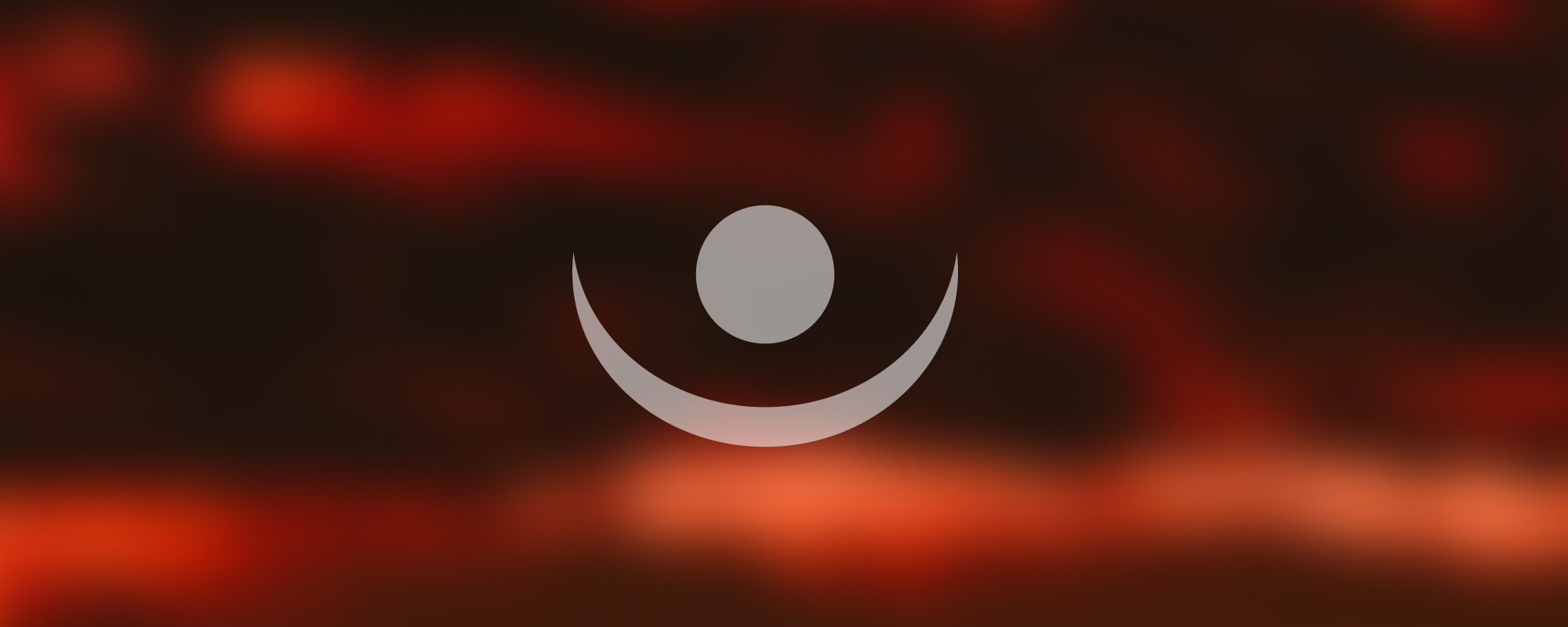
2. Containers
Containers in WPF
Introduction to Containers
In Windows Presentation Foundation (WPF), Containers are objects that can contain other UI elements. They are used to arrange and manage the layout of these elements in the user interface.
Different Types of Containers
- Grid
- UniformGrid
- Canvas
- StackPanel
- WrapPanel
- DockPanel
Grid
A Grid is a container that allows you to create a flexible layout of rows and columns to form a matrix structure. It is particularly useful when you want to divide your interface into distinct areas.
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="*" />
<RowDefinition Height="*" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*" />
<ColumnDefinition Width="*" />
</Grid.ColumnDefinitions>
<!-- Place your controls here -->
</Grid>
UniformGrid
The UniformGrid container is used to create a grid where all cells have the same size. It automatically scales all elements inside it to the same size.
<UniformGrid Rows="2" Columns="2">
<Button Content="Button 1" />
<Button Content="Button 2" />
<Button Content="Button 3" />
<Button Content="Button 4" />
</UniformGrid>
Canvas
A Canvas is a container that allows you to position and layer elements in a free form manner.
<Canvas>
<Ellipse Canvas.Left="50" Canvas.Top="50" Fill="Blue" Height="100" Width="100" />
<Ellipse Canvas.Left="70" Canvas.Top="70" Fill="Green" Height="100" Width="100" />
</Canvas>
StackPanel
A StackPanel is a container that arranges its child elements into a single line that can be oriented horizontally or vertically.
<StackPanel Orientation="Vertical">
<Button Content="Button 1" />
<Button Content="Button 2"/>
<Button Content="Button 3" />
</StackPanel>
WrapPanel
A WrapPanel is a container that positions its children in sequential position from left to right (or top to bottom), breaking the content to the next line at the edge of its containing box.
<WrapPanel>
<Button Content="Button 1" />
<Button Content="Button 2"/>
<Button Content="Button 3" />
<!-- More buttons -->
</WrapPanel>
DockPanel
A DockPanel is a container that allows you to arrange its child elements to the top, bottom, left, and right edges of the panel.
<DockPanel>
<Button Content="Top" DockPanel.Dock="Top"/>
<Button Content="Bottom" DockPanel.Dock="Bottom"/>
<Button Content="Left" DockPanel.Dock="Left"/>
<Button Content="Right" DockPanel.Dock="Right"/>
</DockPanel>
Important Points to Note
- If a container's orientation is vertical, only horizontal alignment will work for its children, and vice versa.
- Negative values should not be used for Margin and Padding. A maximum value of 50 is optimal.
Topics Covered
- Label
- RenderTransform
- RotateTransform, ScaleTransform, SkewTransform, TransformGroup
- CheckBox
- Ellipse, Rectangle, Canvas, StackPanel
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version