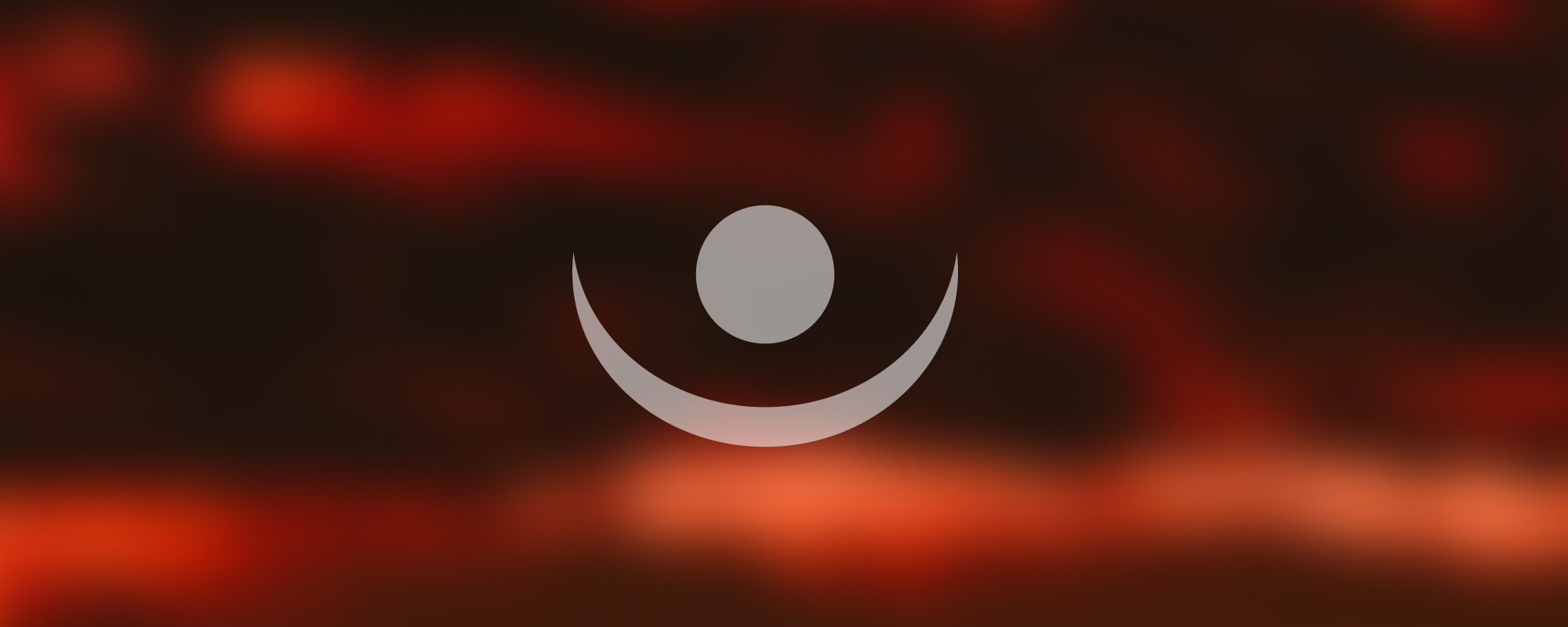
8. MVVM
The Model-View-ViewModel (MVVM) Pattern in WPF
Introduction to Design Patterns
Design Patterns are general solutions designed to tackle common problems in software design. They help simplify and streamline complex projects by providing tested, efficient methods of solving certain problems. However, for simpler projects, implementing design patterns (like MVVM) might not be necessary.
The MVVM Pattern
The Model-View-ViewModel (MVVM) pattern is a design pattern that separates the User Interface (View) from the business logic (ViewModel). This pattern is commonly used in Windows Presentation Foundation (WPF) applications. It promotes loose coupling and increases maintainability and testability.
In MVVM, each View should have a corresponding ViewModel.
Components of MVVM
- Model: Represents the actual data and/or information we are dealing with. The model classes are typically used in conjunction with services or repositories to encapsulate data access and manipulation logic.
- View: The user interface that displays information to the user.
- ViewModel: Acts as a link between the Model and the View. It handles the logic and operations of the View.
Note on Entity and Interaction
- Entity: In the context of databases, an Entity represents a table in the database. Each instance of an entity represents a row in the table.
- Interaction/Command: This is a way to send events from the View to the ViewModel. The command parameter allows us to send any parameter from the View to the ViewModel. In the ViewModel, RelayCommand works for the event.
Code Examples
Below is an example of how a simple MVVM pattern might look in a WPF application:
// Model
public class Product
{
public string Name { get; set; }
public decimal Price { get; set; }
}
// ViewModel
public class ProductViewModel
{
private Product _product;
public ProductViewModel()
{
_product = new Product { Name = "Apple", Price = 1.23M };
}
public string Name
{
get { return _product.Name; }
set { _product.Name = value; }
}
public string Price
{
get { return _product.Price.ToString("C"); }
set
{
decimal price;
if (decimal.TryParse(value, out price))
{
_product.Price = price;
}
}
}
}
// View
<TextBlock Text="{Binding Name}" />
<TextBlock Text="{Binding Price}" />
In this example, the Product
class is the Model, the ProductViewModel
is the ViewModel, and the XAML markup is the View.
The ViewModel includes properties that are data-bound to the View. The ViewModel does not have any knowledge of the View, it simply provides data which the View consumes. This is a key aspect of the MVVM design pattern.
This pattern can be a bit more complex when using RelayCommand for interactions or commands, but the fundamental idea remains the same. The aim is to separate concerns between the View and the business logic.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version