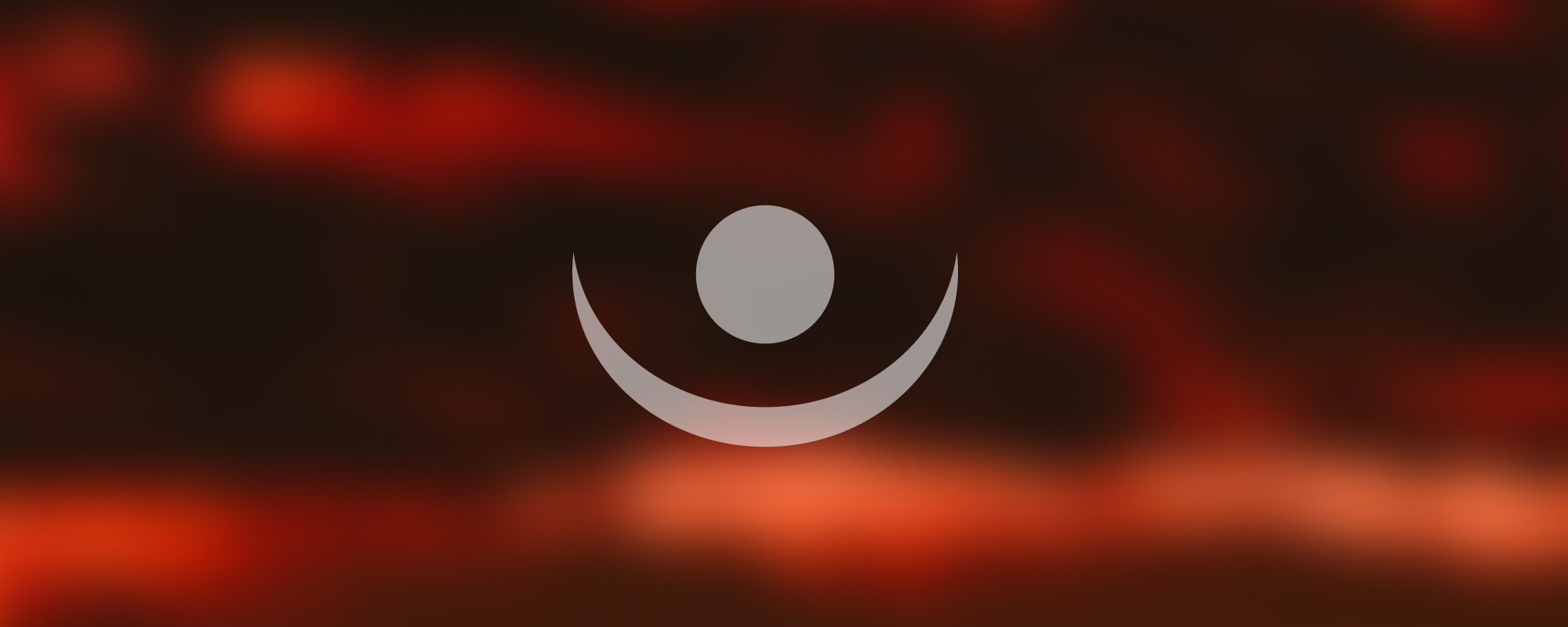
6. Binding
Binding in Windows Presentation Foundation (WPF)
Introduction to Binding
Binding in WPF is a mechanism that allows the flow of data between objects, typically between the user interface (UI) and business logic (also known as the model). This mechanism makes it easy to tie together the model and UI without having to write a lot of glue code.
Windows.Resources
Windows.Resources allows us to store a desired standard (e.g., size, color). This helps to avoid code redundancy. If we change something here, it also changes in all bound places.
StaticResource and DynamicResource
- StaticResource: This cannot be changed during runtime.
- DynamicResource: This can be modified during runtime.
Resource Dictionary
The Resource Dictionary is used globally. It must be added in App.xaml for it to be used everywhere. For writing orderly, it's better to extract resources into a dictionary.
Multiple dictionaries can be used by merging them in App.xaml.
Binding Elements
To bind an element, we use the following syntax:
<TextBox FontSize="{Binding ElementName=slider, Path=value}"></TextBox>
This binds the font size of the textbox to the value of the slider.
Binding Properties
- ElementName: The element we are binding to.
- Path: The property of the bound element.
- Modes
- One Way: When the source changes, the target changes. The source doesn't change when the target changes.
- One Way To Source: The reverse of One Way.
- Two Way: Both change together.
- UpdateSourceTrigger: Determines when the source will change.
Implementing Binding
When the bound property changes, it also changes in the view. To change it, we make the property fullprop and inherit the class from INotifyPropertyChanged (using ComponentModel). After implementing, we write the PropertyChanged event (also write an additional method(OnPropertyChanged)), and we write it in the setter of the fullprops. And whenever that fullprop changes (when set is executed), that method is called (we notify) and the text in the view changes.
ObservableCollection (Collection(List etc)) already has this method (i.e., it notifies the view).
DependencyProperty
Typing propdp tab tab
performs all these actions.
Example:
public class MyButton : Button
{
public static readonly DependencyProperty MyPropertyProperty =
DependencyProperty.Register("MyProperty", typeof(int), typeof(MyButton), new UIPropertyMetadata(0));
public int MyProperty
{
get { return (int)GetValue(MyPropertyProperty); }
set { SetValue(MyPropertyProperty, value); }
}
}
In this example, a DependencyProperty named MyProperty
is defined. It can be used to bind data between XAML and the code-behind in WPF.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version