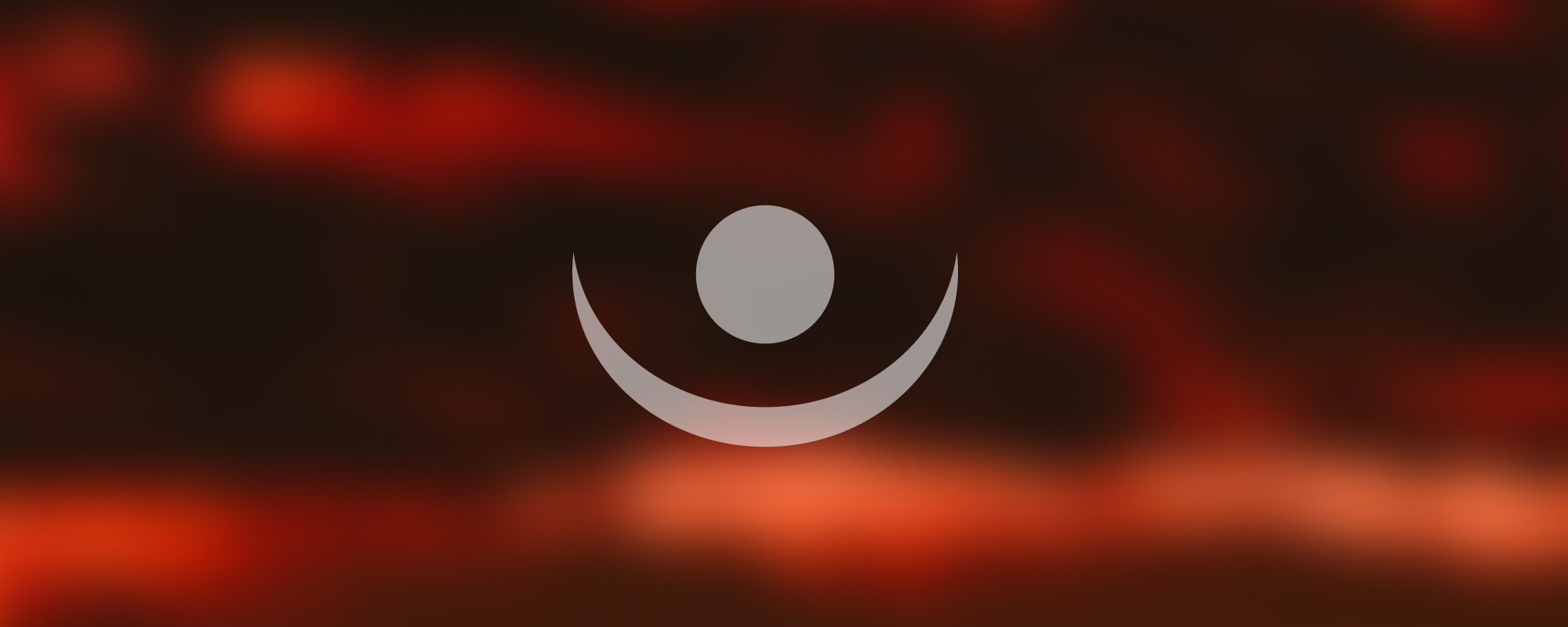
5. Views
Views in WPF
Windows Presentation Foundation (WPF) provides a rich environment for creating desktop applications. Among the various features it offers, an important one is the concept of views, which allow for the display and manipulation of data in various ways. This note will primarily focus on ListView
, GridView
, DataTemplate
, Menu
, ContextMenu
, TreeView
, and Binding
.
ListView in WPF
A ListView
in WPF is a control that displays a list of data items. It uses the ItemSource
property to bind to a collection of data items. The ListView
control has a built-in functionality to automatically generate its columns according to the bound ItemSource
.
Example:
ListView listView = new ListView();
listView.ItemsSource = data; // data can be any enumerable collection
GridView in WPF
GridView
is often used as a view mode for ListView
control. It displays the list of data items in columns for detailed viewing. GridView
uses GridViewColumn
to define the columns that should be displayed.
Example:
GridView gridView = new GridView();
listView.View = gridView;
gridView.Columns.Add(new GridViewColumn { Header = "Name", DisplayMemberBinding = new Binding("Name") });
gridView.Columns.Add(new GridViewColumn { Header = "Age", DisplayMemberBinding = new Binding("Age") });
Binding in WPF
Binding in WPF is a process that establishes a connection between the UI and the business logic. It allows the flow of data between the two. The DisplayMemberBinding
property is used to bind a property of a data item to a particular column.
Example:
GridViewColumn column = new GridViewColumn();
column.DisplayMemberBinding = new Binding("PropertyName");
DataTemplate in WPF
DataTemplate
in WPF is used to define the visualization of data. It allows you to create a custom presentation of a data object on a UI. The ListBoxItemTemplate
uses DataTemplate
to define the appearance of data items in the list.
Example:
<DataTemplate x:Key="myDataTemplate">
<StackPanel Orientation="Horizontal">
<TextBlock Text="{Binding Name}" />
<TextBlock Text="{Binding Age}" />
</StackPanel>
</DataTemplate>
Menu in WPF
The Menu
control in WPF provides a menu in your application. It opens when you perform a left click.
Example:
<Menu>
<MenuItem Header="File">
<MenuItem Header="New"></MenuItem>
<MenuItem Header="Open"></MenuItem>
<MenuItem Header="Save"></MenuItem>
</MenuItem>
</Menu>
ContextMenu in WPF
ContextMenu
is a pop-up menu that appears on a right click. It provides a list of menu items that a user can select.
Example:
<Button Content="Right Click Me">
<Button.ContextMenu>
<ContextMenu>
<MenuItem Header="First Item"/>
<MenuItem Header="Second Item"/>
</ContextMenu>
</Button.ContextMenu>
</Button>
TreeView in WPF
A TreeView
control provides a hierarchical view of data and the user can expand or collapse the nodes to view the data.
Example:
<TreeView>
<TreeViewItem Header="Parent Node">
<TreeViewItem Header="Child Node1"/>
<TreeViewItem Header="Child Node2"/>
</TreeViewItem>
</TreeView>
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version