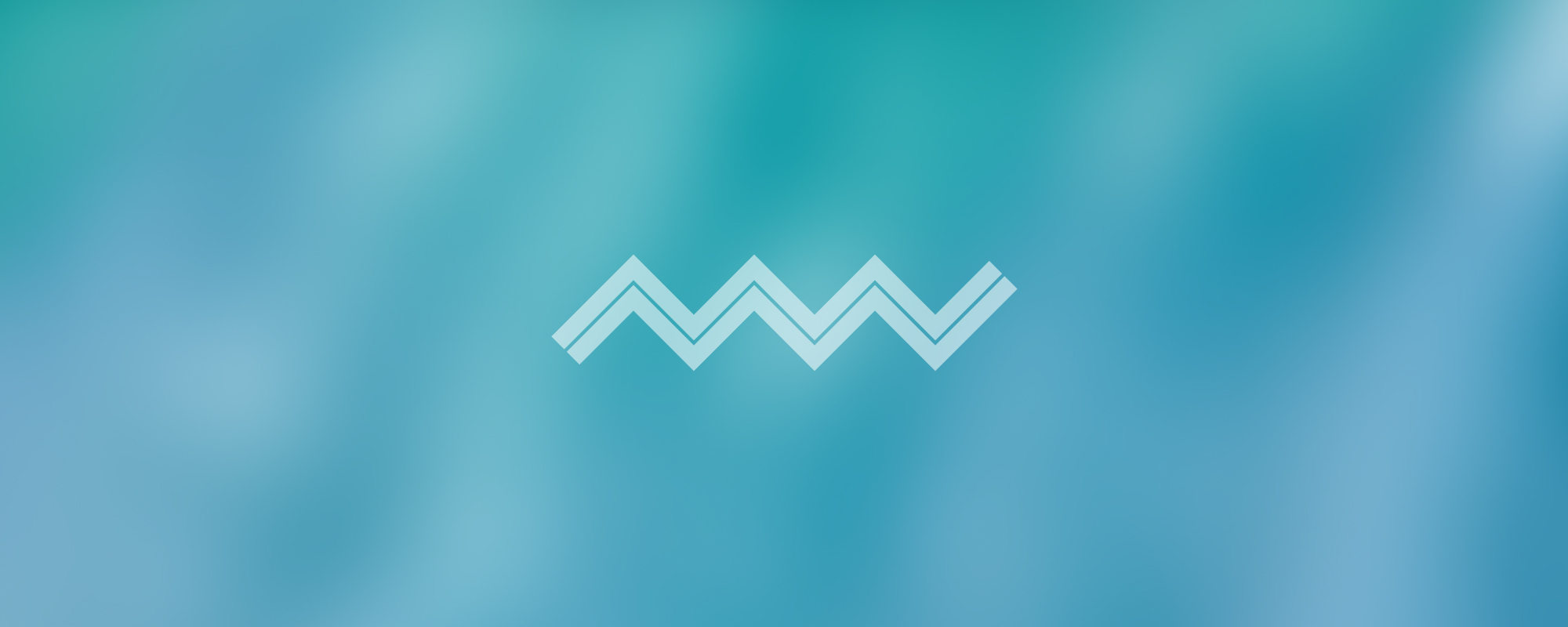
11. UseContext, HTTP Request, Axios
Understanding UseContext, HTTP Request, and Axios in React
Introduction to useMemo Hook in React
Definition & Purpose
useMemo is a built-in hook in React that is used to optimize performance. It memoizes the result of an expensive function and returns the memoized result whenever the function is called with the same inputs. This prevents the function from being executed each time the component renders, hence improving performance.
Key Points:
- useMemo is used for optimization by preventing expensive function calls during every render.
- useMemo will only recompute the memoized value when one of the dependencies has changed.
- Syntax:
useMemo(() => expensiveFunction(), [dependencies]);
Code Example
import React, { useState, useMemo } from 'react';
function App() {
const [number, setNumber] = useState(0);
const [dark, setDark] = useState(false);
const doubleNumber = useMemo(() => {
return slowFunction(number);
}, [number]);
const themeStyles = useMemo(() => {
return {
backgroundColor: dark ? 'black' : 'white',
color: dark ? 'white' : 'black'
}
}, [dark])
return (
<div style={themeStyles}>
<input type="number" value={number} onChange={e => setNumber(parseInt(e.target.value))} />
<button onClick={() => setDark(prevDark => !prevDark)}>Change Theme</button>
<div>{doubleNumber}</div>
</div>
);
}
function slowFunction(num) {
// simulate a slow function
for(let i = 0; i <= 1000000000; i++) {}
return num * 2;
}
export default App;
Utilizing CreateContext in React
Definition & Purpose
CreateContext is a part of React's Context API that is used to avoid prop drilling. It allows us to create a context, which can be thought of as a global data store that can be accessed from any component in the component tree without explicitly passing the data around.
Key Points:
- It is a method on the Context API.
- It allows us to pass data directly from a parent component to a deeply nested child component.
- Syntax:
const MyContext = React.createContext(defaultValue);
Code Example
import React, { createContext, useContext } from 'react';
// Create a Context
const NumberContext = createContext();
function App() {
return (
<NumberContext.Provider value={42}>
<div>
<Display />
</div>
</NumberContext.Provider>
);
}
function Display() {
const value = useContext(NumberContext);
return <div>The answer to the universe is {value}.</div>;
}
export default App;
Understanding Axios and HTTP Requests
Definition & Purpose
Axios is a popular JavaScript library used for making HTTP requests. It provides a simple and convenient interface for sending asynchronous HTTP requests to REST APIs from both web browsers and Node.js applications.
Key Points:
- It is promise-based and supports async and await.
- It has built-in features like intercepting request and response, timeout, and more.
- It can be installed via npm using the following command:
npm install axios
.
Code Example
import React, { useState, useEffect } from 'react';
import axios from 'axios';
function App() {
const [data, setData] = useState(null);
useEffect(() => {
const fetchData = async () => {
const result = await axios('<https://jsonplaceholder.typicode.com/posts>');
setData(result.data);
}
fetchData();
}, []);
return (
<div>
{data && data.map((post) => (
<div key={post.id}>
<h1>{post.title}</h1>
<p>{post.body}</p>
</div>
))}
</div>
);
}
export default App;
In the above example, we're using axios
to fetch data from a REST API and display the data on our component. We're using axios
inside the useEffect
hook to ensure the HTTP request is made after the component mounts.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version