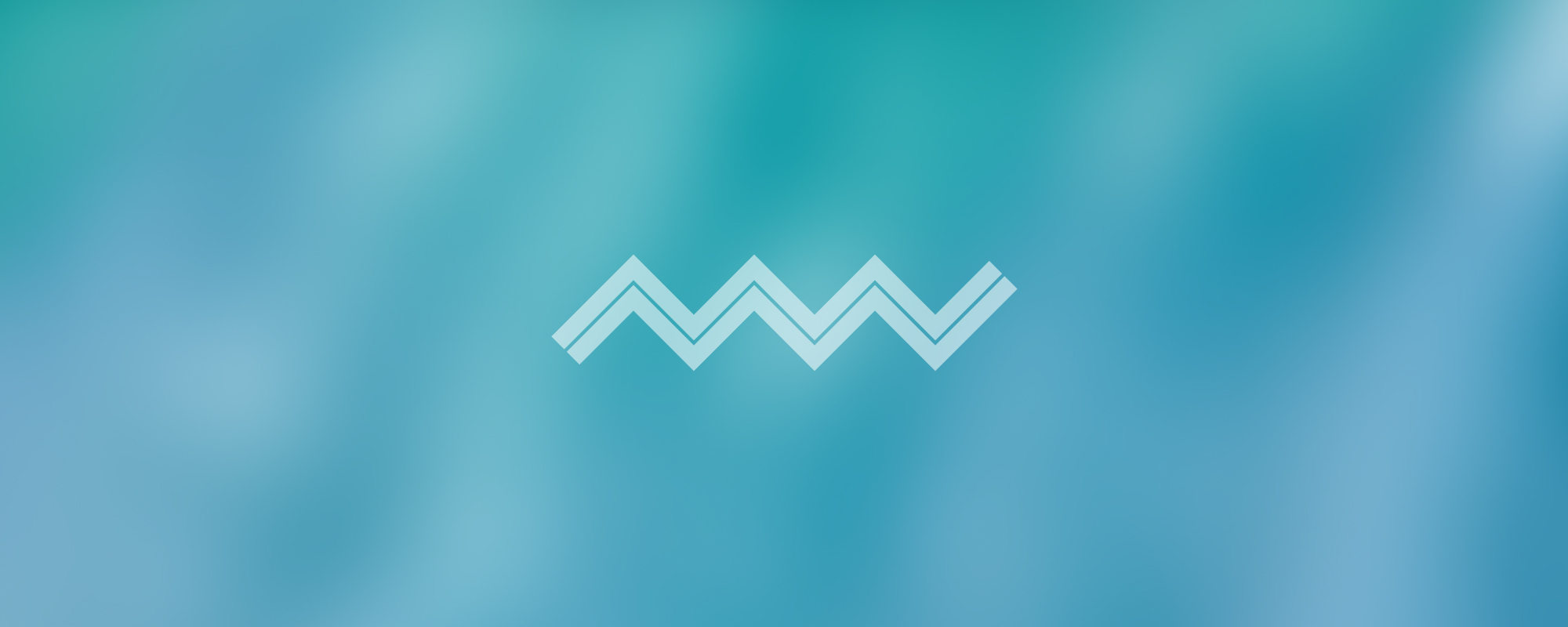
12. Class Components
Class Components in React
Overview
In React, components are the building blocks of your application. They can be created using two primary syntaxes: Class Components and Functional Components. Both types of components serve the same fundamental purpose, which is to define reusable UI elements. However, they have some differences and similarities:
Differences:
Syntax
- Class Components: These are defined as ES6 classes that extend the
React.Component
class. They use arender
method to define the component's output.
- Functional Components: These are regular JavaScript functions that take in
props
as their argument and return JSX to describe the UI.
State
- Class Components: These components can maintain local state using the
state
property. The state can be updated usingthis.setState
method.
- Functional Components: Initially, functional components did not have state. However, since React 16.8, with the introduction of hooks (like
useState
), state can now be used in functional components.
Lifecycle Methods
- Class Components: These components have access to lifecycle methods like
componentDidMount
,componentDidUpdate
, andcomponentWillUnmount
. These methods allow you to perform actions at different stages of a component's life.
- Functional Components: Functional components can achieve similar functionality using React hooks like
useEffect
.
Similarities:
- Props: Both class and functional components can accept and use props (short for properties) to receive data from their parent component. Props are read-only and help in passing data from parent to child components.
- Render Output: Both types of components are responsible for rendering the UI. They return JSX that describes what should be displayed on the screen.
- Reusability: Both class and functional components are reusable. You can use them to build complex UIs by composing smaller components together.
- Context API: Both types of components can access and consume data from the React Context API, which allows you to share data across your component tree without having to pass props explicitly.
- Event Handling: Both types of components can handle events (e.g., click events) and respond to user interactions.
- Children: Both types can have children components nested within them and can render these children components.
Considerations:
Since the introduction of hooks, functional components have become the preferred way to write components in React due to their simplicity and the ability to use state and lifecycle features. However, class components are still widely used in existing codebases and can be useful in certain situations, especially when dealing with legacy code.
If you are starting a new React project or working on a codebase using React 16.8 or later, it's recommended to use functional components with hooks for their simplicity and the direction in which React development is heading.
Class Component Constructor
The constructor in a class component has two main purposes:
- To initialize state.
- To bind functions to the class component.
Example:
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
// Bind function to class component
this.handleIncreaseCounterClick = this.handleIncreaseCounterClick.bind(this);
}
handleIncreaseCounterClick() {
this.setState({ count: this.state.count + 1 });
}
render() {
return (
<div>
<button onClick={this.handleIncreaseCounterClick}>
Increase Count
</button>
<p>Count: {this.state.count}</p>
</div>
);
}
}
Class Component Lifecycle Methods
componentDidCatch()
: This method is invoked if some error occurs during the rendering phase of any lifecycle methods or any children component.
componentDidMount()
: This method allows us to execute the React code when the component is already placed in the DOM (Document Object Model).
componentWillUnmount()
: This method is invoked immediately before a component is unmounted and destroyed. This is where you perform any necessary cleanup, such as invalidating timers, canceling network requests, or cleaning up any subscriptions that were created incomponentDidMount()
.
componentDidUpdate()
: This method is invoked immediately after updating occurs. This method is not called for the initial render. Use this as an opportunity to operate on the DOM when the component has been updated. This is also a good place to do network requests as long as you compare the current props to previous props (e.g. a network request may not be necessary if the props have not changed).
Example:
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
// Bind function to class component
this.handleIncreaseCounterClick = this.handleIncreaseCounterClick.bind(this);
}
componentDidMount() {
console.log('Component has been mounted to the DOM');
}
componentDidUpdate() {
console.log('Component has been updated');
}
componentWillUnmount() {
console.log('Component will be unmounted from the DOM');
}
handleIncreaseCounterClick() {
this.setState({ count: this.state.count + 1 });
}
render() {
return (
<div>
<button onClick={this.handleIncreaseCounterClick}>
Increase Count
</button>
<p>Count: {this.state.count}</p>
</div>
);
}
}
For more examples and a practical application of Class Components in React, you can refer to this example on CodeSandbox: codesandbox.io/s/tmtv63?file=/App.js&utm_medium=sandpack.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version