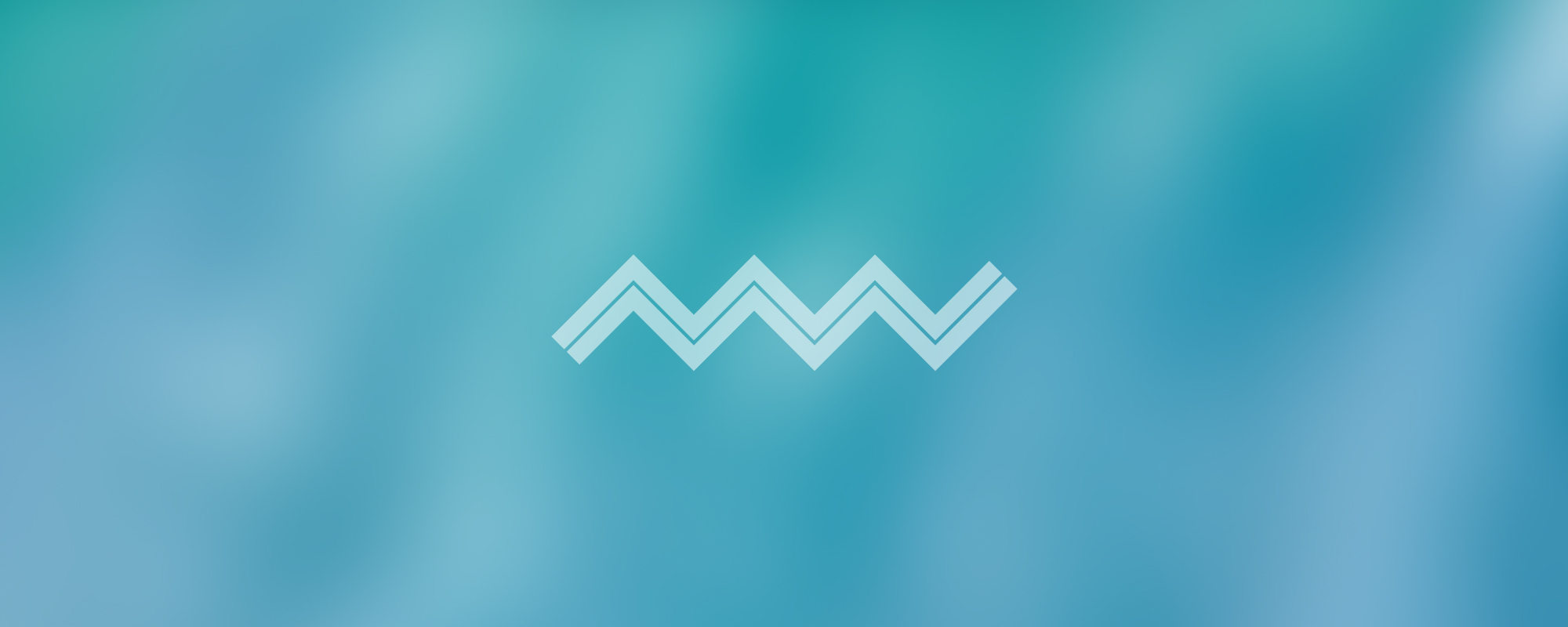
8. Form Validations
Form Validations in React using React Hook Form
Installation of React Hook Form
React Hook Form is a lightweight and efficient package that simplifies form validation in React applications. To install react-hook-form
, navigate to your project's root folder in the terminal or command prompt and execute the following command:
npm install react-hook-form
This command downloads and installs the react-hook-form
package along with its necessary dependencies into your project's node_modules
folder.
Importing and Usage in React Components
Once installed, react-hook-form
can be imported into your React components. Place the import statement at the beginning of your file as shown:
import { useForm } from 'react-hook-form';
With react-hook-form
, form state management, validation and submission in React applications are made simpler and more efficient.
Form Validation with React Hook Form
Form validation is a vital part of any application that requires input from users. It ensures that the data received is as expected. React Hook Form provides a variety of methods for form validation.
Basic Usage
Here is a basic example of how to use the useForm
hook to manage form state and validate user input:
import React from 'react';
import { useForm } from 'react-hook-form';
function App() {
const { register, handleSubmit, errors } = useForm();
const onSubmit = data => console.log(data);
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input name="firstName" ref={register({ required: true })} />
{errors.firstName && 'First name is required'}
<input name="age" ref={register({ pattern: /\\d+/ })} />
{errors.age && 'Age must be a number'}
<input type="submit" />
</form>
);
}
export default App;
In this example, the useForm
hook returns an object with methods and properties that you can use to manage form state and validation. The register
function is used to register input fields with the form so that they can be validated.
The handleSubmit
function is used to handle form submission. It receives a callback function which is called with the form data when the form is valid.
The errors
object contains any validation errors for the registered fields.
For more information on how to use React Hook Form
, refer to the official documentation: React Hook Form Documentation.
Additional Topics
- Advanced validation scenarios with React Hook Form
- Performance optimization with React Hook Form
- Integration of React Hook Form with other UI libraries
- Using React Hook Form with TypeScript
- Form state management with React Hook Form
- Custom validation rules with React Hook Form
- Error handling with React Hook Form
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version