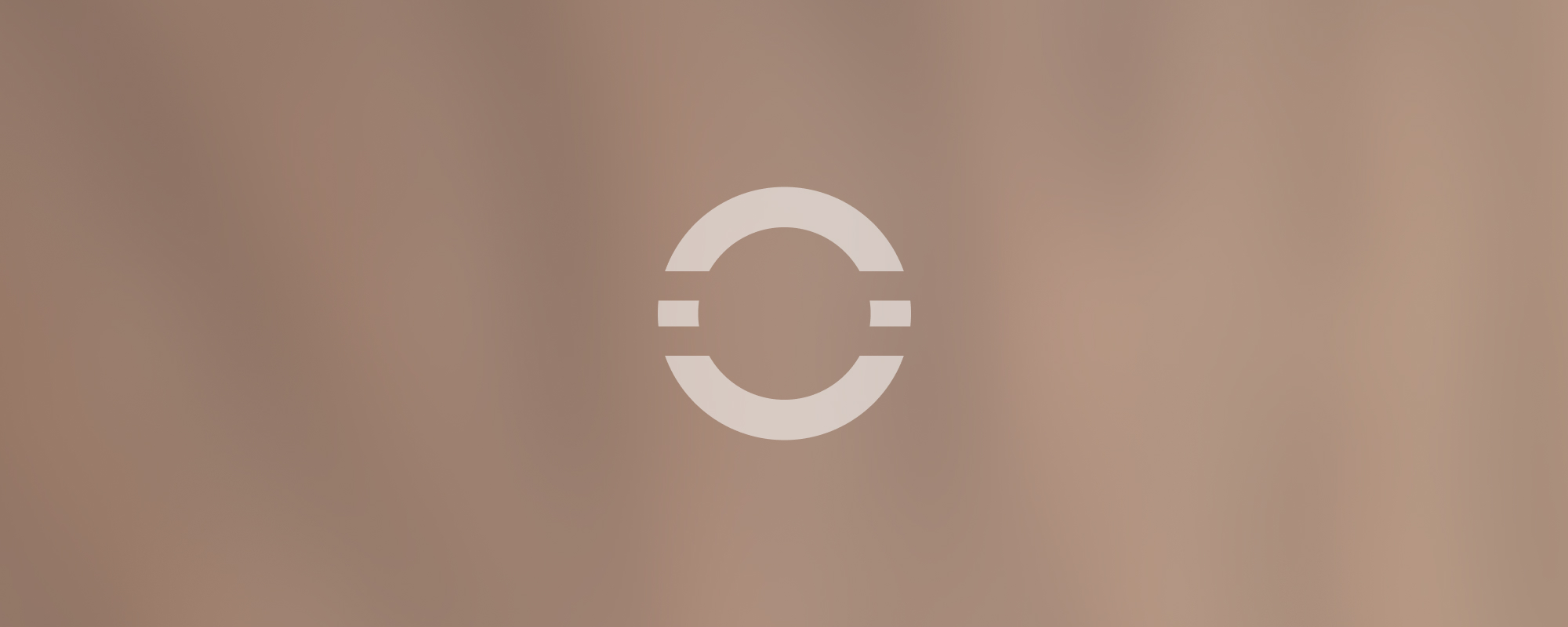
5. Tic Tac Toe
Tic Tac Toe Game in WPF
Introduction
Tic Tac Toe is a classic two player game also known as Noughts and Crosses or Xs and Os. The aim of the game is to complete a line of three marks either horizontally, vertically, or diagonally on a 3x3 grid. In this guide, we will be creating a simple Tic Tac Toe game using Windows Presentation Foundation (WPF) framework.
What is WPF?
WPF (Windows Presentation Foundation) is a free and open-source graphical subsystem originally developed by Microsoft for rendering user interfaces in Windows-based applications. WPF uses XAML (an XML-based language) for designing the user interface.
Key Points:
- WPF supports both vector and raster graphics.
- It allows developers to use hardware acceleration for rendering graphics.
- WPF introduces a new type of layout system.
Creating a Simple WPF Application
Before we delve into creating the Tic Tac Toe game, let's first discuss how to create a simple WPF Application.
// To create a new WPF Application in Visual Studio:
// 1. Click on `File -> New -> Project...`.
// 2. In the `New Project` dialog box, click on `WPF Application`.
// 3. Enter a name for the project and click `OK`.
A new WPF Application includes two main files: MainWindow.xaml
and MainWindow.xaml.cs
. The .xaml
file is used to design the user interface and the .cs
file is used for the logic behind the interface.
Designing Tic Tac Toe Game Interface
Designing our Tic Tac Toe game interface involves creating a 3x3 grid of buttons on which the players will make their moves.
<!-- MainWindow.xaml file -->
<Grid>
<Button x:Name="btn1" Click="Button_Click" />
<Button x:Name="btn2" Click="Button_Click" />
<Button x:Name="btn3" Click="Button_Click" />
<!-- Remaining buttons go here -->
</Grid>
Implementing Tic Tac Toe Logic
// MainWindow.xaml.cs file
bool turn = true; // true = X turn, false = O turn
int turnCount = 0;
private void Button_Click(object sender, RoutedEventArgs e)
{
Button b = (Button)sender;
b.Content = turn ? "X" : "O";
b.IsEnabled = false;
turn = !turn;
turnCount++;
CheckForWinner();
}
private void CheckForWinner()
{
bool thereIsAWinner = false;
// Horizontal checks
if (btn1.Content == btn2.Content && btn2.Content == btn3.Content && !btn1.IsEnabled)
thereIsAWinner = true;
// Remaining checks go here
if (thereIsAWinner)
{
string winner = turn ? "O" : "X";
MessageBox.Show($"{winner} Wins!", "Yay!");
}
else if (turnCount == 9)
{
MessageBox.Show("Draw!", "Bummer!");
}
}
The Button_Click
function is called when a button is clicked. It sets the button's content to "X" or "O" depending on the current player's turn, disables the button to prevent further clicks, and calls the CheckForWinner
function to check if there's a winner after each move.
The CheckForWinner
function checks if there's a winner by comparing the content of the buttons. If all buttons in a row, column or diagonal have the same content and are disabled (indicating a complete move), then there's a winner. If all buttons are disabled and there's no winner, it's a draw.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version