Here's an example of how data is sent using UDP:
import socket
# Create a UDP socket
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
# Define the server address and port
server_address = ('localhost', 12345)
# Message to be sent
message = b'This is our message.'
# Send data
print('Sending {!r}'.format(message))
sent = sock.sendto(message, server_address)
In this example, we create a UDP socket, define the server address and port, then send a message to that server. Note that there's no check to see whether the data was received or not.
When to Use UDP
While UDP is not suitable for all types of applications, it is an ideal choice for real-time applications such as live streaming, online games, and VoIP where speed is crucial and some data loss is acceptable.
Conclusion
UDP is a simple, connection-less protocol that prioritizes speed over reliability. Despite its unreliability, it is employed in various applications where speed is paramount. Understanding its workings and appropriate use cases is important in networking and application development.
Remember, the use of UDP or another protocol like TCP will largely depend on the type of application you're developing and its specific requirements. It's all about making the right choice based on the nature of the data being transmitted and the acceptable margin of error.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version