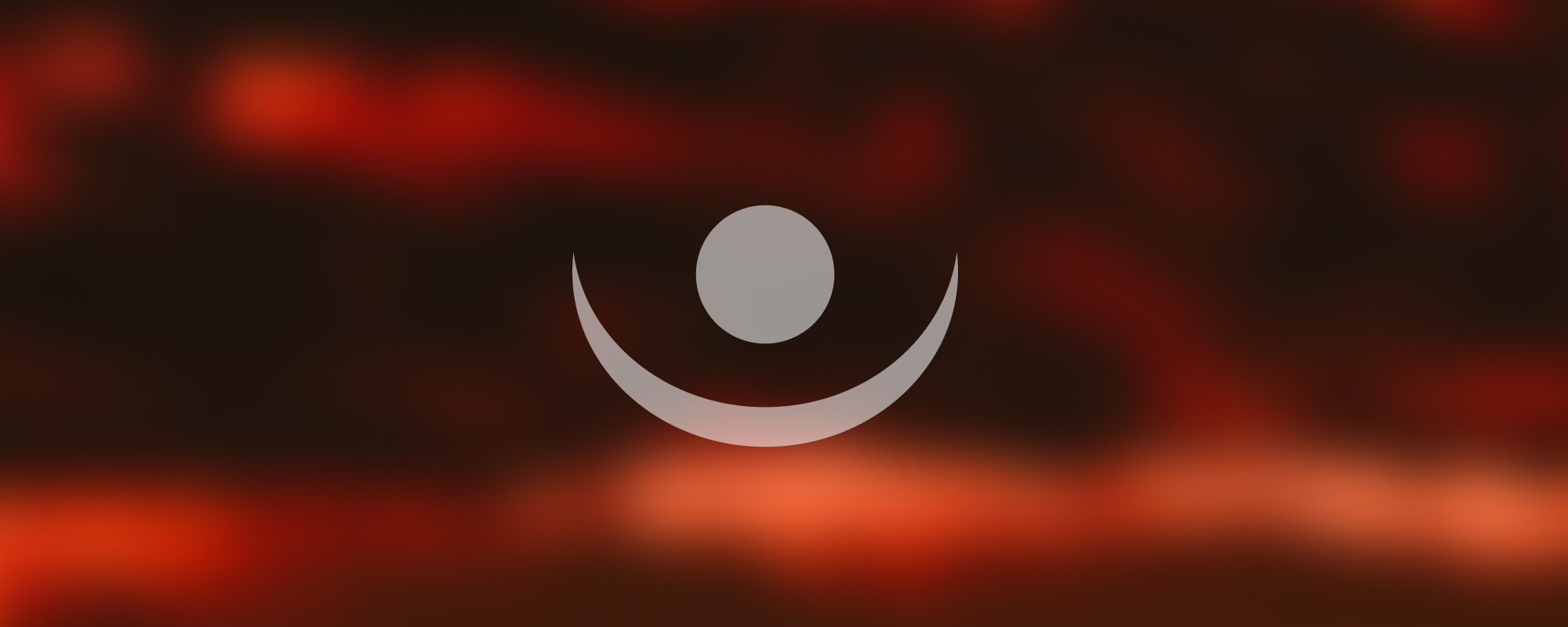
10. WPF Examples, Example With User Controls
WPF Examples: Example With User Controls
Introduction to Windows Presentation Foundation (WPF)
Windows Presentation Foundation (WPF) is a UI framework that creates desktop client applications. The WPF development platform supports a broad set of application development features, including an application model, resources, controls, graphics, layout, data binding, and documents.
What are User Controls in WPF?
User Controls in WPF are containers that encapsulate parts of a User Interface (UI) that are reusable. They are a simplified version of custom controls, and while they lack some of the power and flexibility of custom controls, they are easier to create.
Key Points:
- User controls are a way to create your own controls by grouping existing ones.
- They are typically used when a certain UI is likely to be reused throughout your application.
Creating a User Control
To create a User Control, you can right-click on the project in the Solution Explorer in Visual Studio, then go to Add > New Item > User Control (WPF).
After creating a User Control, you can add existing WPF controls to it, such as buttons, text boxes, labels, and others. These can be arranged and handled in a way that makes sense for the encapsulated functionality you're aiming for.
Example:
<UserControl x:Class="WpfApp1.MyUserControl"
xmlns="<http://schemas.microsoft.com/winfx/2006/xaml/presentation>"
xmlns:x="<http://schemas.microsoft.com/winfx/2006/xaml>"
xmlns:mc="<http://schemas.openxmlformats.org/markup-compatibility/2006>"
xmlns:d="<http://schemas.microsoft.com/expression/blend/2008>"
mc:Ignorable="d"
d:DesignHeight="450" d:DesignWidth="800">
<Grid>
<Button Content="My Button" HorizontalAlignment="Left" Margin="10" VerticalAlignment="Top" Width="75"/>
<TextBox HorizontalAlignment="Left" Height="23" Margin="10,45,0,0" TextWrapping="Wrap" Text="TextBox" VerticalAlignment="Top" Width="120"/>
</Grid>
</UserControl>
Using a User Control in a WPF Window
Once a User Control has been created, it can be used within a WPF window. You need to add a reference to the namespace of the User Control in the window's XAML, and then you can use the User Control as a normal WPF control.
Example:
<Window x:Class="WpfApp1.MainWindow"
xmlns="<http://schemas.microsoft.com/winfx/2006/xaml/presentation>"
xmlns:x="<http://schemas.microsoft.com/winfx/2006/xaml>"
xmlns:local="clr-namespace:WpfApp1"
Title="MainWindow" Height="450" Width="800">
<Grid>
<local:MyUserControl />
</Grid>
</Window>
In the above example, the xmlns:local
attribute defines a namespace reference, with local
being an arbitrary name, and clr-namespace:WpfApp1
being the actual namespace of the User Control. The local:MyUserControl
element then places the User Control in the window.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version