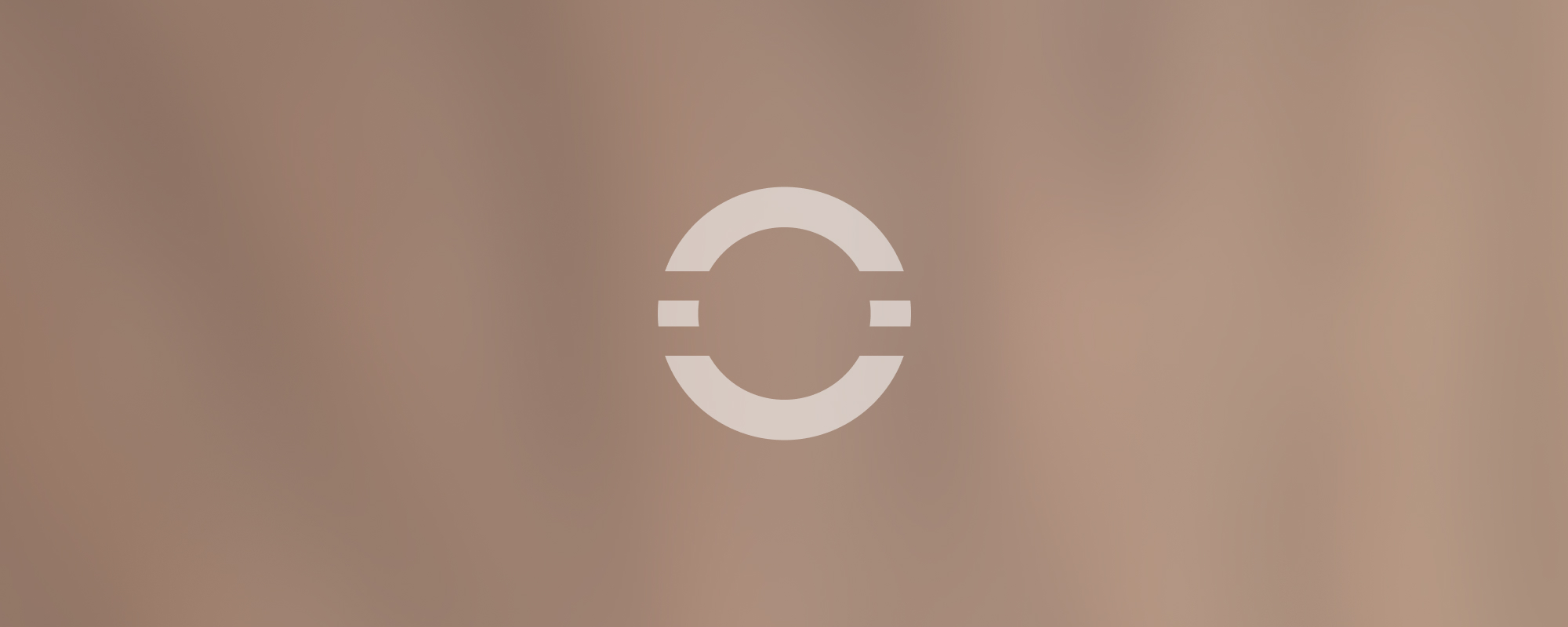
6. Monolith Structure, As Server, UI Clients
Monolith and Microservice Structures
This lesson explores the concepts of Monolith and Microservice structures. It explains how these structures are used in application development and the roles they play in complex project management.
Monolith Structure
A Monolith structure is a software architecture style where a single, self-contained unit holds the entirety of an application's server-side code. All the functionality of an application — data access layer, business logic, and client-side user interface — are tightly coupled and run in a single process, often on a single server.
Application Server
In a Monolith structure, the application server, often denoted as App.Server
, is the central unit where all the server-side operations occur.
Business Layer
The business layer or App.Business
holds the necessary services for business logic. This is where the application's main functionality resides. It contains the rules and processes that the application follows.
Example:
public class BusinessLayer {
// Business Logic
}
Core Layer
The core layer or App.Core
is the heart of the application. It contains the main business logic and rules.
Data Access Layer
The Data Access Layer (DAL) or App.DataAccess
is where the classes for database access reside. It contains methods for interacting with the database.
Example:
public class DataAccessLayer {
// Database Access Methods
}
Entities Layer
The Entities layer or App.Entities
holds all the classes, such as Car, Human, etc. It's where we store various entities or objects that the application interacts with.
Example:
public class Car {
// Car class
}
public class Human {
// Human class
}
Microservice Structure
A Microservice structure is a method of developing software systems that focuses on building single-function modules with well-defined interfaces and operations. The goal of a Microservice structure is to simplify complex projects by breaking them down into smaller applications, each with its own Monolith structure.
The applications in a Microservice structure can be thought of as small, individual monoliths that work together to form a larger, more complex application.
Example:
public class Microservice {
// Microservice Logic
}
In summary, the Monolith and Microservice structures are two different approaches to organizing server-side code. The Monolith structure is a traditional, all-in-one approach, while the Microservice structure breaks down complex applications into smaller, manageable pieces.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version