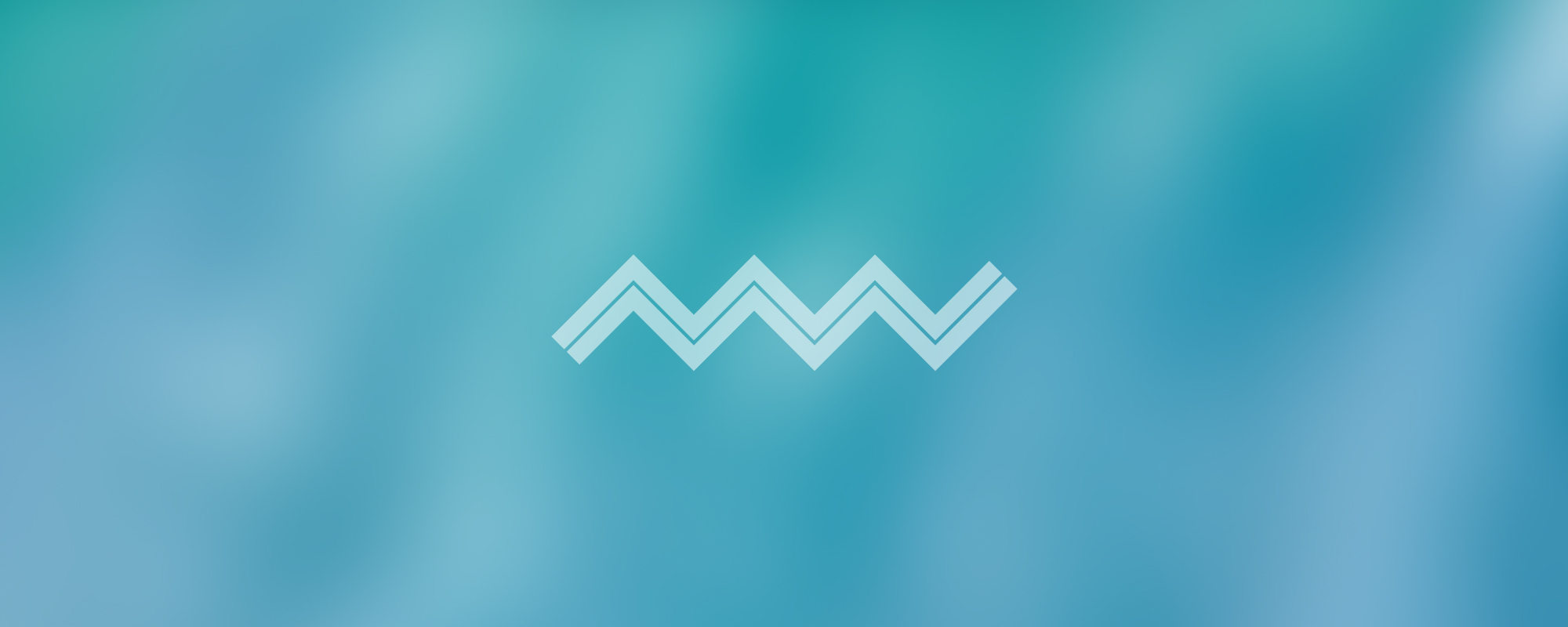
13. Routing
React Routing
Overview
React Routing is a core concept in React applications, providing a way to display different components or pages based on the URL or the route of the application. Essentially, it is a mechanism to navigate between different parts of an application when a user enters a URL or clicks an element (like a link) within the application.
What is Routing?
In the context of a React application, routing is a pattern that provides the user with a way to navigate through the application. It changes the view rendered to the user based on the current URL or the route.
Key Points:
- React Router is the standard routing library for React.
- React Router keeps your UI in sync with the URL.
- It has a simple API with powerful features like lazy code loading, dynamic route matching, and location transition handling built right in.
Introduction to React Router
React Router is a collection of navigational components that compose declaratively with your application. Whether you want to have bookmarkable URLs for your web app or a composable way to navigate in React Native, React Router works wherever React is rendering.
Example:
import React from "react";
import { BrowserRouter as Router, Route, Link } from "react-router-dom";
function App() {
return (
<Router>
<div>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
<li>
<Link to="/topics">Topics</Link>
</li>
</ul>
</nav>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
<Route path="/topics" component={Topics} />
</div>
</Router>
);
}
export default App;
In the above example, we have three navigation links. Clicking each link triggers a route change, rendering a different component depending on the route.
Additional Topics
While the basics of routing are relatively straightforward, there are many advanced topics and techniques that can be used to enhance your React applications. These include nested routing, URL parameters, programmatically navigating, route configuration, and more. These techniques can greatly enhance the usability and user experience of your applications.
For instance, nested routing is a powerful feature that allows you to build more complex UIs, with certain parts of the UI staying the same depending on the route, while other parts change. This is often useful in scenarios where you have a shared layout or structure for multiple routes.
Example of Nested Routing:
import React from 'react';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
function App() {
return (
<Router>
<div>
<Route exact path="/" component={Home} />
<Route path="/topics" component={Topics} />
</div>
</Router>
);
}
function Topics({ match }) {
return (
<div>
<h2>Topics</h2>
<Route path={`${match.path}/:topicId`} component={Topic} />
<Route
exact
path={match.path}
render={() => <h3>Please select a topic.</h3>}
/>
</div>
);
}
export default App;
In this example, the Topics
component is rendering its own Route
components. This means that parts of the Topics
UI can stay the same, while others change depending on the current route, allowing for more complex UI structures.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version