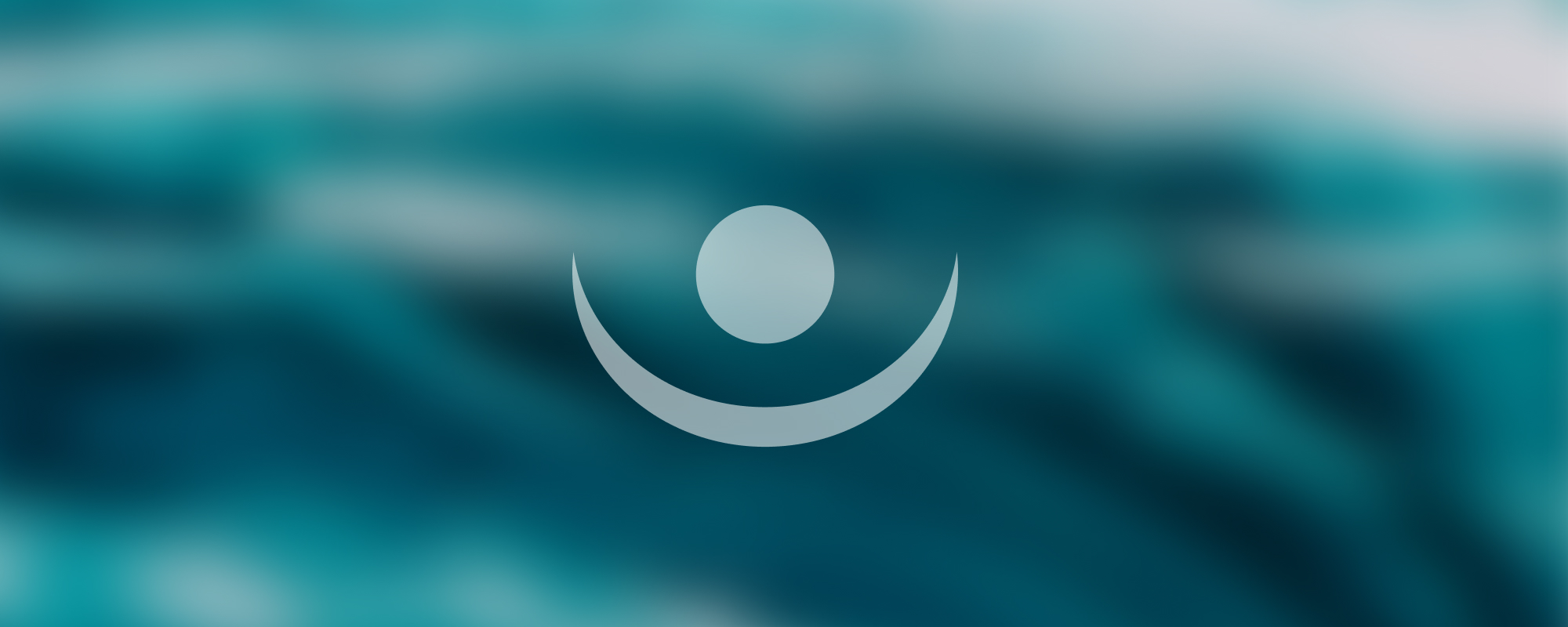
5. Practise
Web Windows Forms: Understanding Panels
Introduction to Panels in Web Windows Forms
A Panel is a container that can hold a group of controls. Panels are used to manage the layout of the form. They are instrumental in grouping UI (User Interface) elements and providing an organized and clean interface.
Key Points:
- A panel can contain other panels, controls and even other containers.
- The property
AutoScroll
of a Panel is set totrue
to automatically display scrollbars when the contents of the panel exceed its boundaries.
The Role of the Panel
The Panel plays a significant role in UI design by acting as a container. It can be used to group related controls together, which can then be collectively hidden, shown, enabled, or disabled.
Example of a Simple Panel
Here is an example of how to create a panel in a Web Windows Form:
// Create a new Panel
Panel panel1 = new Panel();
// Set the location and size of the Panel
panel1.Location = new Point(10, 10);
panel1.Size = new Size(200, 200);
// Add the Panel to the Form
this.Controls.Add(panel1);
In this example, a new Panel named panel1
is created. Its location and size are set before it is added to the form.
Nesting Panels
A Panel can contain other Panels. This is called nesting and it allows for more complex layouts. Nested panels can be individually controlled, meaning they can be shown, hidden, enabled, or disabled separately from the parent Panel.
Example of Nesting Panels
// Create a new Panel
Panel panel1 = new Panel();
// Set the location and size of the Panel
panel1.Location = new Point(10, 10);
panel1.Size = new Size(200, 200);
// Create a second Panel to nest inside panel1
Panel panel2 = new Panel();
// Set the location and size of the nested Panel
panel2.Location = new Point(20,20);
panel2.Size = new Size(100, 100);
// Add panel2 to panel1
panel1.Controls.Add(panel2);
// Add panel1 to the Form
this.Controls.Add(panel1);
In this example, a second Panel named panel2
is created and added to panel1
. The nested Panel can be controlled separately from its parent, providing greater flexibility in UI design.
Conclusion
Panels are an essential part of Web Windows Forms, acting as containers for other controls. They allow for an organized and clean UI design, with the ability to nest panels providing further flexibility and control. Understanding how to use Panels effectively is a key skill in creating user-friendly interfaces.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version