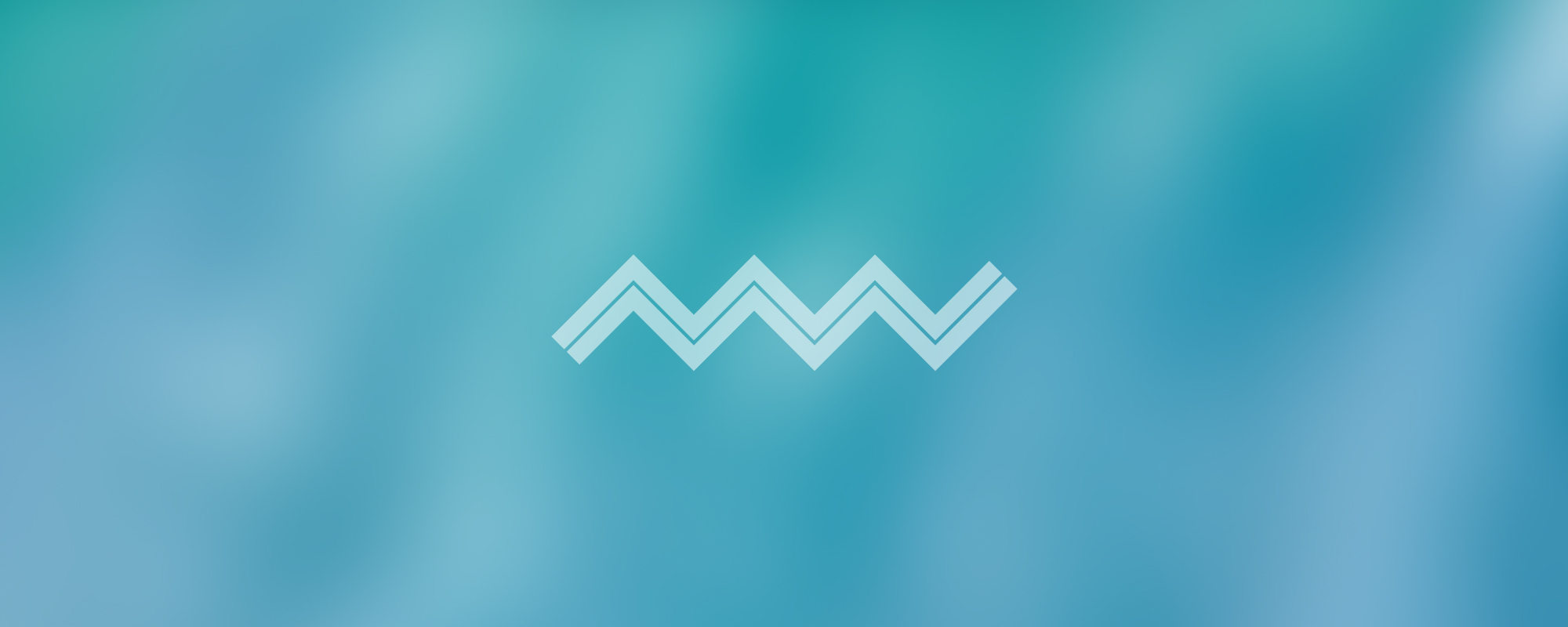
15. Exam Project
React for Exam Project
Introduction to React
React is a declarative, efficient, and flexible JavaScript library for building user interfaces. It allows developers to create large web applications which can change data, without reloading the page. The main purpose of React is to be fast, scalable, and simple. It works only on user interfaces in the application.
Key Points:
- React allows developers to create reusable UI components.
- React uses a virtual DOM which is a lightweight copy of the actual DOM. It allows React to do its computations within this virtual DOM and then updates the web browser's displayed actual DOM, improving performance.
- React follows a unidirectional data flow or data binding.
JSX in React
JSX is a syntax extension for JavaScript. It is used with React to describe what the user interface should look like. By using JSX, we can write HTML structures in the same file that contains JavaScript code.
Example:
const element = <h1>Hello, world!</h1>;
Components in React
In React, Components are the building blocks of any React application and a single app usually consists of multiple components. A component is a JavaScript class or function that accepts inputs (called props
) and returns a React element that describes how a section of the UI should appear.
Example:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
const element = <Welcome name="Sara" />;
ReactDOM.render(
element,
document.getElementById('root')
);
Props in React
Props is short for properties and they are used to pass data from one component to another, as parameters. They are read-only.
Example:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
function App() {
return (
<div>
<Welcome name="Sara" />
<Welcome name="Cahal" />
<Welcome name="Edite" />
</div>
);
}
ReactDOM.render(
<App />,
document.getElementById('root')
);
State in React
State is a built-in object in a component that is used for data that needs to be read, but can also change and be updated over time. It is mostly used with input fields, forms and anywhere you need to update the value dynamically.
Example:
class Clock extends React.Component {
constructor(props) {
super(props);
this.state = {date: new Date()};
}
render() {
return (
<div>
<h1>Hello, world!</h1>
<h2>It is {this.state.date.toLocaleTimeString()}.</h2>
</div>
);
}
}
Conclusion
React is a powerful library for building interactive user interfaces. By understanding the key concepts like JSX, Components, Props, and State, one can effectively build robust web applications for their projects.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version