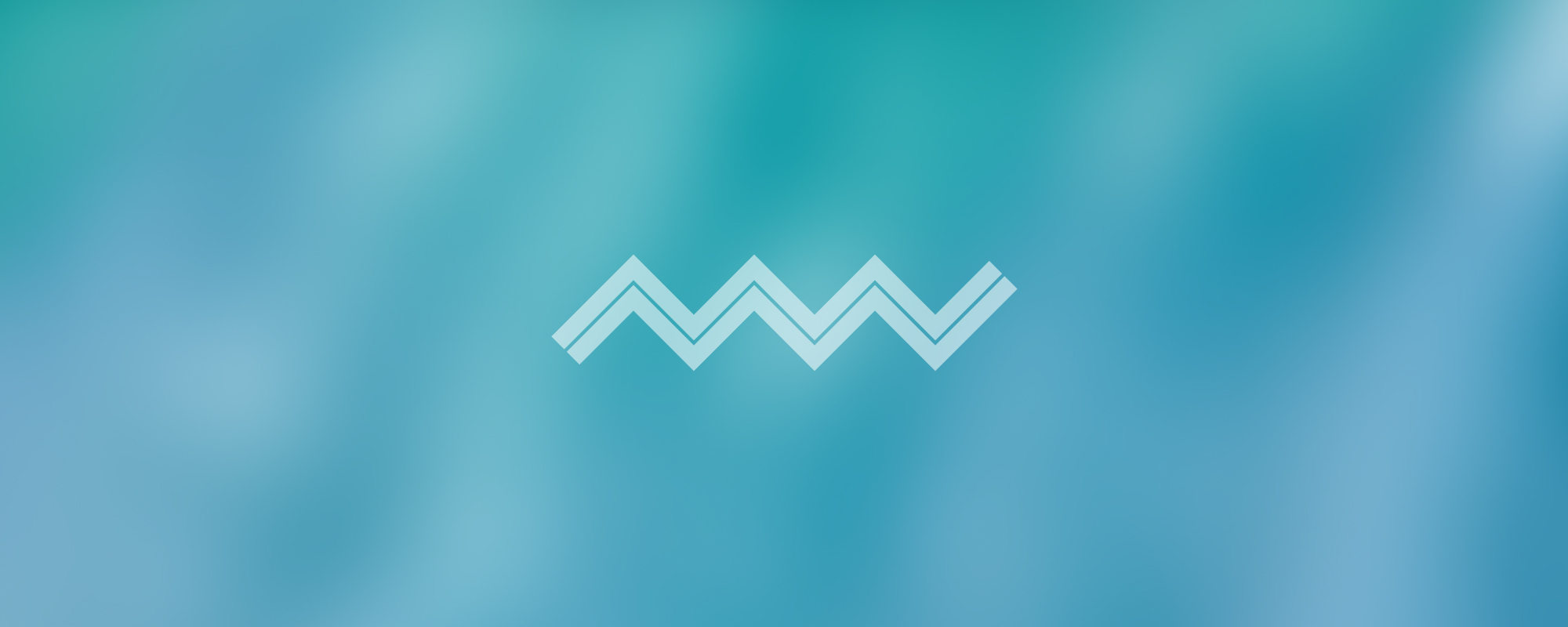
3. Conditional Rendering, Components
Conditional Rendering and Components in React
Introduction
In React, conditional rendering refers to the process of deciding which components to render based on certain conditions. It is roughly equivalent to using if
or conditional
statements in JavaScript.
Understanding Conditional Rendering
Conditional rendering in React works the same way conditions work in JavaScript. Use JavaScript operators like if
or the conditional operator to create elements representing the current state, and let React update the UI to match them.
Code Example
Consider the following example where we use conditional rendering to decide whether to show a packed item with an icon or without an icon.
import React from 'react'
function Item({name, isPacked}){
if (isPacked){
return <li className="item">{name}
<img className='icon'
src='<https://static.vecteezy.com/system/resources/previews/009/362/738/original/tick-icon-accept-approve-sign-design-free-png.png>'
alt='packed-item-icon'></img></li>
}
return <li className="item">{name}</li>
}
export default function PackingList() {
return (
<section>
<h1>
Aykhan's Package List
</h1>
<ul>
<Item isPacked={true} name="Space Suit"></Item>
<Item isPacked={false} name="Helmet with a Golden Leaf"></Item>
<Item isPacked={false} name="Photo of Child"></Item>
</ul>
</section>
)
}
In the above code example, we have a PackingList
component that renders an Item
component for each item in the package list. The Item
component uses a conditional if
statement to decide whether to render the item with a packed-icon or without an icon.
Exporting in JavaScript
The export
statement is used when creating JavaScript modules to export functions, objects, or primitive values from the module so they can be used by other programs with the import
statement.
Consider the following example:
export const people = [
{
id: 1,
name: "Aykhan Ahmadzada",
profession: "Programming",
imageId: 1,
},
{
id: 2,
name: "Javid Ahmad",
profession: "Cyber Security",
imageId: 2,
}
];
In the above example, we export an array of objects people
so that it can be imported and used in other JavaScript files.
Pure Functions
A pure function is a type of function in computer programming that produces the same output for the same input, and it does not have any side effects on the program or the outside world. In other words, a pure function is solely determined by its input, and it doesn't modify any external state or variables.
Here's an example of a pure function:
function square(x) {
return x * x;
}
In the above example, the function square
is a pure function because it always returns the same result given the same input and it does not modify any external state or variables.
Summary
In summary, React offers a powerful way to handle conditional rendering which is a crucial aspect in creating dynamic web applications. Coupled with the understanding of how to properly use components, export data and pure functions, you are now equipped with the knowledge to design and structure your React applications in a more efficient and effective manner.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version