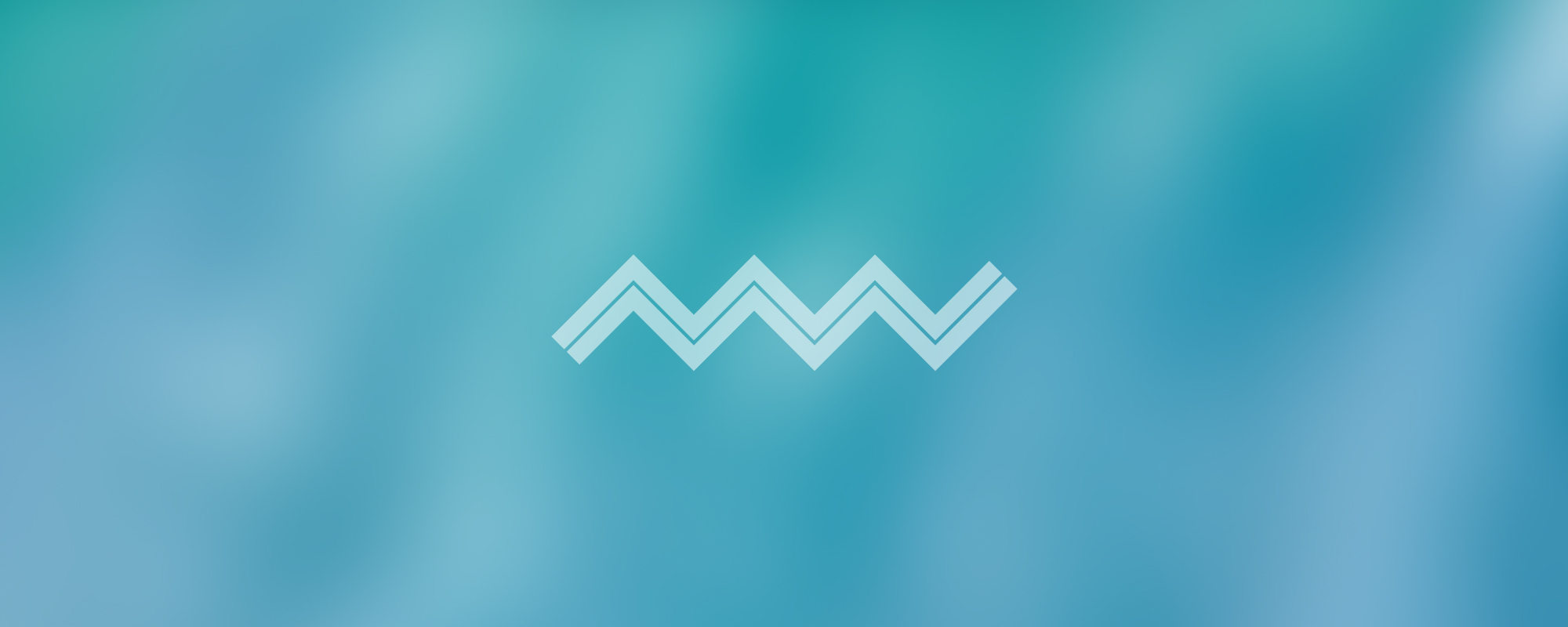
14. Redux Toolkit
Redux Toolkit and React Hooks
Overview of React Hooks
React hooks are functions that allow you to use state and lifecycle methods in functional components, without writing a class component. They were introduced in React 16.8. Here is a list of some commonly used React hooks:
useState
This hook is essential for managing component state. It allows you to add state to functional components.
const [count, setCount] = useState(0);
useEffect
This hook is critical for side effects and lifecycle management. It runs after every render and it's like a combination of componentDidMount
, componentDidUpdate
, and componentWillUnmount
in class components.
useEffect(() => {
document.title = `You clicked ${count} times`;
}, [count]); // Only re-run the effect if count changes
useContext
This hook is important for sharing data across components. It allows you to use context without wrapping a component in a Context.Consumer
.
const ThemeContext = React.createContext('light');
const theme = useContext(ThemeContext);
useReducer
This hook is useful for complex state logic. It's an alternative to useState
and it's ideal for when the next state depends on the previous one.
const [state, dispatch] = useReducer(reducer, initialState);
useRef
This hook is handy for accessing and interacting with DOM elements. It allows you to get a reference to a DOM node or an instance of a component.
const myRef = useRef(null);
useMemo
This hook optimizes expensive computations. It will only recompute the memoized value when one of the dependencies has changed.
const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
useCallback
This hook optimizes callback functions. It's useful when passing callbacks to optimized child components that rely on reference equality to prevent unnecessary renders.
const memoizedCallback = useCallback(
() => {
doSomething(a, b);
},
[a, b],
);
useLayoutEffect
This hook is similar to useEffect
but it runs synchronously. It fires before the useEffect
and before the browser visually updates the page.
useLayoutEffect(() => {
// Your code here
});
useHistory, useParams, useLocation, useRouteMatch
These hooks are part of React Router and allow you to manipulate the browser's history, retrieve parameters from the current route, access the current URL location, and match the current URL against a specific route respectively.
useSelector Hook in Redux Toolkit
useSelector
is a function provided by the React Redux library, which is commonly used in React applications to access and retrieve data from the Redux store.
Redux is a state management library for JavaScript applications, and React Redux is a library that provides integration between Redux and React.
How to use useSelector
Below are the steps to use useSelector
hook in a React application:
- Import necessary dependencies:
Import the
useSelector
function from thereact-redux
library. Also import any actions or selectors that you want to use.import { useSelector } from 'react-redux';
- Create a component:
Define a functional component in your React application. This component will use
useSelector
to access data from the Redux store.function MyComponent() { const myData = useSelector((state) => state.myReducer.myData); return ( <div> <h1>My Data</h1> <p>{myData}</p> </div> ); }
- Configure the Redux store:
Configure the Redux store in your application. This involves creating a store, defining reducers, and setting up the store provider at the top level of your application.
import { createStore, combineReducers } from 'redux'; import { Provider } from 'react-redux'; const initialState = { myData: '' }; function myReducer(state = initialState, action) { switch (action.type) { case 'SET_MY_DATA': return { ...state, myData: action.payload }; default: return state; } } const rootReducer = combineReducers({ myReducer, }); const store = createStore(rootReducer); ReactDOM.render( <Provider store={store}> <App /> </Provider>, document.getElementById('root') );
- Dispatch actions to update state:
To update the state in your Redux store, you'll typically dispatch actions. For instance, if you want to update
myData
, you would dispatch an action like this:dispatch({ type: 'SET_MY_DATA', payload: 'New Data' });
The Redux reducer (
myReducer
in this example) will handle this action and update the state accordingly.useSelector
will automatically re-render your component when the Redux store's state changes, so your component will reflect the updated data.
That's the basic usage of useSelector
in a React Redux application. It allows you to connect your React components to the Redux store, access state, and re-render components when the state changes.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version