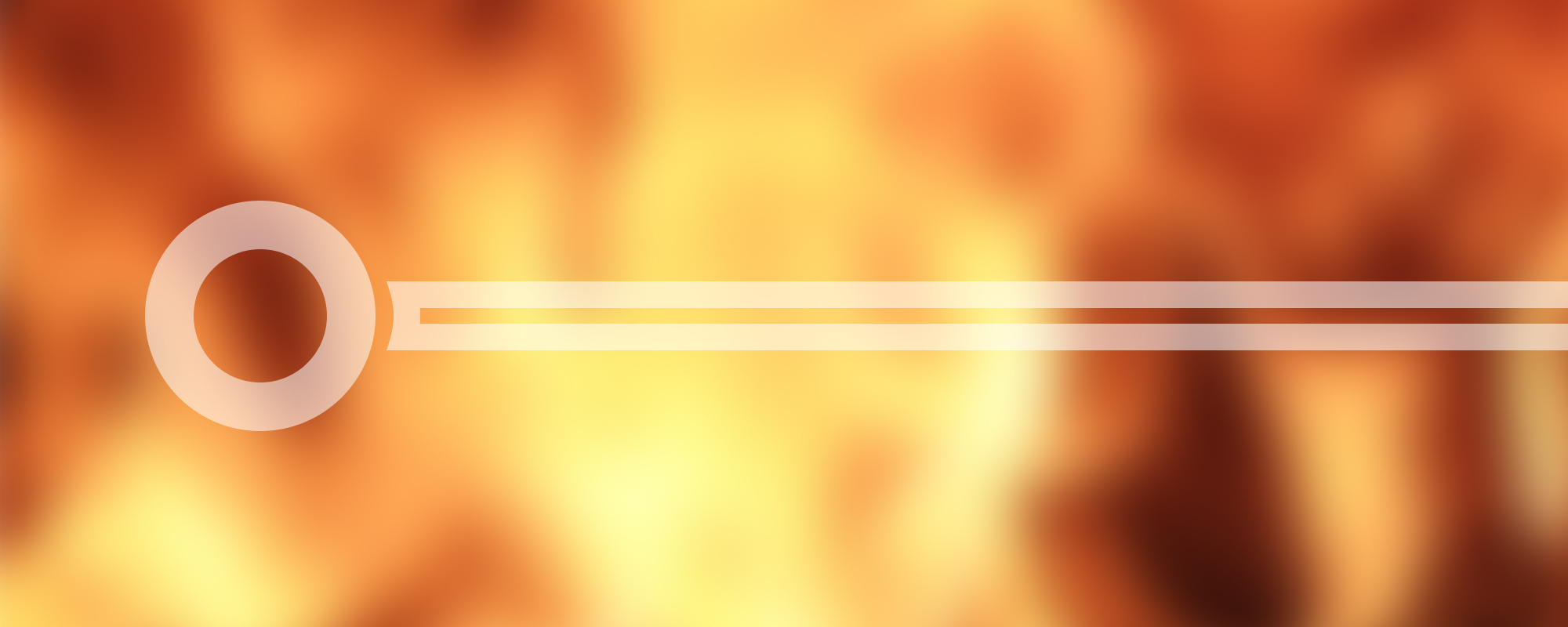
7. Async, Await, Task, API Practise
Async, Await, Task, and API Practice in C#
Introduction to Async and Await
The async and await keywords in C# are used to perform asynchronous operations. Asynchronous programming is a means of writing non-blocking code by running a task in a new thread, and not waiting for it to complete and continue with the rest of the code.
Key Points:
- The async keyword is used to define a method as asynchronous.
- The await keyword is used inside an async method to suspend the execution until the awaited task completes.
Understanding Task in C#
A Task in C# is an object that represents some work that should be done. The Task can tell you if the work is completed and if the operation returns a result, the Task gives you the result.
Key Points:
- A Task can be in one of three states: Running, Waiting, or Completed.
- The Task class comes with static methods like Run and WhenAll for running tasks.
Using Async, Await, and Task Together
By using async, await, and Task together, you can write asynchronous code that is easy to read and understand.
Example:
public async Task<string> FetchDataAsync(string url) {
using (HttpClient client = new HttpClient()) {
string result = await client.GetStringAsync(url);
return result;
}
}
API Practice with Async, Await, and Task
When calling APIs, it's important to use async and await to prevent blocking the main thread.
Example:
public async Task CallAPIAsync(string url) {
HttpClient client = new HttpClient();
string result = await client.GetStringAsync(url);
Console.WriteLine(result);
}
public async Task Main(string[] args) {
await CallAPIAsync("<https://api.github.com/users/dotnet/repos>");
}
In the example above, we are calling GitHub's API to get a list of repositories for the ".NET" user. The GetStringAsync
method is asynchronous and returns a Task. We use the await
keyword to pause the execution of the CallAPIAsync
method until GetStringAsync
completes.
Best Practices
- Always use
async Task
instead ofasync void
for your async methods.
- Use
async
andawait
as much as possible when dealing with I/O-bound operations like calling APIs, reading files or querying databases.
- Avoid using
.Result
or.Wait()
in async methods as they can lead to deadlock.
- Use
Task.Run()
for CPU-bound operations.
Summary
The async and await keywords, along with the Task class, are powerful tools in C# for writing asynchronous code. They allow you to write code that is non-blocking and responsive, which is particularly important when calling APIs or doing other I/O-bound operations.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version