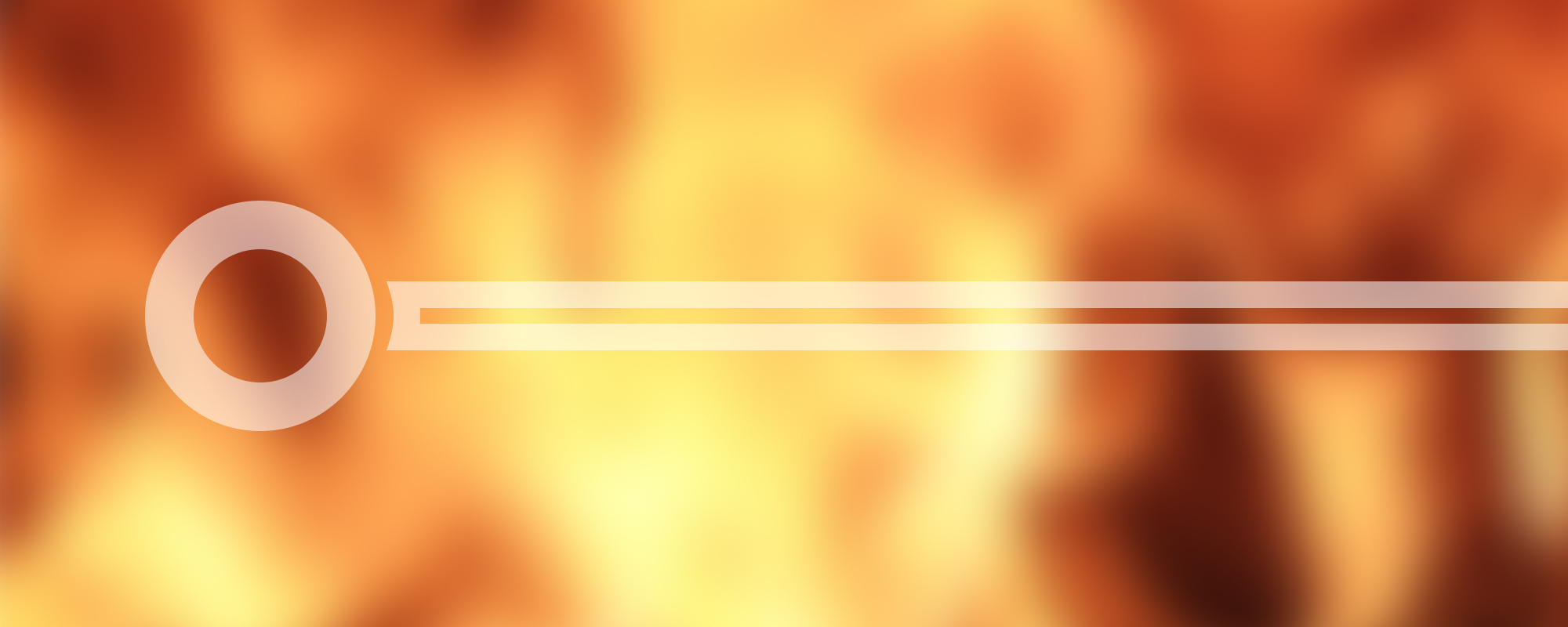
3. ThreadPool
ThreadPool in C#
What is ThreadPool?
A ThreadPool is a collection of threads that can be used to post work items, process asynchronous I/O, wait on behalf of other threads, and process timers. It is a way to reuse threads, as thread creation and destruction can be costly in terms of system resources.
In a typical system, multiple users may make requests simultaneously. For example, 5-6 users may arrive in a second, each creating a new thread. Each time a thread is created, 1MB of space in RAM is allocated, which can lead to memory and time issues.
However, with a ThreadPool, we have roughly 2000 threads (1000 active, 1000 passive). When a thread is needed, instead of creating a new one, we take one from the pool. This approach avoids potential memory problems.
When to use ThreadPool
- For short-term tasks, we allocate a thread from the ThreadPool.
- For long-term tasks, we create a new thread.
ThreadPool and Delegates
In C#, a delegate is a reference type variable that holds the reference to a method. A delegate is especially useful when you want to pass a method as a parameter.
In the context of ThreadPool, the .Invoke()
method of a delegate calls the method in the same thread. However, the .BeginInvoke()
method calls the method in a new thread.
Example:
public delegate void SimpleDelegate(string param);
public class Program
{
public static void MyFunc(string param)
{
Console.WriteLine("I was called by delegate ...");
Console.WriteLine("I got parameter {0}", param);
}
static void Main(string[] args)
{
SimpleDelegate simpleDelegate = new SimpleDelegate(MyFunc);
// This will be called in the same thread
simpleDelegate.Invoke("Invoke");
// This will be called in a new thread from ThreadPool
simpleDelegate.BeginInvoke("BeginInvoke", null, null);
}
}
In the above example, MyFunc
is called twice - once with Invoke
and once with BeginInvoke
. The Invoke
call executes the method in the same thread as the Main
method, while the BeginInvoke
call executes the method in a new thread from the ThreadPool.
In conclusion, the ThreadPool is a crucial component in managing system resources efficiently and effectively in multithreaded programming, by reusing threads instead of creating and destroying them for each task. It is important to understand its usage and the implications of using ThreadPool threads versus creating new threads.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version