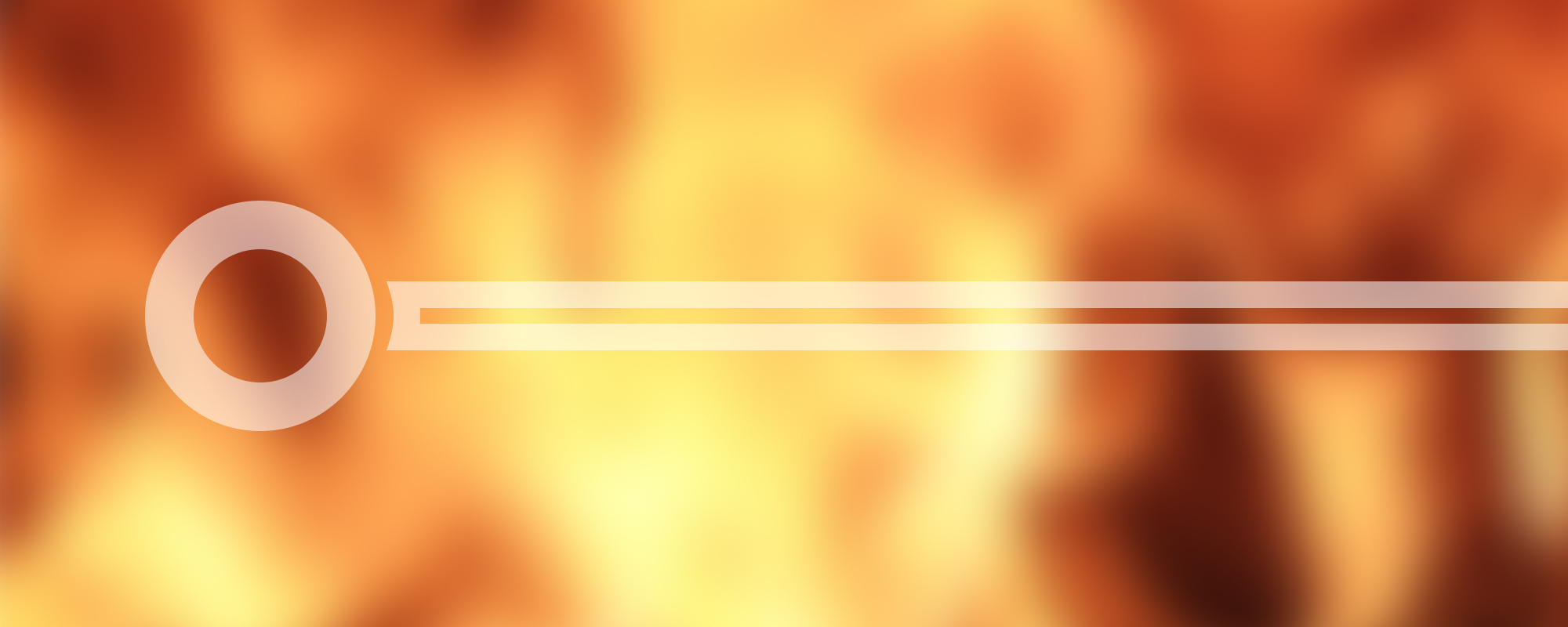
6. Task, Auto Reset Event
Task and AutoResetEvent in C#
Introduction
The C# language provides various ways to handle multithreaded programming, two of which include the Task
class and the AutoResetEvent
class.
AutoResetEvent
The AutoResetEvent
class in C# is a signalling mechanism. It plays a vital role in thread synchronization, allowing one thread to unblock another. This class is found within the System.Threading
namespace.
Key Points:
- An
AutoResetEvent
is used to send a signal to a thread that something happened.
- It has two primary states: signaled and non-signaled.
- When in the signaled state, if a thread calls
WaitOne
, the thread continues execution and theAutoResetEvent
returns to the non-signaled state.
- If a thread calls
WaitOne
while theAutoResetEvent
is in the non-signaled state, the thread will block until another thread changes the state to signaled using theSet
method.
Example:
AutoResetEvent autoResetEvent = new AutoResetEvent(false);
Thread thread1 = new Thread(() => {
Console.WriteLine("Thread1 is waiting...");
autoResetEvent.WaitOne();
Console.WriteLine("Thread1 is released...");
});
Thread thread2 = new Thread(() => {
Console.WriteLine("Thread2 is working...");
Thread.Sleep(2000); // simulates work
autoResetEvent.Set();
Console.WriteLine("Thread2 has signaled.");
});
thread1.Start();
thread2.Start();
Task Class
The Task
class represents a single operation that does not return a value and that usually executes asynchronously. The Task
class is a part of the System.Threading.Tasks
namespace.
Key Points:
- The
Task
class leverages the thread pool to execute tasks in the background.
- Unlike a thread, a
Task
can return a result.
- A
Task
can be awaited, meaning it can be paused until the result is ready.
- The
TaskCreationOptions
enumeration provides several options for customizing the behavior of a task.
TaskCreationOptions
TaskCreationOptions
is an enumeration that provides several options to customize the behavior of Task
. The LongRunning
option indicates that the task will take a long time to execute.
Example:
var task = Task.Factory.StartNew(() => TaskMethod("Task 1"), TaskCreationOptions.LongRunning);
Await vs Result
The key difference between await
and Result
is that Result
blocks the current thread until the task is finished executing, while await
does not block the current thread but instead yields control back to the caller until the task is completed.
Example:
public async Task<int> CalculateResult()
{
await Task.Delay(5000); // simulates some calculation
return 5;
}
public async Task TestMethod()
{
Task<int> calculation = CalculateResult();
int result = await calculation; // does not block the thread
Console.WriteLine(result);
}
public void BlockingMethod()
{
Task<int> calculation = CalculateResult();
int result = calculation.Result; // blocks the thread
Console.WriteLine(result);
}
In conclusion, understanding the Task
and AutoResetEvent
classes in C# is crucial for effective multithreaded programming. These classes provide the tools needed to create responsive applications that can handle a multitude of tasks simultaneously.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version