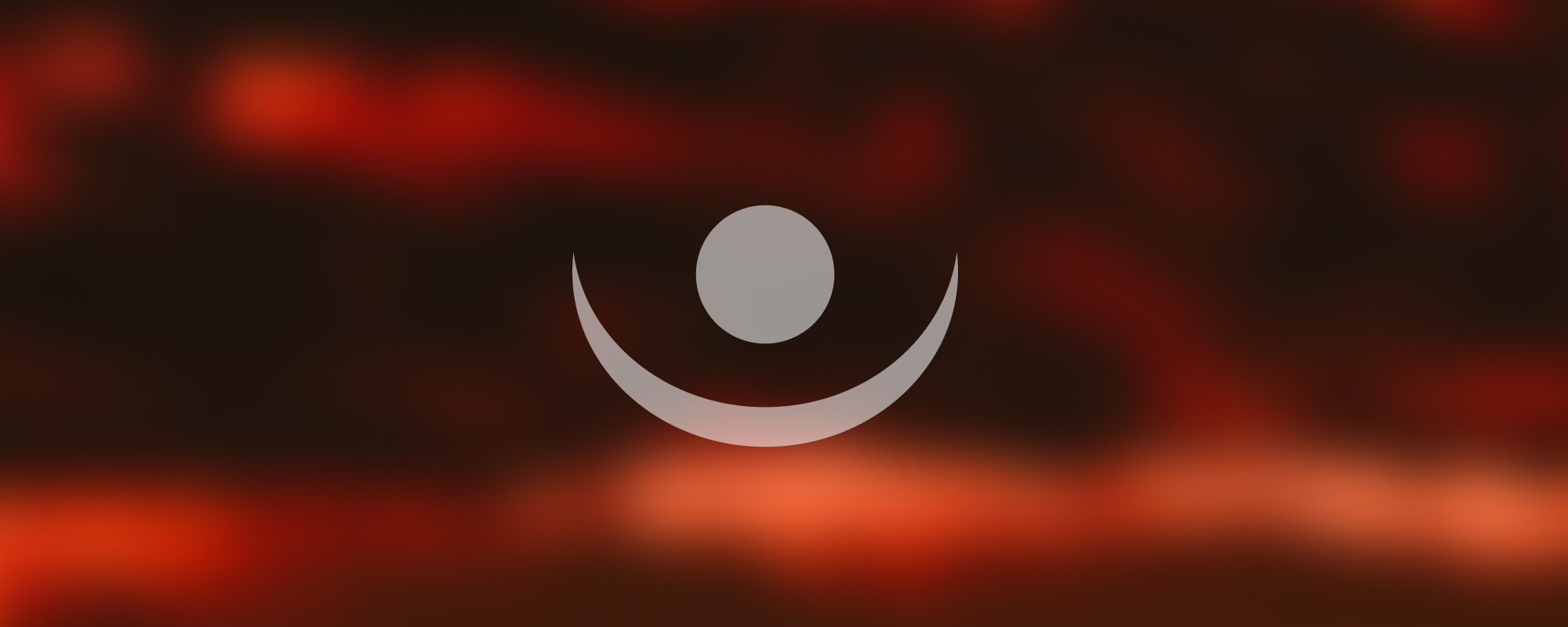
9. Styles, Animations
Styles and Animations in WPF (Windows Presentation Foundation)
Introduction to Styles
In Windows Presentation Foundation (WPF), styles are an efficient way to create a consistent user interface across your application. They help to reduce the redundancy of code by allowing you to define a set of property values to apply to multiple elements.
Key Points:
- Styles in WPF avoid code repetition.
- Styles can be written in a
Windows.Resources
or dictionaries.
- Styles written in dictionaries are visible across all windows (it's necessary to add the dictionary to
app.xaml
).
- Internal styles are written inside specific elements and are only applicable to that element.
Style.Triggers
Triggers in WPF are actions that are taken when specific conditions are met. They can be used to change the appearance or behavior of a control when an event occurs or a condition is met.
Triggers (Event Triggers)
Event triggers allow you to perform an action when a specified event is fired. This can be used to change the appearance of a control or start an animation.
Example:
<Button Width="100" Height="30" Content="Button">
<Button.Triggers>
<EventTrigger RoutedEvent="Button.Click">
<BeginStoryboard>
<Storyboard>
<DoubleAnimation Storyboard.TargetProperty="FontSize" To="30" Duration="0:0:0.2"/>
</Storyboard>
</BeginStoryboard>
</EventTrigger>
</Button.Triggers>
</Button>
DataTriggers
Data triggers allow you to perform an action based on the value of a bound property. This can be used to change the appearance of a control based on the value of a data-bound property.
Example:
<Button Width="100" Height="30" Content="{Binding ButtonContent}">
<Button.Style>
<Style TargetType="Button">
<Style.Triggers>
<DataTrigger Binding="{Binding ButtonContent}" Value="Click Me">
<Setter Property="Background" Value="Blue"/>
</DataTrigger>
</Style.Triggers>
</Style>
</Button.Style>
</Button>
ControlTemplate
A ControlTemplate
in WPF provides a way to redefine the entire visual tree of a control, essentially resetting and rewriting its appearance.
ContentPresenter
In WPF, a ContentPresenter
is an element that is used to display the content of another element. It's often used within a ControlTemplate
to specify where the content should be placed.
Example:
<ControlTemplate TargetType="Button">
<Border Background="{TemplateBinding Background}">
<ContentPresenter HorizontalAlignment="Center" VerticalAlignment="Center"/>
</Border>
</ControlTemplate>
Notes about SVG
SVG (Scalable Vector Graphics) is an XML-based vector image format for two-dimensional graphics with support for interactivity and animation. It's preferred for high-quality visuals as it doesn't pixelate on zooming. You can create SVG images using tools like Figma.com or Visual Studio Blend.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version