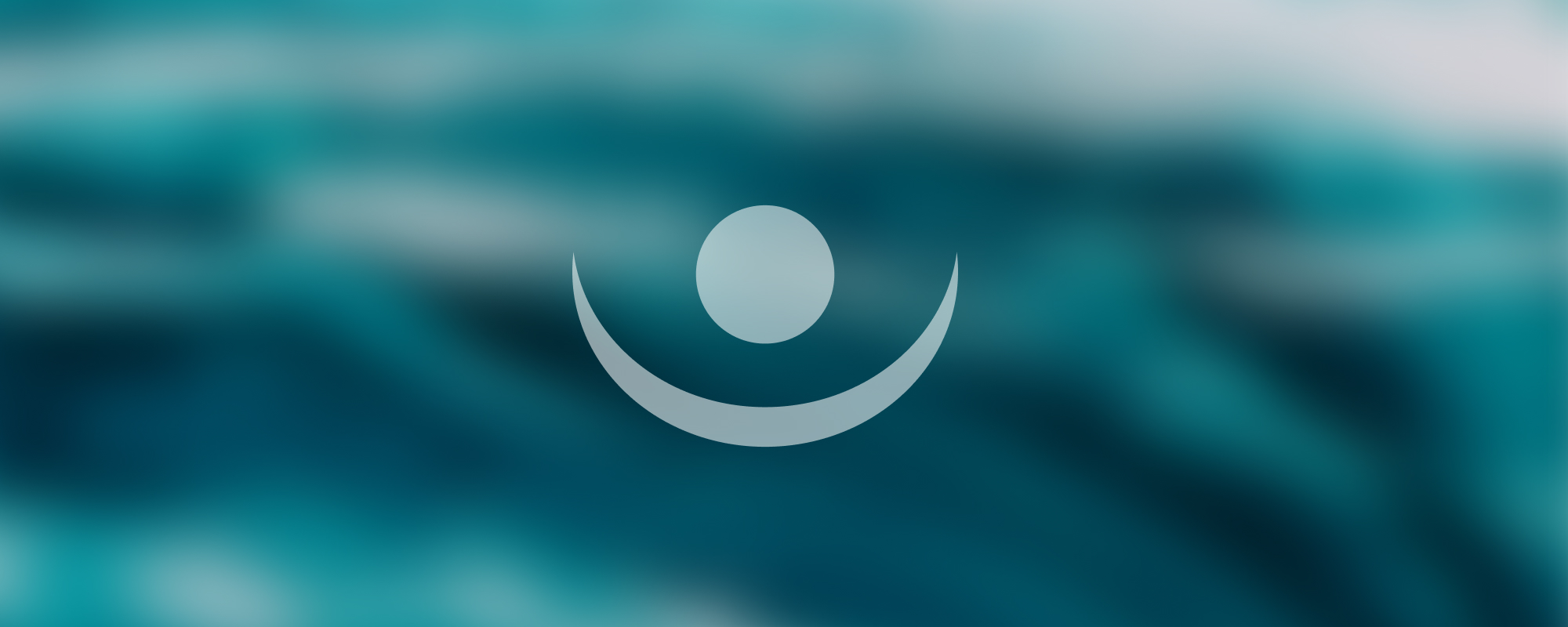
7. MVP
Web Windows Forms: Dialogs and Design Patterns
Introduction to Dialogs
Dialogs in Web Windows Forms are components that perform certain operations or actions. They are typically used to interact with the user and request input or provide options for a specific task. Although they perform an important function, they are not visible on the page.
OpenFileDialog
The OpenFileDialog is used to select a file. It opens a dialog box where you can browse and pick files from your system. This dialog is commonly used for opening or importing files.
Example:
OpenFileDialog openFileDialog = new OpenFileDialog();
if(openFileDialog.ShowDialog() == DialogResult.OK)
{
string fileName = openFileDialog.FileName;
// do something with the file
}
FontDialog
The FontDialog is used to select a font. It opens a dialog box where you can choose a font, font style, and size, among other things. This is particularly useful when you want to provide customization options for text-based elements in your application.
Example:
FontDialog fontDialog = new FontDialog();
if(fontDialog.ShowDialog() == DialogResult.OK)
{
Font font = fontDialog.Font;
// apply the font to a control
}
SaveFileDialog
The SaveFileDialog is used to save a file. It opens a dialog box to select a location to save a file and type a filename. This is often used when you need to export or save data to a file.
Example:
SaveFileDialog saveFileDialog = new SaveFileDialog();
if(saveFileDialog.ShowDialog() == DialogResult.OK)
{
string filePath = saveFileDialog.FileName;
// save data to the file
}
ColorDialog
The ColorDialog is used to select a color. It opens a dialog box where you can choose a color from the available options. This dialog is often used when you need to provide color options, for example, for a drawing tool or to customize the appearance of an application.
Example:
ColorDialog colorDialog = new ColorDialog();
if(colorDialog.ShowDialog() == DialogResult.OK)
{
Color color = colorDialog.Color;
// use the color for something
}
Design Patterns
A design pattern is a general repeatable solution to a commonly occurring problem in software design. It represents the best practices evolved over time by experienced software developers.
Model View Presenter (MVP)
MVP is a derivative of the known MVC (Model View Controller) design pattern but adapted for modern use. In this pattern, the Presenter contains the UI business logic for the View. All invocations from the View delegate directly to Presenter.
Model View Controller (MVC)
MVC is a design pattern used to decouple user-interface (view), data (model), and application logic (controller). This pattern helps to achieve separation of concerns.
Model View ViewModel (MVVM)
MVVM is a design pattern for building user interfaces. It's used to separate the application's user interface logic from its business logic. This pattern is commonly used in WPF, Silverlight, and other XAML-based applications.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version