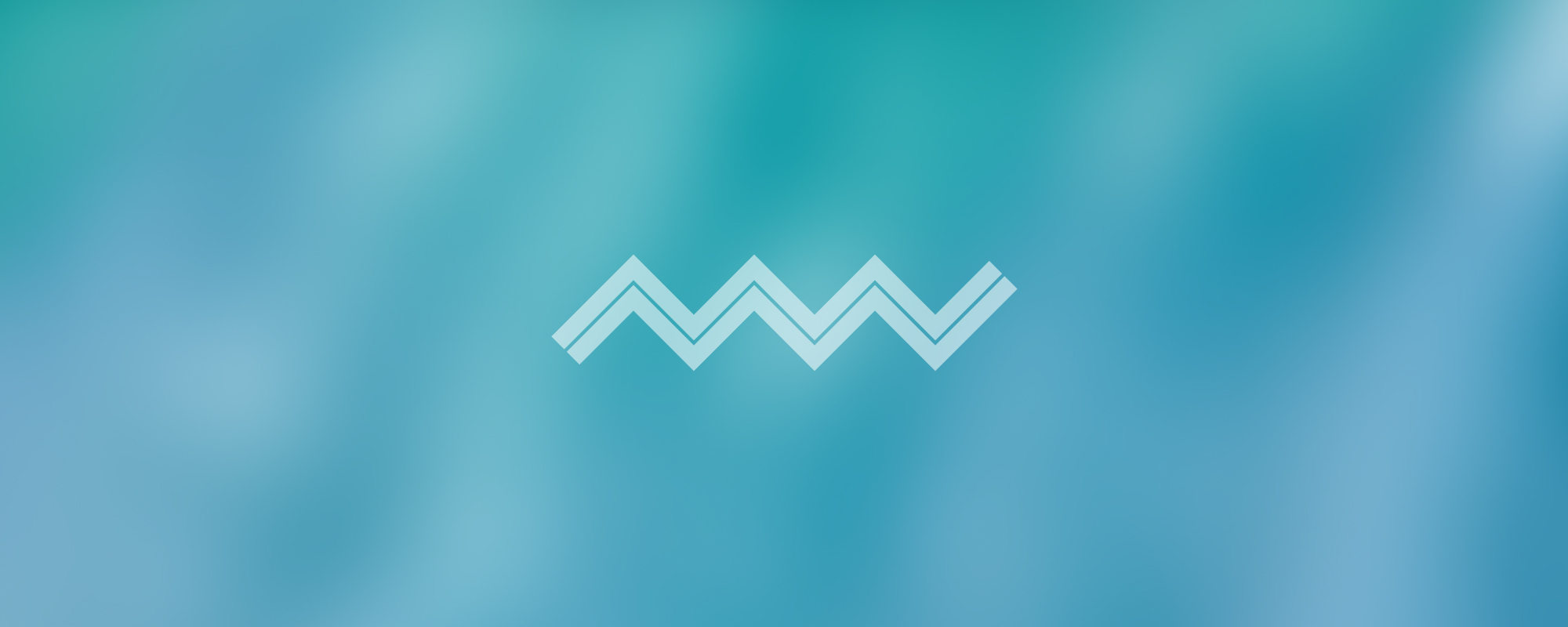
1. Introduction to React
Introduction to React
Overview
React is a powerful JavaScript library that enhances the functionality of web applications, making them more efficient and responsive. It was developed by Facebook and is renowned for its Single Page (SP) technology, making it an ideal choice for modern web development.
Key Features of React
Virtual DOM (VDOM)
React employs a concept known as the Virtual DOM (VDOM). It only renders parts of the web page that have changed, without refreshing the whole page. This results in improved performance and a smoother user experience.
JSX - JavaScript and XML
React introduces JSX (JavaScript and XML), which allows HTML code to be written within JavaScript. This feature makes it easier to create complex UI components.
Redux
Redux is a separate package that helps manage the state of an application. Although Redux is not tied directly to React, they work beautifully together, hence the term "React Redux" is often used.
Node.js Runtime Environment
With the advent of Node.js, it's now possible to write back-end code using JavaScript. This makes JavaScript a full-stack language and further increases its utility in web development.
React Development Environment
Installing Necessary Packages
Before starting with React, certain packages need to be installed for a better development experience:
Auto Rename Tag
: Automatically renames paired HTML/XML tags.
Bracket Pair Colorization Toggler
: Helps in color-matching scopes.
ES7 React/Redux/GraphQL/React-Native snippets
: Helps in debugging JavaScript code.
ESLint
: Enhances code quality.
Prettier - Code formatter
: Formats code for better readability.
To install these packages, use the following commands:
npm install -g create-react-app
npx create-react-app intro
React Components
In React, data can be passed from a Parent Component to a Child Component. However, data cannot be passed from a Child Component to a Parent Component. This limitation can be overcome using React Redux.
Component Drilling
The process of passing data from the Parent Component to a grandchild Component (i.e., from one child component to another child component) is known as Component Drilling.
Reactstrap
Reactstrap is a library that turns Bootstrap classes into React components. For instance, <div class=”container”></div>
in Bootstrap becomes <Container></Container>
in Reactstrap.
To install Reactstrap and Bootstrap, use the following commands:
npm install reactstrap
npm install bootstrap
Example:
import React from "react";
import { Container } from "reactstrap";
const Example = () => {
return (
<Container>
This is a Container component in Reactstrap.
</Container>
);
};
export default Example;
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version