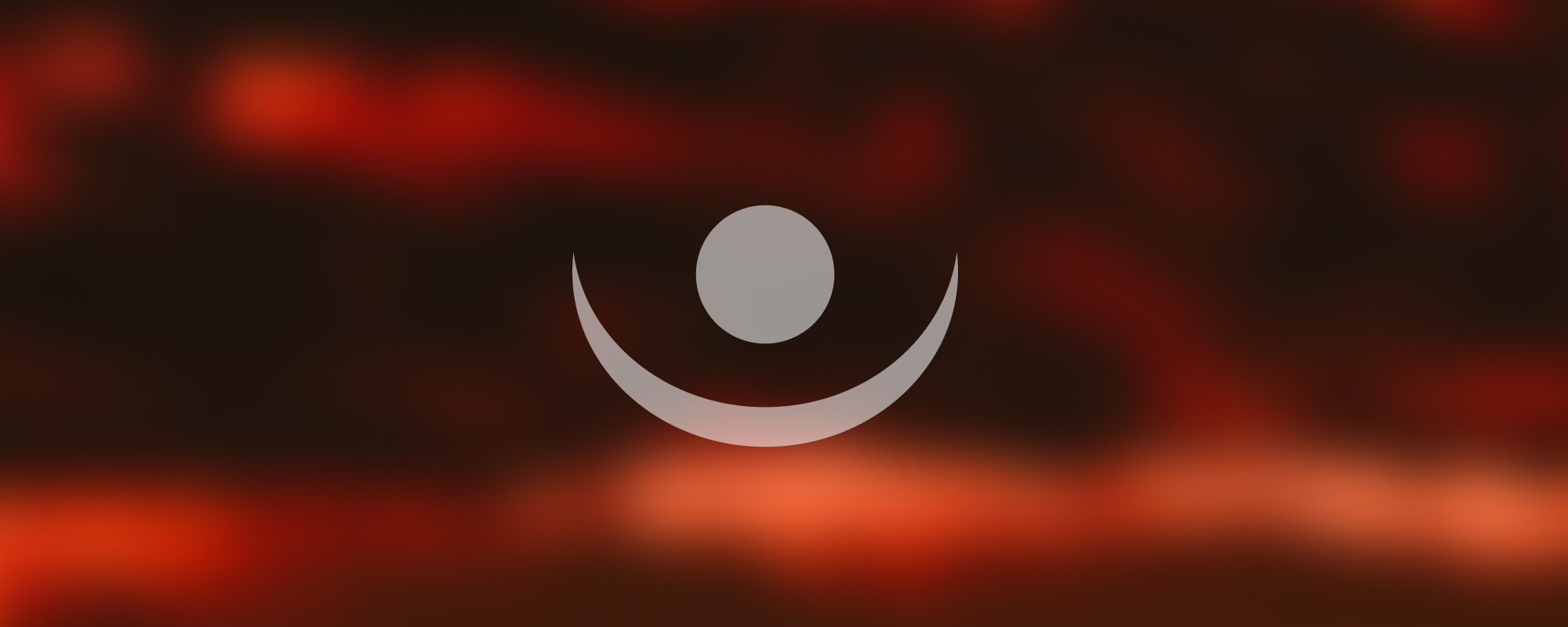
4. Controls 2
Controls 2 in WPF
Windows Presentation Foundation (WPF) provides a set of UI controls that you can use to create rich user interfaces. This lesson covers the following controls: ProgressBar, Slider, TabControl, ListBox, and ComboBox.
ProgressBar Control
The ProgressBar control in WPF represents a progress bar that visually indicates the progress of a long-running operation.
Key Properties:
Maximum
: The maximum value of the progress bar.
Minimum
: The minimum value of the progress bar.
Value
: The current progress value.
ProgressBar progressBar = new ProgressBar();
progressBar.Maximum = 100;
progressBar.Minimum = 0;
progressBar.Value = 50;
Slider Control
The Slider control in WPF represents a control that lets the user select from a range of values by moving a thumb control along a track.
Example:
Slider slider = new Slider();
slider.Minimum = 0;
slider.Maximum = 100;
slider.Value = 50;
TabControl
The TabControl in WPF allows you to split your interface up into different areas, each accessible by clicking on the tab header, usually positioned at the top of the control. It's a useful control for saving space on your form. With TabControl, there's no need to create separate user controls.
Example:
TabControl tabControl = new TabControl();
TabItem tabItem1 = new TabItem();
tabItem1.Header = "Tab 1";
TabItem tabItem2 = new TabItem();
tabItem2.Header = "Tab 2";
tabControl.Items.Add(tabItem1);
tabControl.Items.Add(tabItem2);
ListBox Control
The ListBox control in WPF displays a list of items to the user. The ListBox control can be customized by changing the ItemsPanel
property.
WrapPanel
A WrapPanel arranges its child elements from left to right, breaking content to the next line at the edge of the enclosing box.
<ListBox>
<ListBox.ItemsPanel>
<ItemsPanelTemplate>
<WrapPanel />
</ItemsPanelTemplate>
</ListBox.ItemsPanel>
</ListBox>
VirtualizingStackPanel
A VirtualizingStackPanel arranges its items in a single line which can be oriented vertically or horizontally. It creates visual elements for data items when they're visible and discards them when they're not.
<ListBox>
<ListBox.ItemsPanel>
<ItemsPanelTemplate>
<VirtualizingStackPanel />
</ItemsPanelTemplate>
</ListBox.ItemsPanel>
</ListBox>
ComboBox Control
The ComboBox control is similar to ListBox but the list is displayed in a drop down. Like ListBox, ComboBox can be populated with custom items and uses the same panel templates.
WrapPanel
<ComboBox>
<ComboBox.ItemsPanel>
<ItemsPanelTemplate>
<WrapPanel />
</ItemsPanelTemplate>
</ComboBox.ItemsPanel>
</ComboBox>
VirtualizingStackPanel
<ComboBox>
<ComboBox.ItemsPanel>
<ItemsPanelTemplate>
<VirtualizingStackPanel />
</ItemsPanelTemplate>
</ComboBox.ItemsPanel>
</ComboBox>
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version