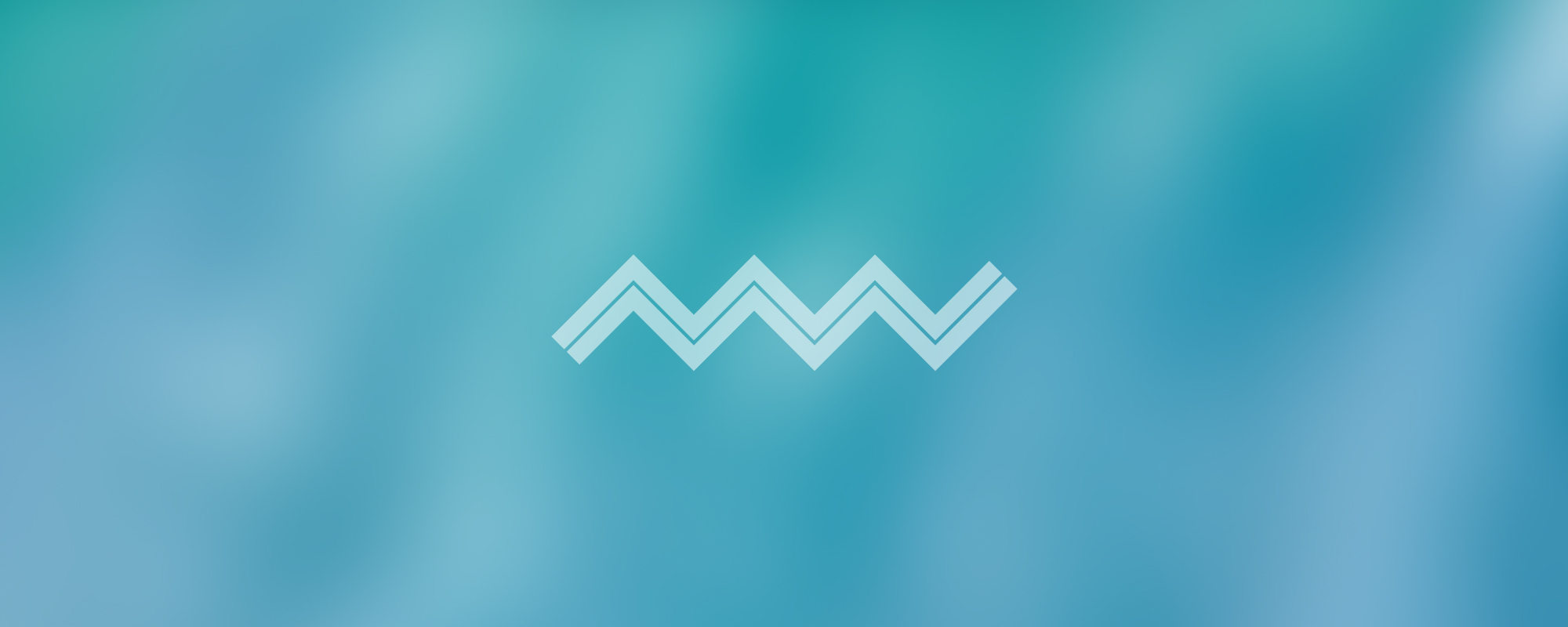
9. Fragments, Profiler
Fragments, Profiler in React
UseRef
UseRef is a hook provided by React that allows us to preserve data across the entire lifespan of a component without triggering a re-render. This differs from useState which does the same thing but causes the component to re-render.
Fragment Tag
The Fragment tag in React allows us to group multiple elements together without adding unnecessary nodes to the DOM. This is useful when you want to return multiple elements from a component's render method.
When we use a regular HTML tag, like div
, the tag is added to the DOM. However, when we use a Fragment, no additional nodes are added to the DOM.
Example
import React, { Fragment } from 'react'
export default function MyList() {
return (
<Fragment>
<Fragment>
<li>Watermelon</li>
<li>Apple</li>
<li>Mango</li>
</Fragment>
<Fragment>
<li>Mercedes</li>
<li>BMW</li>
<li>Kia</li>
</Fragment>
</Fragment >
)
}
In the above example, instead of using div
, we used Fragment
. This prevents the addition of an extra node (the container of MyList
, which would be a div
). Only the li
elements are added.
The shorthand for Fragment
is <>...</>
. We can rewrite the above example as follows:
<>
<>
<li>Watermelon</li>
<li>Apple</li>
<li>Mango</li>
</>
<>
<li>Mercedes</li>
<li>BMW</li>
<li>Kia</li>
</>
</>
Profiler
Profiler is a tool provided by React for examining the performance of a React application. It can be used to identify parts of a React application that are slow and may benefit from optimizations such as memoization.
Here is an example of a skeleton Profiler usage:
import {Profiler} from 'react';
function App() {
return (
<Profiler id="MyApp" onRender={callback}>
<div>
{/* <Counter></Counter> */}
{/* <MyList></MyList> */}
{/* <Blog></Blog> */}
</div>
</Profiler>
);
}
Profiler is used during the development phase and not included in the production build. This is because profiling can be an expensive operation and may slow down your application.
createPortal
createPortal is a method provided by React that allows you to render children into a different part of the DOM. This is useful for cases like modals, where the modal component needs to be a child of the application component for React context purposes, but needs to be a child of the body DOM node for stacking and positioning purposes.
Please note that the above descriptions are general overviews of the concepts. To fully understand and utilize these features, you should refer to the official React documentation.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version