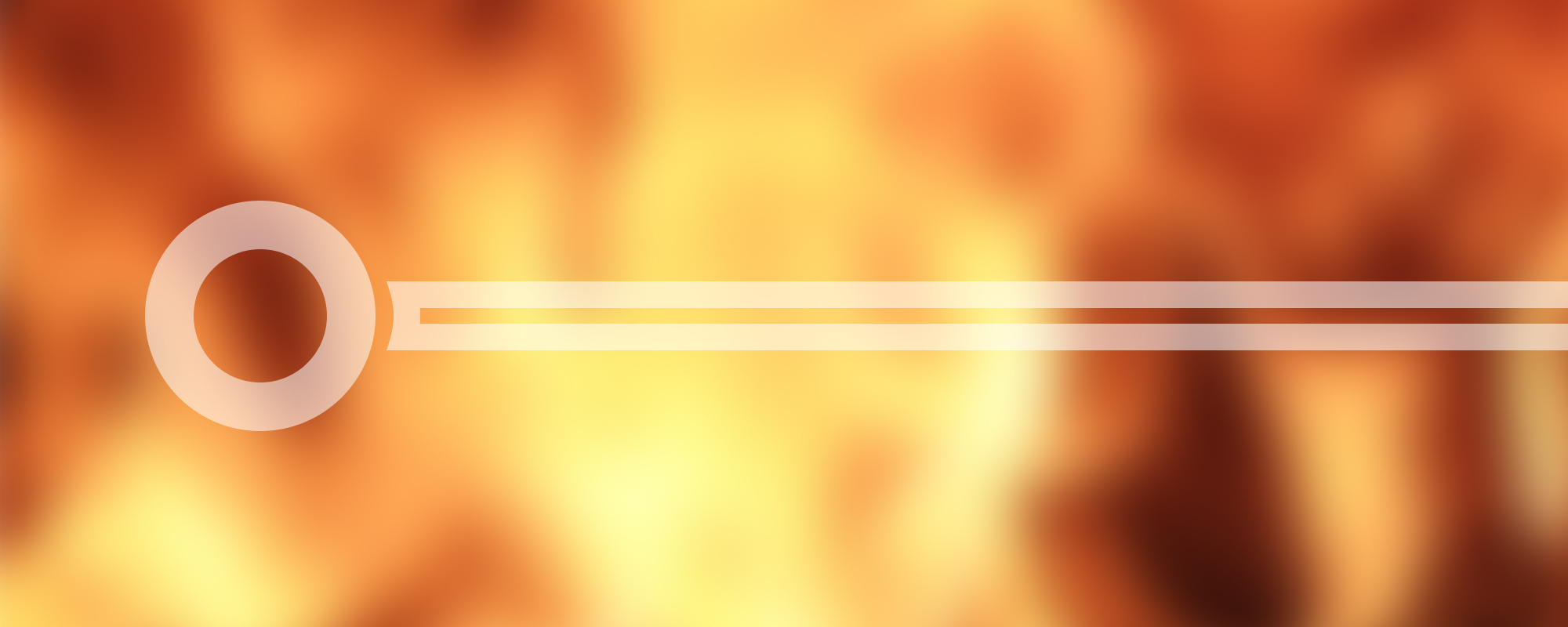
2. Thread
App Domains and Threads in C#
App Domain
Definition
An App Domain in C# is a mechanism that isolates executed applications from each other. This isolation helps in achieving robust security and a stable environment for applications.
Uses of App Domain
- Resource Management: App Domains help in discarding unwanted processed packages, effectively managing system resources.
- Security: If we're unsure about third-party elements (e.g., what they will do, possibly tampering with disk content), App Domains provide a layer of protection.
Key Points
- To get the domain of a program, use:
string path = AppDomain.CurrentDomain.SetupInformation.ApplicationBase;
- It's essential to Unload the domain at the end:
AppDomain.Unload(domain);
Threads
Definition
A Thread is a separate path of execution in a program. It allows for concurrent operations, enhancing the program's efficiency.
ThreadStart
ThreadStart is a delegate used to define the methods that the thread will execute upon starting.
Example:
ThreadStart threadStart = new ThreadStart(Something);
Thread t = new Thread(threadStart);
t.Start();
Alternatively, you can create a thread without explicitly using ThreadStart as follows:
Thread t = new Thread(() =>
{
Something();
});
t.Start();
Or simply:
Thread th = new Thread(Something);
th.Start();
Background and Foreground Threads
Definition
Threads in .NET can be classified as either Foreground or Background threads.
- Foreground Threads: These are independent threads. The application will not terminate until all foreground threads finish execution.
- Background Threads: These are dependent on foreground threads. They are automatically stopped when all foreground threads are completed.
Example
When we enter a game, and it starts to load (this is a background thread). If we force close the game during loading (foreground thread stops), the game loading thread (background thread) also stops.
To set a thread as a background thread:
thread.IsBackground= true;
Thread.Join()
Thread.Join() is a method that blocks the calling thread until a thread terminates. This can be useful when one thread depends on the result of another thread.
Example:
Thread t = new Thread(Something);
t.Start();
t.Join(); // This will block the calling thread until `t` finishes execution
AppDomain Hierarchy
An AppDomain can have multiple threads, and each thread can have multiple tasks. This structure forms the AppDomain hierarchy. This hierarchy helps manage application control flow and resource allocation effectively.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version