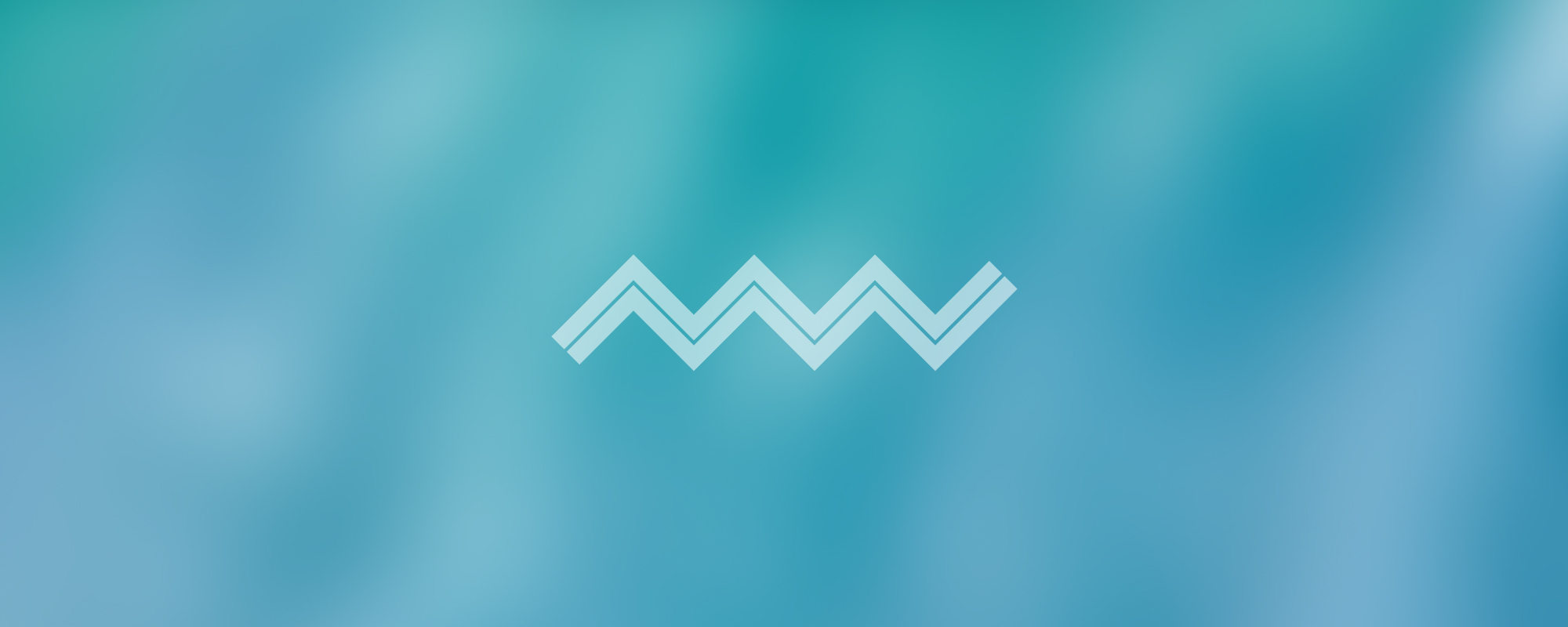
5. To Do List, Practice with React Elements
To Do List: Practice with React Elements
Introduction to React Elements
React Elements are the smallest building blocks of a React app. They are objects that describe what you want to see on the screen. They are immutable and don't have their state.
Key Points:
- React elements are immutable.
- They do not have their own state.
- React elements can be easily created with JSX syntax.
Creating React Elements
React elements are created using React.createElement()
. This method takes three arguments: the type of element, any attributes it has, and its children.
Example:
const element = React.createElement(
'h1',
{className: 'greeting'},
'Hello, world!'
);
However, most React developers prefer to write these elements in JSX. JSX is a syntax extension for JavaScript that looks similar to HTML. Babel compiles JSX down to React.createElement()
calls.
Example:
const element = <h1 className="greeting">Hello, world!</h1>;
Rendering React Elements
React elements can be rendered to the DOM using ReactDOM.render()
. The ReactDOM.render()
method takes two arguments: a React element, and a DOM node where the element will be rendered.
Example:
ReactDOM.render(
element,
document.getElementById('root')
);
To Do List Application using React Elements
Let's create a simple To-Do List application using React elements. We will represent each to-do item as a React element.
const todoItems = ['Item 1', 'Item 2', 'Item 3'];
const element = (
<div>
<h1>Todo List</h1>
<ul>
{todoItems.map((item, index) =>
<li key={index}>{item}</li>
)}
</ul>
</div>
);
ReactDOM.render(
element,
document.getElementById('root')
);
In the above example, each to-do item is represented as a <li>
element. The map()
function is used to create an array of <li>
elements. Each element is given a unique key
prop to help React identify which items have changed, are added, or are removed.
Remember, practicing with React elements is key to understanding how React works and building larger, more complex applications. So keep practicing and keep building!
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version