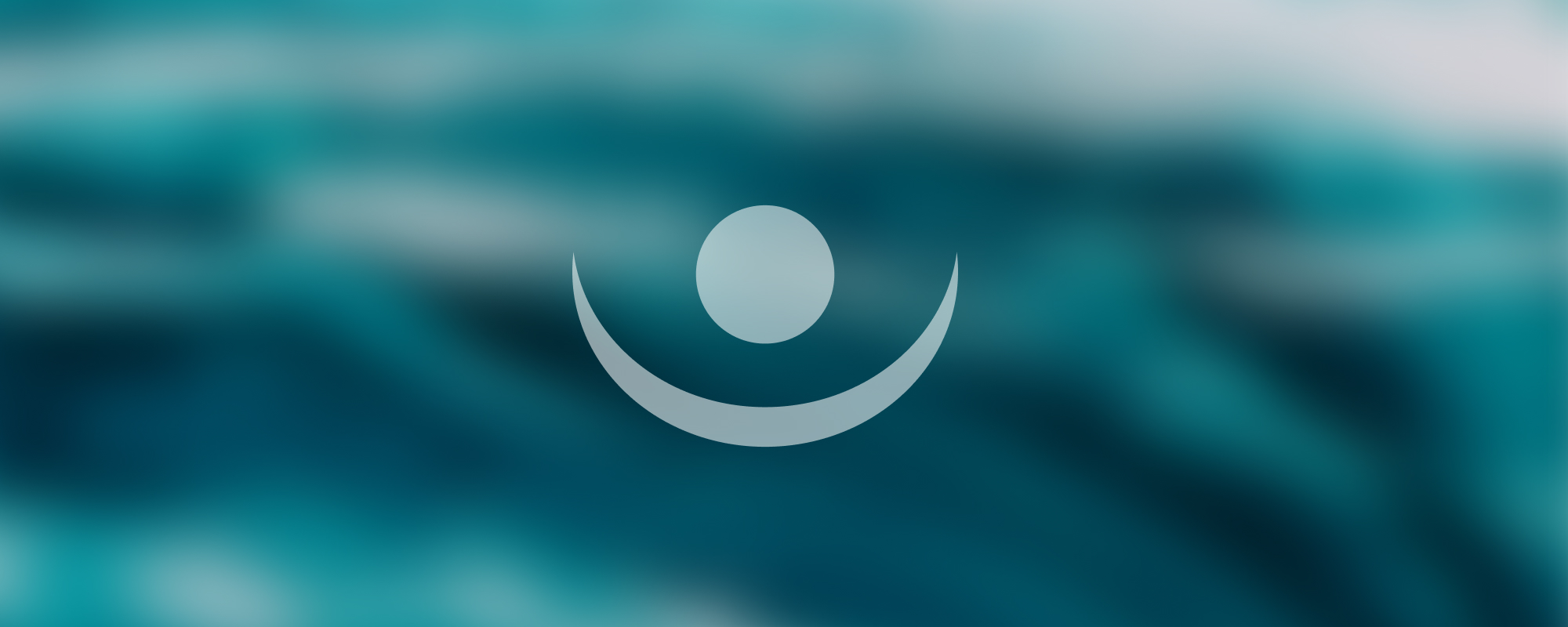
2. Controls
Web Windows Forms Controls
Web Windows Forms provides various controls that allow the user to interact with an application. These include TextBox, RichTextBox, MaskedTextBox, CheckBox, RadioBox, and GroupBox.
TextBox Control
The TextBox control allows the user to enter text in an application. It provides additional functionality that is not found in the standard Windows text box control, including multiline editing and password character masking.
Key Properties
- Multiline: Allows the TextBox to display or accept multiple lines of text.
- ScrollBars: Provides scroll bars if the text exceeds the visible area of the TextBox.
- AcceptsTab and AcceptsReturn: When set to true, these properties enable greater text manipulation in a multiline TextBox control.
Example:
TextBox textBox1 = new TextBox();
textBox1.Multiline = true;
textBox1.ScrollBars = ScrollBars.Vertical;
textBox1.AcceptsReturn = true;
textBox1.AcceptsTab = true;
RichTextBox Control
The RichTextBox control allows the user to enter and edit text, providing more advanced formatting features than the TextBox control. The text content can be assigned directly to the control, or loaded from a rich text format (RTF) or plain text file.
Key Operations
- Select: Highlight a portion of the text within the control.
- Copy: Copy the selected text to the clipboard.
- Paste: Insert clipboard content into the control.
- Cut: Remove the selected text and copy it to the clipboard.
- Undo: Revert the last operation.
- Redo: Apply again the last operation that was undone.
Example:
RichTextBox richTextBox1 = new RichTextBox();
richTextBox1.LoadFile("example.rtf");
richTextBox1.SelectAll();
richTextBox1.Copy();
MaskedTextBox Control
The MaskedTextBox control is an enhanced TextBox control that supports a declarative syntax for accepting or rejecting user input. It allows you to specify the required input characters, optional input characters, the type of input expected at a given position in the mask, mask literals, and special processing for input characters.
Example:
MaskedTextBox maskedTextBox1 = new MaskedTextBox();
maskedTextBox1.Mask = "(999) 000-0000"; // Phone number format
CheckBox Control
The CheckBox control allows the user to make a binary choice, i.e., a choice between two options, such as yes/no or on/off.
Example:
CheckBox checkBox1 = new CheckBox();
checkBox1.Text = "Check me";
checkBox1.Checked = true; // The CheckBox is checked by default
RadioBox Control
The RadioBox control allows the user to select one option from a group of choices. When one RadioBox is selected, all others are automatically unselected.
Example:
RadioButton radioButton1 = new RadioButton();
radioButton1.Text = "Option 1";
RadioButton radioButton2 = new RadioButton();
radioButton2.Text = "Option 2";
GroupBox Control
The GroupBox control is a container control that can hold a group of other controls like CheckBoxes or RadioBoxes. It provides an easy way to visually group related controls.
Example:
GroupBox groupBox1 = new GroupBox();
groupBox1.Controls.Add(radioButton1);
groupBox1.Controls.Add(radioButton2);
Remember, these controls play a critical role in creating your user interface and enabling user interaction. Understanding how to utilize them effectively can greatly enhance your ability to create dynamic and interactive applications.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version