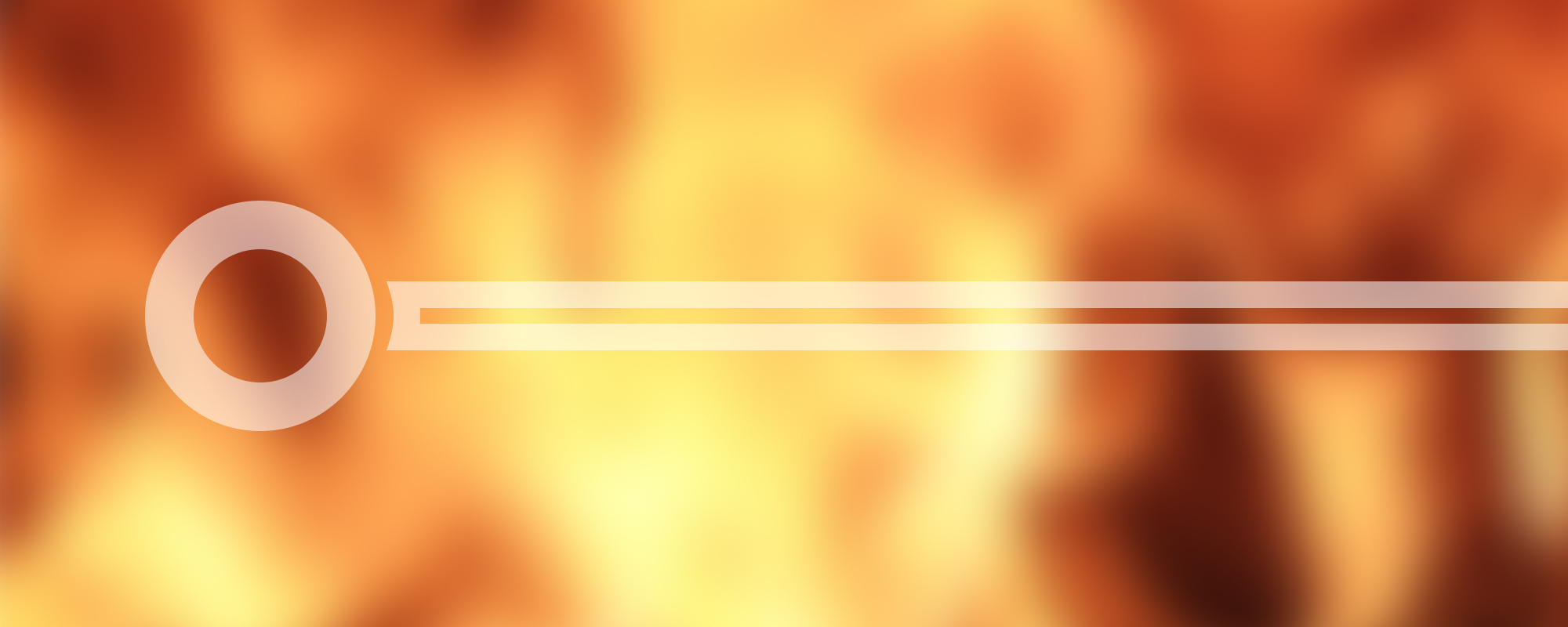
5. Semaphore
Semaphore in Multi-threading
Introduction to Semaphore
A semaphore is a high-level synchronization primitive that controls access to shared resources by multiple processes in a concurrent system. Unlike Mutex (Mutual exclusion) which allows only one thread to access a shared resource at a time, a semaphore can allow one or more threads to access shared resources simultaneously.
Key Points:
- A semaphore maintains a count of permits. If a thread wants to access a shared resource, it must first acquire a permit from the semaphore.
- If the semaphore's count of permits is zero, the thread will be blocked until a permit is available.
- When a thread has finished using the resource, it must release the permit back to the semaphore.
Types of Semaphore
There are two types of semaphores:
1. Semaphore Slim
Semaphore Slim is an application-level semaphore, which is lighter and faster than a system-level semaphore. It is suitable for scenarios where a large number of threads need to access shared resources concurrently.
2. Semaphore (System Level)
System-level semaphore is a semaphore that can be used across multiple processes. It is suitable for inter-process communication and synchronization.
Examples of Semaphore Usage
Here is an example of how to use a semaphore in C#:
using System;
using System.Threading;
public class Program {
private static Semaphore _pool;
static void Main() {
_pool = new Semaphore(0, 3);
for(int i = 1; i <= 5; i++) {
Thread t = new Thread(new ParameterizedThreadStart(Worker));
t.Start(i);
}
_pool.Release(3);
Console.ReadLine();
}
static void Worker(object num) {
_pool.WaitOne();
Console.WriteLine("Thread {0} begins and waits for the semaphore.", num);
Thread.Sleep(1000 * (int)num);
Console.WriteLine("Thread {0} releases the semaphore.", num);
Console.WriteLine("Previous semaphore count: {0}", _pool.Release());
}
}
In this code, a Semaphore named _pool is created with an initial count of zero and a maximum count of three. Five threads are created, each calling the Worker method. The Worker method waits for a permit from the semaphore before it can execute. When the Worker method is done, it releases the semaphore, making the permit available to the next thread. The semaphore's count of permits is increased by three using the _pool.Release(3) method, allowing three threads to enter the semaphore.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version