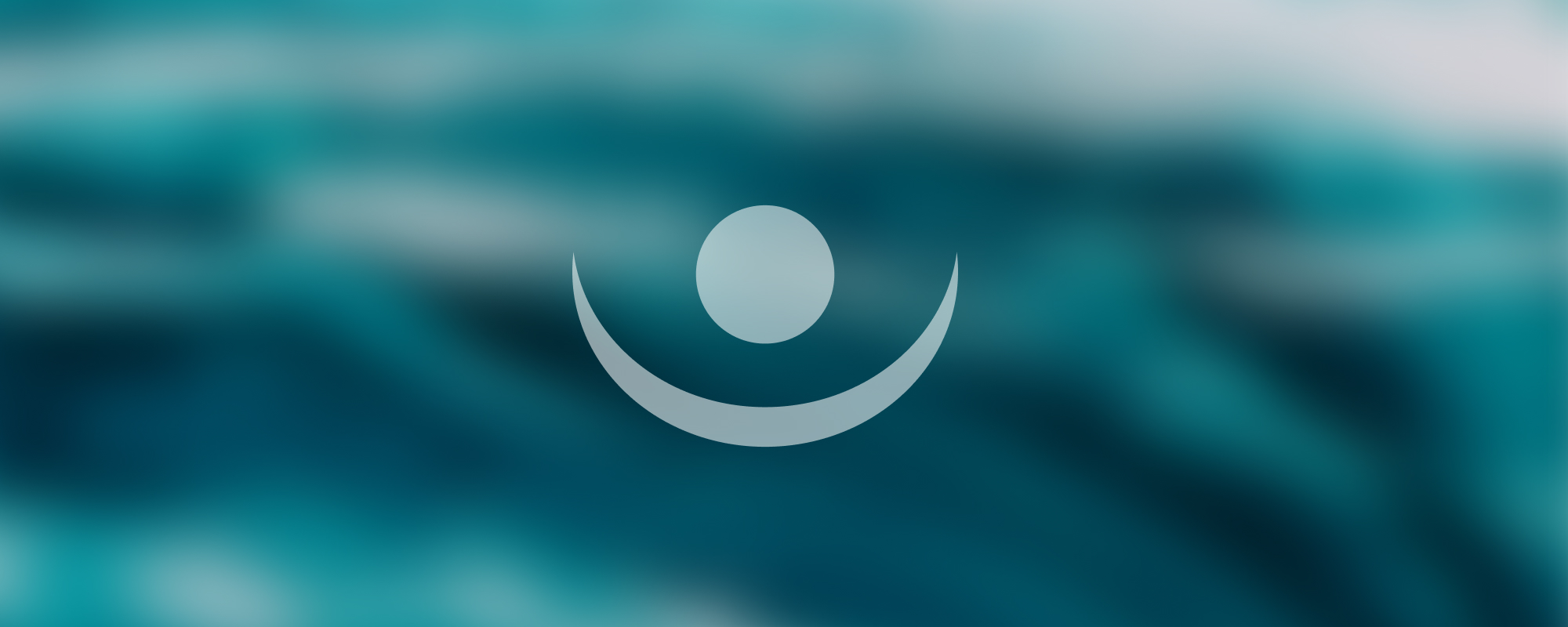
1. Introduction To Windows Forms
Introduction to Windows Forms in C#
What is Windows Forms?
Windows Forms (WinForms) is a UI framework within .NET used for building desktop applications. Primarily, it is written in C#. WinForms provides a rich set of controls that you can use to build highly interactive and responsive UIs.
Key Points:
- WinForms is part of the .NET framework.
- It is mainly used with the C# programming language.
- It allows for the creation of desktop applications.
Understanding Windows Forms
A form in Windows Forms is essentially a window or dialog box that can host controls. These controls can be buttons, text boxes, drop-down lists, and many other UI elements. Each form in Windows Forms acts as a container for these controls.
Displaying a Form
The method form.Show()
is used to display a form as an extra window that does not prevent the user from interacting with other windows.
Example:
Form myForm = new Form();
myForm.Show();
In contrast, form.ShowDialog()
opens the form as a modal dialog box. This means the user cannot switch to another window until they have closed the dialog box.
Example:
Form myForm = new Form();
myForm.ShowDialog();
Event Handling in Windows Forms
In Windows Forms, an event is a response to a specific action taken by the user or system. Examples of events include a button click, a form load, or a key press.
Defining an Event Handler
An event handler is a method that is called when an event occurs. The method MyForm_Click
in the example below is an event handler that is triggered when the form is clicked.
private void MyForm_Click(object sender, EventArgs e)
{
throw new NotImplementedException();
}
In this example, sender
represents the source of the event (like a form or a button), and EventArgs e
contains additional event-specific information.
Triggering Events
To trigger the MyForm_Click
event, you would typically wire it up to a form's Click
event in the form's constructor or the Load
event, like so:
public class MyForm : Form
{
public MyForm()
{
this.Click += MyForm_Click;
}
private void MyForm_Click(object sender, EventArgs e)
{
// Handle the event here
}
}
In this code, the MyForm_Click
method will be called whenever the form is clicked.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version