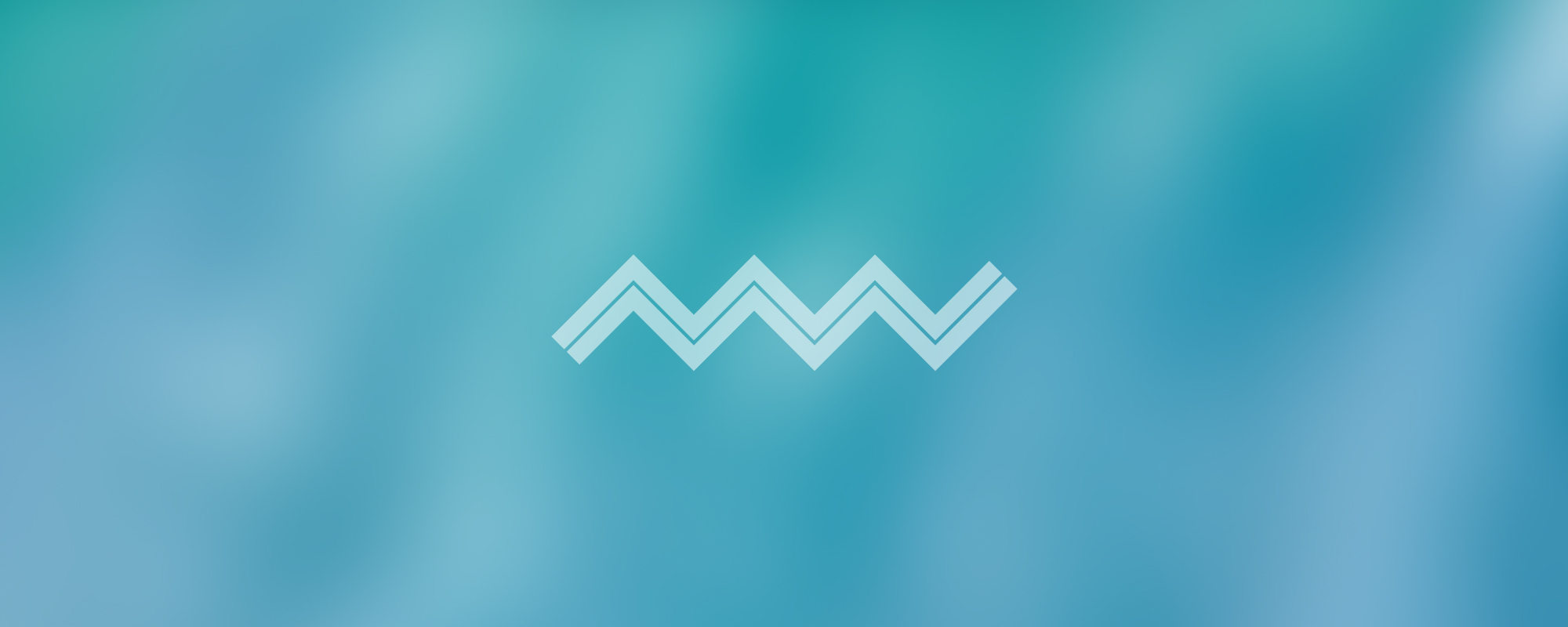
16. Handle Exception
Handling Exceptions in React
Introduction
In React, handling exceptions is a crucial part of developing resilient applications. JavaScript errors inside components can cause the entire React application to crash, which is a poor user experience. Thus, to prevent this, React introduces Error Boundaries.
What are Error Boundaries?
An Error Boundary is a React component that catches JavaScript errors anywhere in their child component tree, logs those errors, and displays a fallback UI instead of the component tree that crashed.
Key Points:
- Error Boundaries catch errors during rendering, in lifecycle methods, and constructors of the whole tree below them.
- Error Boundaries do not catch errors for:
- Event handlers
- Asynchronous code (e.g., setTimeout or requestAnimationFrame callbacks)
- Server-side rendering
- Errors thrown in the error boundary itself
Creating an Error Boundary
To create an Error Boundary, you need a class component with two methods: static getDerivedStateFromError()
and componentDidCatch()
.
Example:
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
// Update state so the next render will show the fallback UI.
return { hasError: true };
}
componentDidCatch(error, info) {
// You can also log the error to an error reporting service
logErrorToMyService(error, info);
}
render() {
if (this.state.hasError) {
// You can render any custom fallback UI
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
Using an Error Boundary
To utilize the Error Boundary, you can use it as a regular component to wrap around potentially error-prone components.
Example:
<ErrorBoundary>
<MyComponent />
</ErrorBoundary>
Handling Uncaught Exceptions
There are cases where exceptions may occur outside of the React component tree (e.g., in event handlers or asynchronous code). These are not caught by Error Boundaries. For these situations, you can use traditional JavaScript error handling techniques such as try-catch
statements.
Example:
try {
// potentially error-prone code
} catch (error) {
console.error(error);
// handle the error
}
By understanding and properly implementing exception handling in React, you can create more robust applications that can withstand and recover from unexpected failures.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version