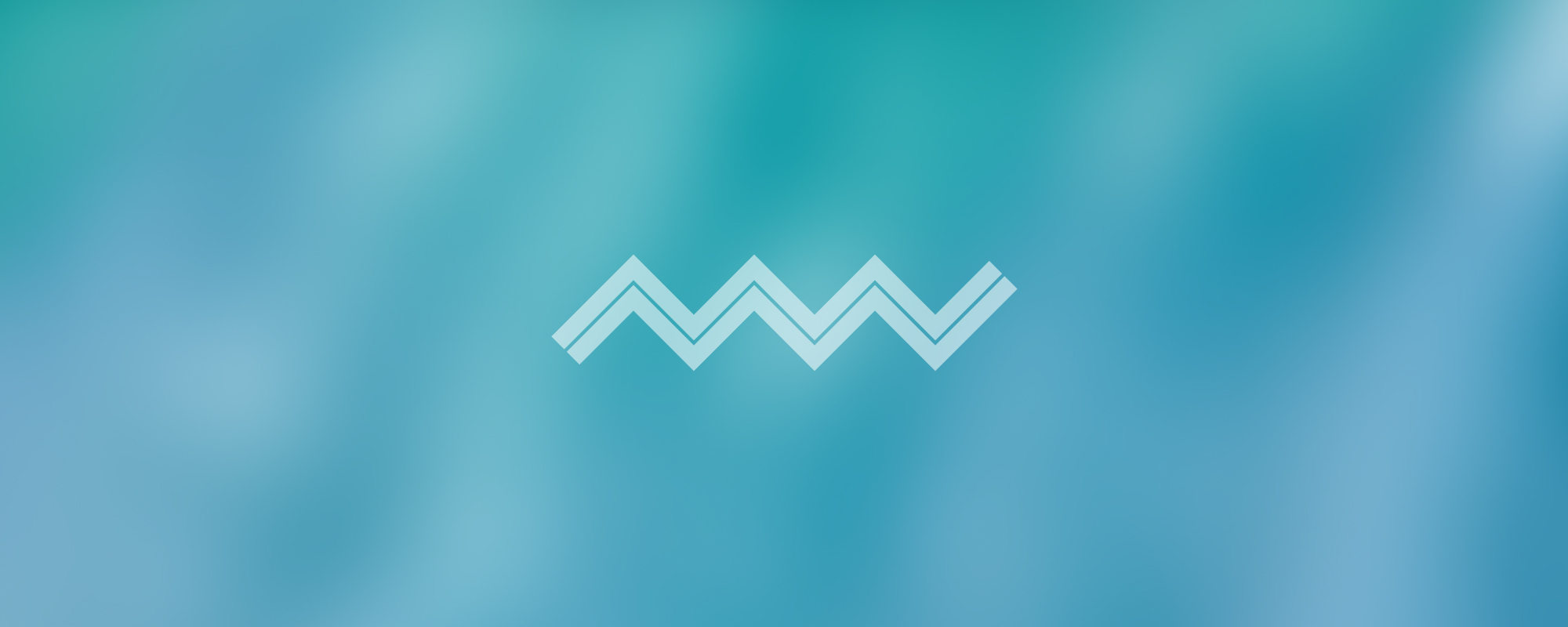
10. UseEffect
React Hooks: useEffect
Introduction
useEffect is a React Hook that allows you to perform side effects in function components. It is used to synchronize a component with an external system and can be used when receiving a response from an API, connecting to a network, etc.
Understanding Side Effects
A side effect is a term originating from the world of functional programming, and it refers to an operation that modifies the state of the system outside the function scope. In React, this could be anything that interacts with the outside world (API calls, timers, manual DOM manipulations, etc.) or the component state.
Using useEffect
useEffect is called after the component renders. Depending upon its dependency list (the second argument to useEffect), it can run after every render or only when certain state/props change.
Here is an example of how to use useEffect:
function VideoPlayer({src, isPlaying}){
const ref = useRef(null);
useEffect(() => {
if (isPlaying){
ref.current.play();
}
else{
ref.current.pause();
}
})
return <video ref={ref} src={src}></video>
}
In the VideoPlayer
component above, after the component renders, the code block inside useEffect
will run. It checks whether the video should be playing or paused based on the isPlaying
prop and acts accordingly.
Dependencies in useEffect
The second argument to useEffect is an array of dependencies. useEffect will only re-run if any of the dependencies have changed since the last render. Here is how it can be used:
useEffect(() => {
// Code to run on change of VAR1 or VAR2
}, [VAR1, VAR2])
In the above example, the code block inside useEffect
will run whenever VAR1
or VAR2
changes.
Conclusion
Understanding useEffect
and its usage is crucial for managing side effects in React. It allows you to handle component synchronization with external systems and execute code based on changes in your component's state or props.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version