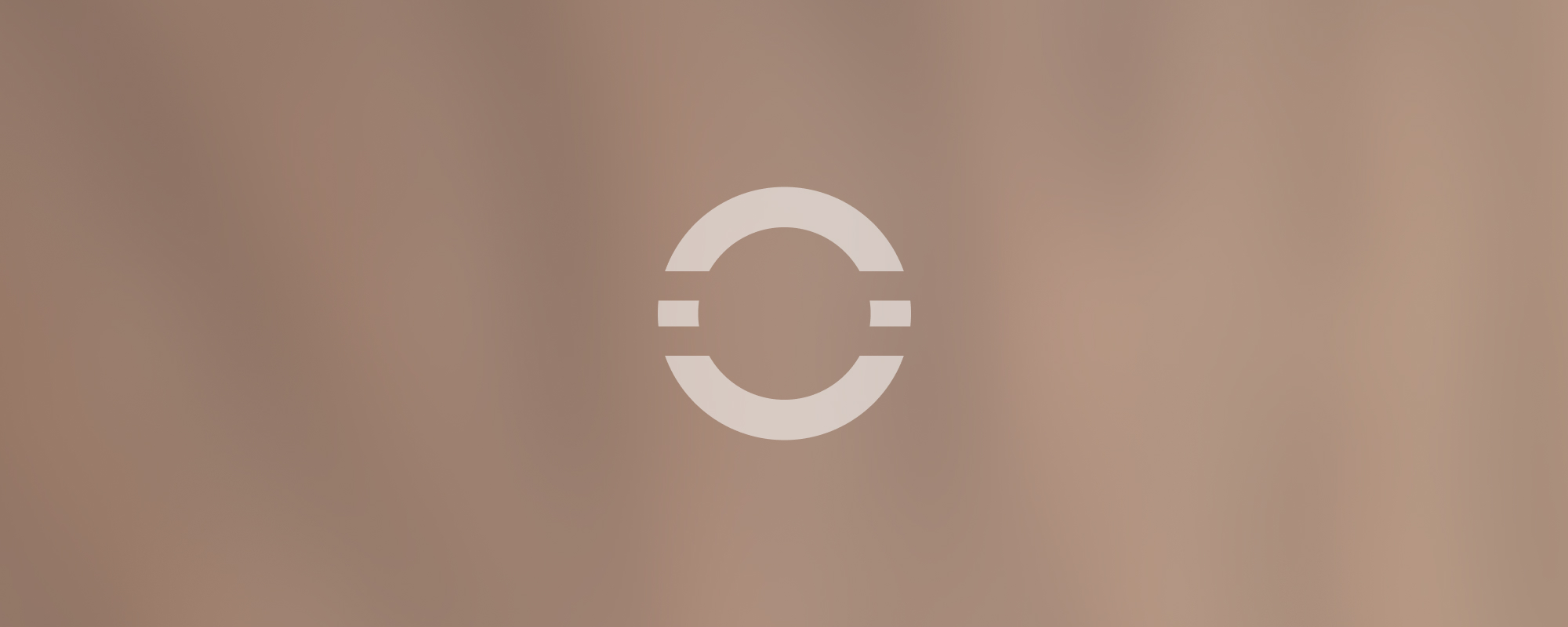
4. Monitor Live Project
Monitor Live Project
Monitoring live projects involves the real-time tracking and managing of ongoing projects and applications. It is crucial to keep an eye on the performance of your application, identify any potential issues, and make necessary improvements. This process can be done manually, or with the help of monitoring tools.
One way to monitor live projects is to create your own Team Viewer App. Team Viewer is a popular software that allows users to remotely access and manage devices. Building a similar app can provide customized control and monitoring over your projects.
Creating Your Own Team Viewer App
Building your own Team Viewer-like application involves several key steps. This includes setting up the remote connection, ensuring secure data transmission, and designing the user interface for easy navigation.
Key Points:
- A Team Viewer-like app facilitates remote access and management of devices.
- It should provide real-time updates and reports on the system's performance.
- Security measures should be put in place to protect data from unauthorized access.
Setting Up the Remote Connection
The first step in creating your own Team Viewer-like app is setting up the remote connection. This involves configuring your app to connect to remote devices and retrieve real-time data.
Example:
public class RemoteConnection {
public void connectToRemoteDevice(String deviceID){
// Connect to the remote device using the device ID
}
}
public class App {
public static void main(String[] args) {
RemoteConnection remoteConnection = new RemoteConnection();
remoteConnection.connectToRemoteDevice("12345678");
}
}
Ensuring Secure Data Transmission
When dealing with remote connections, it's crucial to ensure secure data transmission. This prevents unauthorized access and maintains the integrity of the data.
Example:
public class SecureDataTransmission {
public void transmitData(String data){
// Encrypt the data before transmission
}
}
public class App {
public static void main(String[] args) {
SecureDataTransmission secureDataTransmission = new SecureDataTransmission();
secureDataTransmission.transmitData("Sensitive Information");
}
}
Designing User Interface
The user interface is crucial for ease of navigation and user experience. It should provide a clear overview of the system, real-time updates, and easy access to control features.
public class UserInterface {
public void displaySystemOverview(){
// Display the system overview
}
public void displayRealtimeUpdates(){
// Display real-time updates
}
public void displayControlFeatures(){
// Display control features
}
}
public class App {
public static void main(String[] args) {
UserInterface userInterface = new UserInterface();
userInterface.displaySystemOverview();
userInterface.displayRealtimeUpdates();
userInterface.displayControlFeatures();
}
}
By successfully integrating these components, you can build an application that can effectively monitor live projects and provide a customized and controlled environment for project management.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version