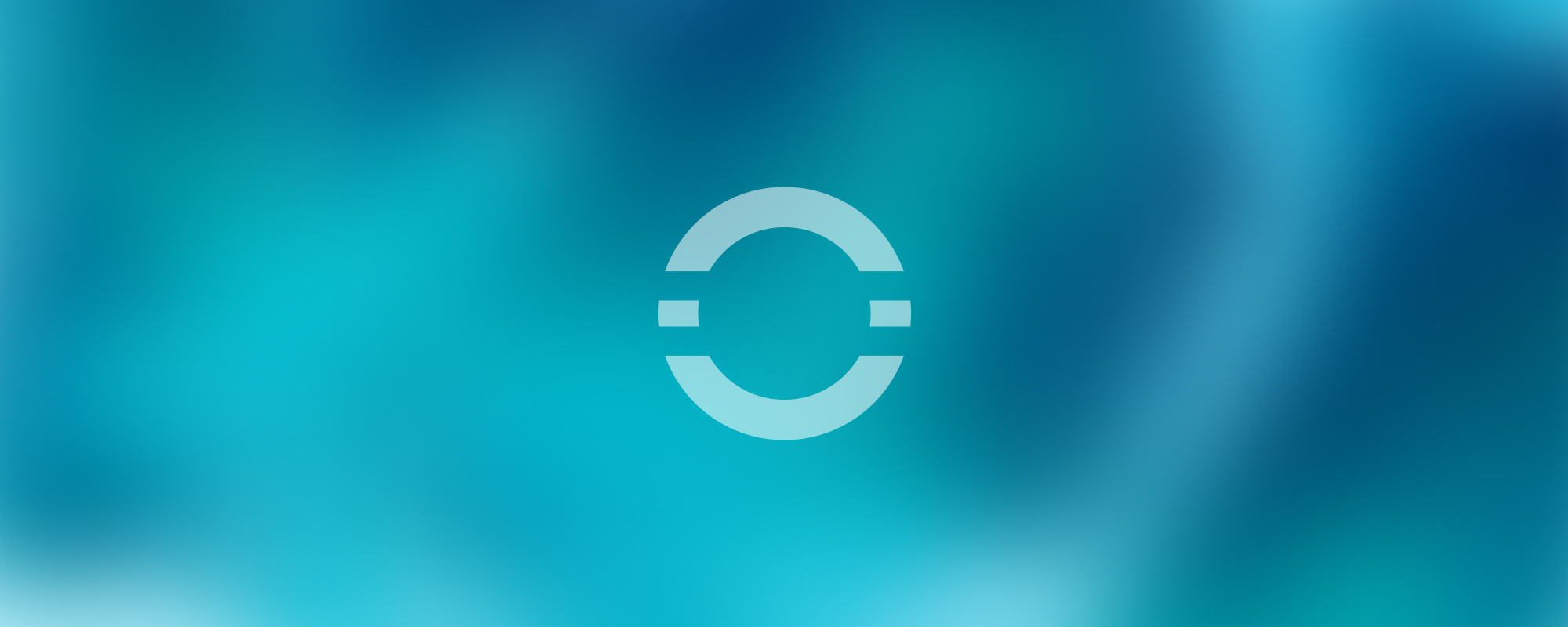
10. SASS, SCSS
SASS and SCSS in Web Programming
Introduction to SASS
SASS (Syntactically Awesome Stylesheets) is a preprocessor scripting language that is interpreted or compiled into Cascading Style Sheets (CSS). It offers a more dynamic approach to styling webpages, enhancing the capabilities of CSS with features such as variables, mixins, and nesting.
Setting Up SASS
Before you can start using SASS, you need to set it up in your development environment. Here are the steps to do that:
- Download the SASS extension for your code editor.
- Download the Live SASS Compiler extension for your code editor.
- Install SASS globally in your command line or terminal with the following command:
npm install -g sass
.
After installation, use the 'Watch SASS' feature to automatically convert your SASS code into CSS code. This creates a style.css.map
file linked to your style.css
file. Any code written in your style.scss
file (that you created) will be linked to the style.css
file.
Note: To run SASS files, Node.js must be installed on your computer.
SASS Syntax
Unlike CSS, SASS allows for nesting of selectors, making your code cleaner and easier to read. The syntax difference is as follows:
CSS Syntax:
nav ul { }
SASS Syntax:
nav {
ul { }
}
Importing Other CSS or SCSS Files
To import another CSS or SCSS file in SASS, use the @import
directive as follows:
@import url(reset.scss);
Creating a Base Style with Mixins
Mixins allow you to define styles that can be reused throughout your stylesheet. They can also accept arguments which makes them extremely flexible.
To define a mixin:
@mixin style_name {
properties;
}
To use a mixin:
element {
@include style_name;
}
You can also pass in parameters to your mixins, similar to a function:
@mixin style_name($param1, $param2) {
property1: $param1;
property2: $param2;
}
h1 {
@include style_name(red, 12em);
}
Inheritance in SASS
To inherit properties from one selector to another, use the @extend
directive:
.my_style {
properties;
}
.my_style_child {
@extend .my_style;
}
SASS Functions
SASS also supports the use of functions. Here are a few examples:
Power function:
@function pow($base, $exponent) {
$result: 1;
@for $_ from 1 to $exponent {
$result: $result * $base;
}
@return $result;
}
Color inversion function:
@function invert($color, $amount: 100%) {
$inverse: change-color($color, $hue: hue($color) + 180);
@return mix($inverse, $color, $amount);
}
These functions can be used in your stylesheet to manipulate properties and values.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version