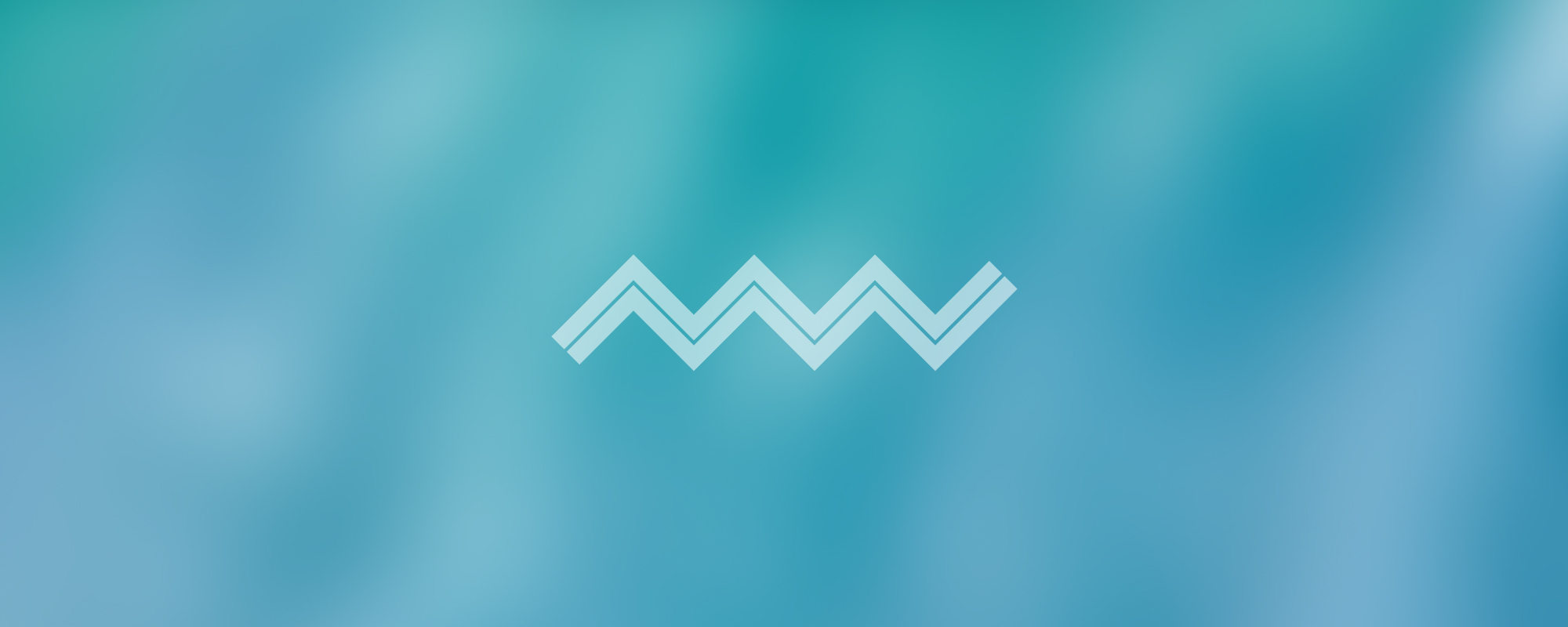
6. Work with Arrays, Hooks
Working with Arrays and Hooks in React
Introduction to Arrays in React
In React, an array is a data structure that can store multiple elements of the same type. It is often used in combination with the map() function to dynamically generate a list of components.
Key Points:
- An array can store multiple values in a single variable.
- Array elements can be accessed by their index number.
Use of Arrays in React
Arrays in React are primarily used for list rendering. List Rendering is a technique in React that allows you to display a list of similar items in a component.
Example:
const names = ['John', 'Paul', 'George', 'Ringo'];
function App() {
return (
<div>
{names.map(name => <p>{name}</p>)}
</div>
);
}
export default App;
Introduction to Hooks in React
React Hooks are functions that let you hook into React state and lifecycle features from function components. They were introduced in React version 16.8 to enable stateful logic in function components, which were previously stateless.
Key Points:
- Hooks allow you to use state and other React features without writing a class.
- The most frequently used hooks are
useState
anduseEffect
.
Use of Hooks in React
Hooks provide a way to handle stateful logic in functional components. The useState
hook lets you add state to functional components, while the useEffect
hook allows you to perform side effects, like data fetching or manual DOM manipulation.
Example:
import React, { useState, useEffect } from 'react';
function App() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `Count: ${count}`;
}, [count]);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default App;
Conclusion
Working with arrays and hooks in React is essential for creating dynamic and interactive user interfaces. Understanding how to use these concepts effectively will greatly enhance your ability to build complex applications with React.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version