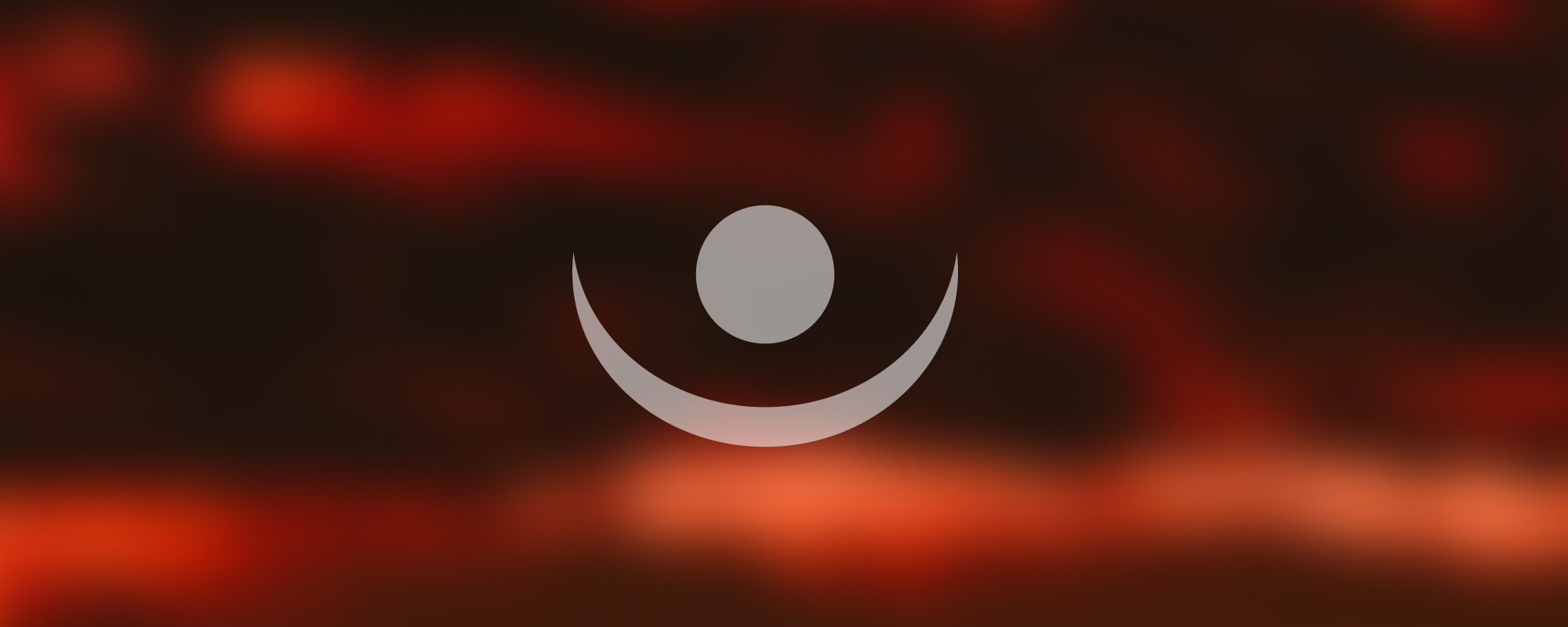
3. Controls
Controls in WPF (Windows Presentation Foundation)
Introduction
Windows Presentation Foundation (WPF) is a UI framework that creates desktop client applications. The WPF development platform supports a broad set of application development features, including an application model, resources, controls, graphics, layout, data binding, and documents. This note focuses on the Controls aspect of WPF.
Buttons in WPF
Button Control
A Button in WPF is an interactive UI control that users can click to perform an action. The Button.BitmapEffect
property allows you to set visual effects on the button, such as drop shadow, blur, or glow. For instance, you can use the DropShadowBitmapEffect
to add a shadow effect to the button.
Example:
Button button = new Button();
DropShadowBitmapEffect myDropShadowEffect = new DropShadowBitmapEffect();
button.BitmapEffect = myDropShadowEffect;
RadioButton Control
RadioButtons can be grouped together to ensure that only one button in a group can be selected at a time. This can be achieved using the GroupName
property.
Example:
RadioButton radioButton1 = new RadioButton();
RadioButton radioButton2 = new RadioButton();
radioButton1.GroupName = "group1";
radioButton2.GroupName = "group1";
ToggleButton Control
A ToggleButton
is a button that can be toggled between two states. It is useful when you want a button that can be checked or unchecked like a checkbox but looks like a button.
Example:
ToggleButton toggleButton = new ToggleButton();
toggleButton.Content = "Toggle Button";
RepeatButton Control
The RepeatButton
control is a button that raises click events repeatedly from the time it is pressed until it is released. This is useful for scenarios where a continuous action should be triggered while a button is being pressed.
Example:
RepeatButton repeatButton = new RepeatButton();
repeatButton.Content = "Repeat Button";
repeatButton.Click += (s, e) => {
// Action to perform on click
};
Tooltip Control
A ToolTip
can be attached to any FrameworkElement
in WPF. It provides supplementary information about the function of the control. The tooltip text is set by the ToolTip
property.
Example:
Button button = new Button();
button.ToolTip = "This is a tooltip";
GroupBox Control
A GroupBox
is a control that provides a group box that can display a title and host content within a border. The title is set by the Header
property.
Example:
GroupBox groupBox = new GroupBox();
groupBox.Header = "This is a group box";
ScrollViewer Control
A ScrollViewer
is a control that provides a scrollable area that can contain other visible elements. It provides horizontal and vertical scroll bars.
Example:
ScrollViewer scrollViewer = new ScrollViewer();
Expander Control
An Expander
control is like a collapsible group box. It includes a header and a collapsible content area. This is similar to the ComboBox in Windows Forms.
Example:
Expander expander = new Expander();
expander.Header = "Expander Header";
expander.Content = "Expander Content";
These are some of the basic controls in WPF which are used to build the user interface of a WPF application. There are many more controls available in WPF, and each provides a wide variety of properties that can be set to customize its behavior and appearance.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version